When Should I Use Redis Instead of a Traditional Database?
Use Redis instead of a traditional database when your application requires speed and real-time data processing, such as for caching, session management, or real-time analytics. Redis excels in: 1) Caching, reducing load on primary databases; 2) Session management, simplifying data handling across servers; 3) Real-time analytics, enabling instant data processing and analysis.
When should you use Redis instead of a traditional database? This question often arises when developers are looking to optimize their application's performance and scalability. Redis, an in-memory data structure store, shines in scenarios where speed and real-time data processing are crucial. If your application frequently deals with caching, session management, real-time analytics, or needs to handle high-throughput data operations, Redis is likely a better choice than traditional databases like MySQL or PostgreSQL.
Let's dive deeper into the world of Redis and explore why and when it should be your go-to solution.
Redis is not just another database; it's a powerhouse for handling data in memory, which translates to lightning-fast read and write operations. I've worked on projects where the need for instant data access was paramount. For instance, in a real-time bidding system for an ad platform, we used Redis to store and retrieve bidding data in milliseconds, something a traditional database couldn't handle efficiently.
Another scenario where Redis excels is in caching. Imagine an e-commerce platform where product details are accessed thousands of times per second. Storing this data in Redis as a cache layer significantly reduces the load on your primary database, improving overall system performance. I've seen this approach cut down response times by up to 90% in some cases.
Session management is another area where Redis shines. In a distributed web application, managing user sessions across multiple servers can be a nightmare. Redis, with its ability to store session data in memory and replicate it across nodes, simplifies this process immensely. I once worked on a gaming platform where Redis helped manage millions of concurrent user sessions, ensuring a seamless experience without the overhead of traditional databases.
Real-time analytics is another domain where Redis proves its worth. When you need to process and analyze data as it streams in, Redis's pub/sub messaging model can be a game-changer. I've implemented real-time analytics for a social media platform where Redis helped us analyze user interactions instantly, providing insights that would have been delayed with traditional databases.
However, Redis isn't a silver bullet. It's important to consider its limitations. Redis stores data in memory, which means it's not suitable for storing large amounts of data that don't need immediate access. For long-term data storage, traditional databases are still the better choice. Also, while Redis can persist data to disk, its primary strength lies in its in-memory operations, so if data durability is your top priority, you might want to stick with traditional databases.
When integrating Redis into your application, here are some practical tips and code snippets to get you started:
For caching, you might use Redis like this:
import redis # Initialize Redis client redis_client = redis.Redis(host='localhost', port=6379, db=0) # Set a key-value pair redis_client.set('product:123', 'Laptop') # Get the value product = redis_client.get('product:123') print(product.decode('utf-8')) # Output: Laptop
For session management, you could implement it like this:
import redis import json # Initialize Redis client redis_client = redis.Redis(host='localhost', port=6379, db=0) def set_session(user_id, session_data): # Convert session data to JSON session_json = json.dumps(session_data) # Set session data with expiration time (e.g., 1 hour) redis_client.setex(f'session:{user_id}', 3600, session_json) def get_session(user_id): # Retrieve session data session_json = redis_client.get(f'session:{user_id}') if session_json: return json.loads(session_json.decode('utf-8')) return None # Example usage user_id = 'user123' session_data = {'username': 'john_doe', 'logged_in': True} set_session(user_id, session_data) retrieved_session = get_session(user_id) print(retrieved_session) # Output: {'username': 'john_doe', 'logged_in': True}
For real-time analytics, you might use Redis's pub/sub capabilities:
import redis # Initialize Redis client redis_client = redis.Redis(host='localhost', port=6379, db=0) # Publisher def publish_message(channel, message): redis_client.publish(channel, message) # Subscriber def subscribe_to_channel(channel): pubsub = redis_client.pubsub() pubsub.subscribe(channel) for message in pubsub.listen(): if message['type'] == 'message': print(f"Received message on channel {channel}: {message['data'].decode('utf-8')}") # Example usage channel = 'user_activity' publish_message(channel, 'User logged in') subscribe_to_channel(channel) # This will print: Received message on channel user_activity: User logged in
When using Redis, consider the following best practices and potential pitfalls:
Data Eviction: Redis has several eviction policies (e.g.,
volatile-lru
,allkeys-lru
). Choose the right one based on your use case. I've seen projects struggle with memory issues because they didn't set an appropriate eviction policy.Persistence: While Redis can persist data to disk, it's not as robust as traditional databases. Consider using Redis as a cache and a traditional database for persistent storage.
Scalability: Redis Cluster can help scale your Redis deployment, but it adds complexity. Plan your scaling strategy carefully. I've worked on projects where Redis Cluster was a lifesaver, but it required careful planning and monitoring.
Data Types: Redis supports various data types like strings, lists, sets, and hashes. Use the right data type for your use case to optimize performance. For instance, using a set for unique elements can be more efficient than a list.
Connection Pooling: To handle high concurrency, use connection pooling. I've seen applications slow down because they were creating new connections for every request.
In conclusion, Redis is an incredibly powerful tool for specific use cases like caching, session management, and real-time analytics. However, it's not a replacement for traditional databases but rather a complementary solution that can significantly enhance your application's performance and scalability. By understanding its strengths and limitations, you can make informed decisions on when to leverage Redis in your projects.
