


Mastering Binary Data Handling with Go's 'encoding/binary' Package: A Comprehensive Guide
The encoding/binary package in Go is essential because it provides a standardized way to read and write binary data, ensuring cross-platform compatibility and handling different endianness. It offers functions like Read, Write, ReadUvarint, and WriteUvarint for precise control over binary data streams, and is crucial for developers working with binary formats.
Diving into the world of Go programming, you'll inevitably encounter the need to handle binary data. Whether it's for network communication, file I/O, or any other low-level operations, understanding how to manipulate binary data efficiently is crucial. This guide aims to master the use of Go's encoding/binary
package, a powerful tool in your Go programming arsenal.
Let's start by tackling a fundamental question: why is the encoding/binary
package essential in Go? The answer lies in its ability to provide a standardized way to read and write binary data. This package is vital for ensuring cross-platform compatibility and handling different endianness, which can be a nightmare without proper tools. It simplifies the process of encoding and decoding data, making it indispensable for developers working with binary formats.
Now, let's delve deeper into the encoding/binary
package. It's not just about reading and writing data; it's about doing so with precision and control. The package offers functions like Read
, Write
, ReadUvarint
, and WriteUvarint
, which cater to different needs, from simple integer operations to more complex variable-length encodings. The beauty of encoding/binary
lies in its flexibility and the fine-grained control it provides over the binary data stream.
Let's explore this with a practical example. Suppose you need to write a 32-bit integer to a file in big-endian format. Here's how you can do it:
package main import ( "encoding/binary" "fmt" "os" ) func main() { file, err := os.Create("binary_data.bin") if err != nil { fmt.Println("Error creating file:", err) return } defer file.Close() value := uint32(42) err = binary.Write(file, binary.BigEndian, value) if err != nil { fmt.Println("Error writing to file:", err) return } fmt.Println("Successfully wrote 42 to the file in big-endian format.") }
This code snippet showcases the simplicity and power of the encoding/binary
package. By specifying binary.BigEndian
, we ensure that the data is written in a format that can be universally understood, which is particularly important in networked applications or when dealing with files that need to be shared across different systems.
However, using encoding/binary
isn't without its challenges. One common pitfall is dealing with endianness. If you're working on a system that uses little-endian and you're writing data for a big-endian system, you need to be meticulous about specifying the correct endianness. A mistake here can lead to data corruption or misinterpretation, which can be catastrophic in certain applications.
Another aspect to consider is performance. While encoding/binary
provides a convenient API, it's not always the most performant solution for high-throughput applications. In such cases, you might need to consider lower-level approaches or even writing custom binary handling code to squeeze out every bit of performance. However, for most applications, the trade-off between ease of use and performance is well worth it.
Let's look at a more advanced example where we read a variable-length integer from a byte slice:
package main import ( "encoding/binary" "fmt" ) func main() { data := []byte{0x96, 0x01} value, n := binary.Uvarint(data) if n <= 0 { fmt.Println("Error decoding varint") return } fmt.Printf("Decoded value: %d\n", value) }
This example demonstrates the use of binary.Uvarint
, which is particularly useful for efficiently encoding integers in a compact form. The function returns both the decoded value and the number of bytes consumed, allowing for precise control over the data stream.
When using encoding/binary
, it's also important to consider error handling. The package returns errors for various conditions, such as when the buffer is too small or when the data cannot be decoded correctly. Properly handling these errors is crucial for writing robust code.
In terms of best practices, always ensure that you're using the correct endianness for your target system. Additionally, consider using io.Reader
and io.Writer
interfaces when working with streams, as they provide a more flexible and reusable approach to handling binary data.
In conclusion, mastering the encoding/binary
package in Go is a game-changer for any developer working with binary data. It provides a robust, efficient, and standardized way to handle binary operations, making it an essential tool in your Go toolkit. Whether you're dealing with network protocols, file formats, or any other binary data, understanding and effectively using encoding/binary
will elevate your Go programming skills to new heights.
The above is the detailed content of Mastering Binary Data Handling with Go's 'encoding/binary' Package: A Comprehensive Guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










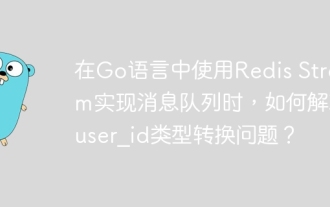
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
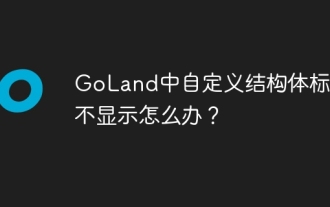
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
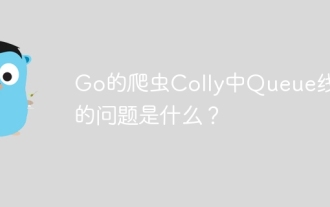
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
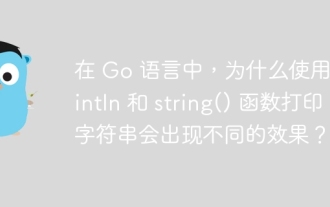
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
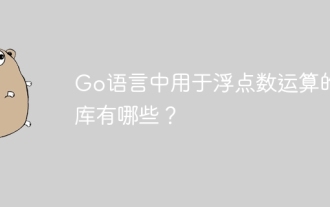
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
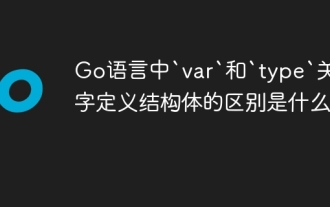
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
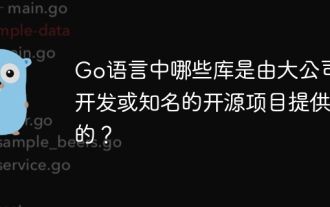
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
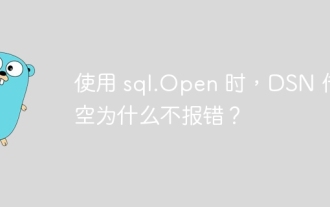
When using sql.Open, why doesn’t the DSN report an error? In Go language, sql.Open...
