


Javascript Data Types : Is there any difference between Browser and NodeJs?
JavaScript core data types are consistent in browsers and Node.js, but they are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
When diving into JavaScript, understanding data types is fundamental, but it's equally important to grap how these types behave across different environments like browsers and Node.js. Let's explore this fascinating topic, sharing insights and experiences along the way.
In JavaScript, the core data types are consistent across environments: Number
, String
, Boolean
, Undefined
, Null
, Object
, and Symbol
(introduced in ES6). However, the way these types are handled or the additional types available can different slightly between browsers and Node.js, which can lead to some interesting nuances and potential pitfalls.
Let's start with a simple yet revealing example. In both environments, you can declare a variable and assign it a value:
let myNumber = 42; console.log(typeof myNumber); // Output: "number"
This code snippet works independently in both a browser and Node.js, showing that the basic data types are indeed the same. But let's dig deeper.
One of the key differences lies in the global
object. In a browser, the global object is window
, whereas in Node.js, it's global
. This difference can affect how you access global variables or functions:
// In a browser console.log(window); // Outputs the window object // In Node.js console.log(global); // Outputs the global object
This distinction is cruel when writing cross-platform JavaScript. If you're developing a library or application that needs to run in both environments, you'll need to handle these differences gracefully. A common approach is to use a conditional check:
const globalThis = (() => { if (typeof window !== 'undefined') { return window; } else if (typeof global !== 'undefined') { return global; } else { throw new Error('No global object found'); } })(); console.log(globalThis); // Works in both browser and Node.js
This snippet demonstrates a practical solution to the global object issue, but it also highlights a broader point: understanding the environment-specific nuances can help you write more robust code.
Another area where differences emerge is with Buffer
objects, which are unique to Node.js. Buffers are used to handle binary data, something that's not directly available in browsers. Here's a quick example:
// This will only work in Node.js const buffer = Buffer.from('Hello, World!'); console.log(buffer); // Outputs: <Buffer 48 65 6c 6c 6f 2c 20 57 6f 72 6c 64 21>
If you try to run this in a browser, you'll get an error because Buffer
is not defined. This is a clear example of how Node.js extends JavaScript with additional types that are not part of the standard ECMAScript specification.
When it comes to performance, there are also subtle differences. For instance, Node.js, being a server-side environment, often has different performance characteristics compared to browsers. Memory management, garbage collection, and even the JavaScript engine itself (V8 in both, but optimized differently) can lead to variations in how data types are handled.
From my experience, one of the trickiest aspects is dealing with Date
objects. While the Date
object itself is consistent across environments, the way time zones are handled can different. In a browser, the time zone is typically set by the user's system settings, whereas in Node.js, you might need to explicitly set the time zone:
// In Node.js process.env.TZ = 'America/New_York'; const date = new Date(); console.log(date); // Outputs date in New York time zone
This can lead to unexpected behavior if you're not careful, especially when dealing with applications that need to handle dates across different time zones.
In terms of best practices, always consider the environment in which your code will run. If you're writing code that needs to be cross-platform, use environment detection and conditional logic to handle differences. Also, be aware of the additional types and features available in Node.js, like Buffer
, and plan accordingly.
To wrap up, while the core JavaScript data types remain the same across browsers and Node.js, the way they're handled and the additional types available can lead to some interesting challenges and opportunities. By understanding these differences, you can write more versatile and robust JavaScript code that performs well in any environment.
So, keep exploring, keep learning, and don't be afraid to dive into the nuances of JavaScript across different platforms. It's these subtleties that make programming such a rewarding journey.
The above is the detailed content of Javascript Data Types : Is there any difference between Browser and NodeJs?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










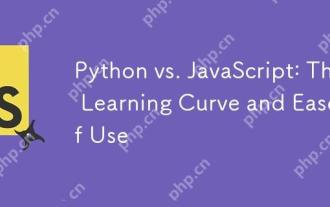
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
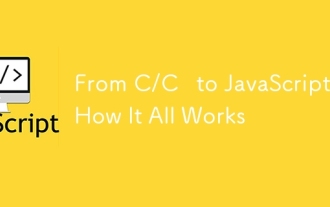
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
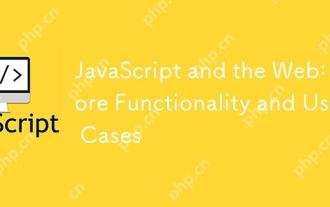
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
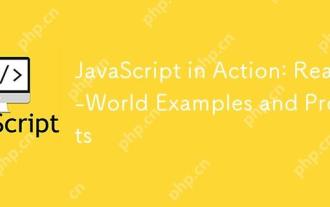
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
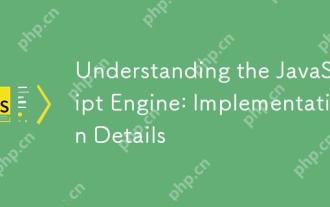
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.
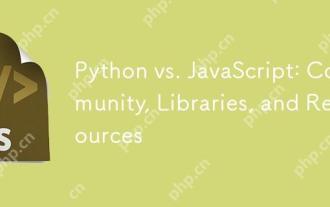
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
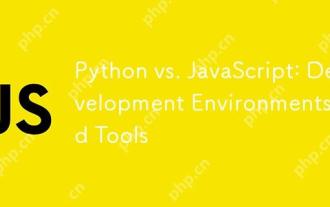
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
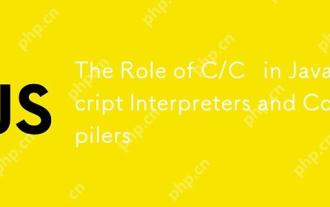
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