The above is the detailed content of When Should I Use Redis Instead of a Traditional Database?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










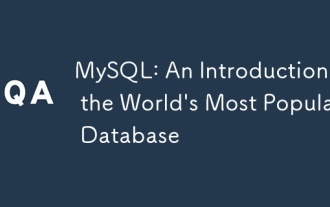
MySQL is an open source relational database management system, mainly used to store and retrieve data quickly and reliably. Its working principle includes client requests, query resolution, execution of queries and return results. Examples of usage include creating tables, inserting and querying data, and advanced features such as JOIN operations. Common errors involve SQL syntax, data types, and permissions, and optimization suggestions include the use of indexes, optimized queries, and partitioning of tables.
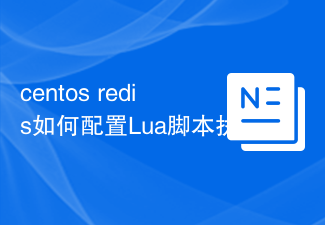
On CentOS systems, you can limit the execution time of Lua scripts by modifying Redis configuration files or using Redis commands to prevent malicious scripts from consuming too much resources. Method 1: Modify the Redis configuration file and locate the Redis configuration file: The Redis configuration file is usually located in /etc/redis/redis.conf. Edit configuration file: Open the configuration file using a text editor (such as vi or nano): sudovi/etc/redis/redis.conf Set the Lua script execution time limit: Add or modify the following lines in the configuration file to set the maximum execution time of the Lua script (unit: milliseconds)
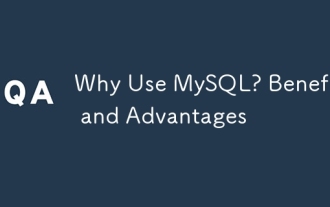
MySQL is chosen for its performance, reliability, ease of use, and community support. 1.MySQL provides efficient data storage and retrieval functions, supporting multiple data types and advanced query operations. 2. Adopt client-server architecture and multiple storage engines to support transaction and query optimization. 3. Easy to use, supports a variety of operating systems and programming languages. 4. Have strong community support and provide rich resources and solutions.
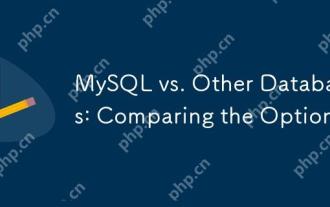
MySQL is suitable for web applications and content management systems and is popular for its open source, high performance and ease of use. 1) Compared with PostgreSQL, MySQL performs better in simple queries and high concurrent read operations. 2) Compared with Oracle, MySQL is more popular among small and medium-sized enterprises because of its open source and low cost. 3) Compared with Microsoft SQL Server, MySQL is more suitable for cross-platform applications. 4) Unlike MongoDB, MySQL is more suitable for structured data and transaction processing.

Oracle is not only a database company, but also a leader in cloud computing and ERP systems. 1. Oracle provides comprehensive solutions from database to cloud services and ERP systems. 2. OracleCloud challenges AWS and Azure, providing IaaS, PaaS and SaaS services. 3. Oracle's ERP systems such as E-BusinessSuite and FusionApplications help enterprises optimize operations.
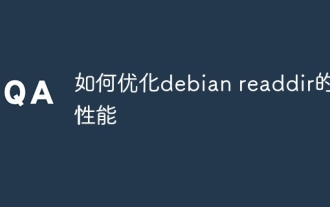
In Debian systems, readdir system calls are used to read directory contents. If its performance is not good, try the following optimization strategy: Simplify the number of directory files: Split large directories into multiple small directories as much as possible, reducing the number of items processed per readdir call. Enable directory content caching: build a cache mechanism, update the cache regularly or when directory content changes, and reduce frequent calls to readdir. Memory caches (such as Memcached or Redis) or local caches (such as files or databases) can be considered. Adopt efficient data structure: If you implement directory traversal by yourself, select more efficient data structures (such as hash tables instead of linear search) to store and access directory information
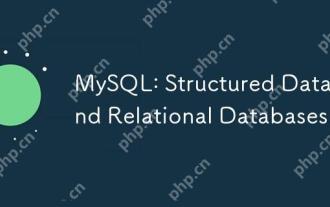
MySQL efficiently manages structured data through table structure and SQL query, and implements inter-table relationships through foreign keys. 1. Define the data format and type when creating a table. 2. Use foreign keys to establish relationships between tables. 3. Improve performance through indexing and query optimization. 4. Regularly backup and monitor databases to ensure data security and performance optimization.
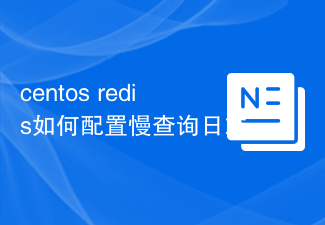
Enable Redis slow query logs on CentOS system to improve performance diagnostic efficiency. The following steps will guide you through the configuration: Step 1: Locate and edit the Redis configuration file First, find the Redis configuration file, usually located in /etc/redis/redis.conf. Open the configuration file with the following command: sudovi/etc/redis/redis.conf Step 2: Adjust the slow query log parameters in the configuration file, find and modify the following parameters: #slow query threshold (ms)slowlog-log-slower-than10000#Maximum number of entries for slow query log slowlog-max-len
