CSS ID and Class: common mistakes
IDs should be used for JavaScript hooks, while classes are better for styling. 1) Use classes for styling to allow for easier reuse and avoid specificity issues. 2) Use IDs for JavaScript hooks to uniquely identify elements. 3) Avoid deep nesting to keep selectors simple and improve performance. 4) Be consistent in your approach to prevent specificity conflicts.
When it comes to CSS, using IDs and classes correctly can be the difference between clean, maintainable code and a messy, hard-to-debug stylesheet. Let's dive into the common mistakes people make with CSS IDs and classes and how to avoid them.
In the world of web development, I've seen many projects where the misuse of CSS selectors led to bloated stylesheets and performance issues. Understanding the nuances of IDs and classes is crucial for any developer looking to write efficient and scalable CSS.
Let's start with a simple example to illustrate the difference between IDs and classes:
/* ID selector */ #header { background-color: #f0f0f0; } /* Class selector */ .nav-item { color: #333; }
In this snippet, #header
targets a unique element with the ID "header," while .nav-item
can be applied to multiple elements with the class "nav-item." This distinction is fundamental, but it's surprising how often it's overlooked.
One common mistake I've encountered is using IDs for styling elements that should be styled with classes. IDs are meant to be unique, and using them for styling can lead to specificity issues and make your CSS harder to override. Here's an example of what not to do:
/* Don't do this */ #button { background-color: blue; color: white; padding: 10px; border: none; }
Instead, use classes for styling reusable components:
/* Do this */ .button { background-color: blue; color: white; padding: 10px; border: none; }
This approach allows you to reuse the style across multiple buttons without the specificity issues that come with IDs.
Another pitfall is nesting selectors too deeply. While it might seem like a good idea to target elements based on their parent-child relationships, it can lead to overly specific selectors that are hard to maintain. Consider this example:
/* Avoid deep nesting */ .header .nav .nav-item .link { color: #333; }
A better approach would be to use a class directly on the element you want to style:
/* Use a class instead */ .nav-link { color: #333; }
This not only makes your CSS more maintainable but also improves performance by reducing the complexity of the selector.
Performance is another area where mistakes with IDs and classes can have a significant impact. Using IDs can be faster for the browser to match, but they can also lead to slower rendering if overused due to the increased specificity. Classes, on the other hand, are more flexible and usually the better choice for styling.
Here's a performance comparison between using IDs and classes:
/* ID selector - faster to match but less flexible */ #main-content { font-size: 16px; } /* Class selector - more flexible but slightly slower to match */ .main-content { font-size: 16px; }
In practice, the difference in performance is usually negligible, but it's worth considering when optimizing your CSS.
One of the most frustrating issues I've dealt with is the specificity wars that arise from using IDs and classes incorrectly. When you start mixing IDs and classes, you can end up with CSS that's hard to override. Consider this scenario:
/* High specificity */ #header .nav-item { color: #333; } /* Lower specificity */ .nav-item { color: #666; }
In this case, the .nav-item
inside #header
will always be #333
due to the higher specificity of the ID selector. To avoid this, stick to a consistent approach, such as using classes for styling and IDs for JavaScript hooks.
Finally, let's talk about best practices for using IDs and classes effectively. Here are some tips I've learned over the years:
- Use classes for styling: Classes are more flexible and allow for easier reuse of styles.
- Use IDs for JavaScript hooks: IDs are great for uniquely identifying elements for JavaScript interactions.
- Avoid deep nesting: Keep your selectors simple and flat to improve maintainability and performance.
- Be consistent: Choose one approach and stick to it to avoid specificity issues.
By understanding these common mistakes and following best practices, you can write cleaner, more efficient CSS that's easier to maintain and scale. Remember, the goal is to make your stylesheets work for you, not against you.
The above is the detailed content of CSS ID and Class: common mistakes. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










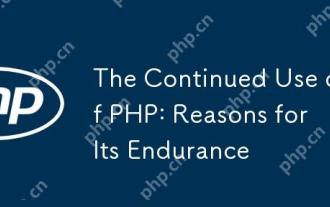
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.

IIS and PHP are compatible and are implemented through FastCGI. 1.IIS forwards the .php file request to the FastCGI module through the configuration file. 2. The FastCGI module starts the PHP process to process requests to improve performance and stability. 3. In actual applications, you need to pay attention to configuration details, error debugging and performance optimization.
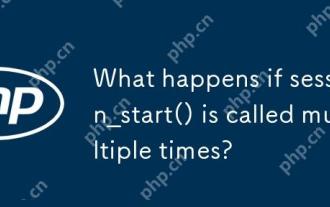
Multiple calls to session_start() will result in warning messages and possible data overwrites. 1) PHP will issue a warning, prompting that the session has been started. 2) It may cause unexpected overwriting of session data. 3) Use session_status() to check the session status to avoid repeated calls.

AI can help optimize the use of Composer. Specific methods include: 1. Dependency management optimization: AI analyzes dependencies, recommends the best version combination, and reduces conflicts. 2. Automated code generation: AI generates composer.json files that conform to best practices. 3. Improve code quality: AI detects potential problems, provides optimization suggestions, and improves code quality. These methods are implemented through machine learning and natural language processing technologies to help developers improve efficiency and code quality.
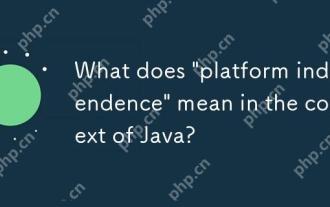
Java's platform independence means that the code written can run on any platform with JVM installed without modification. 1) Java source code is compiled into bytecode, 2) Bytecode is interpreted and executed by the JVM, 3) The JVM provides memory management and garbage collection functions to ensure that the program runs on different operating systems.
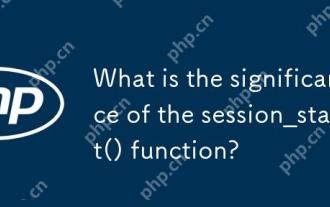
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.

HTML5 brings five key improvements: 1. Semantic tags improve code clarity and SEO effects; 2. Multimedia support simplifies video and audio embedding; 3. Form enhancement simplifies verification; 4. Offline and local storage improves user experience; 5. Canvas and graphics functions enhance the visualization of web pages.
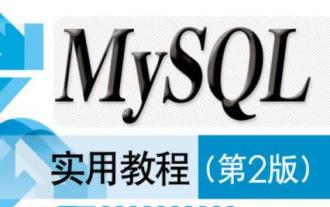
MySQL functions can be used for data processing and calculation. 1. Basic usage includes string processing, date calculation and mathematical operations. 2. Advanced usage involves combining multiple functions to implement complex operations. 3. Performance optimization requires avoiding the use of functions in the WHERE clause and using GROUPBY and temporary tables.
