Implementing Soft Deletes with Laravel's SoftDeletes Trait
Soft deletes in Laravel are implemented using the SoftDeletes trait, which marks records as deleted without removing them. 1) Add a 'deleted_at' column to your table. 2) Use the SoftDeletes trait in your model. 3) Soft deleted records are excluded from queries by default, but can be included using 'withTrashed' or 'onlyTrashed' methods.
When it comes to managing data in a database, the concept of "soft deletes" is a game-changer. Instead of permanently deleting records, soft deletes allow you to mark them as deleted, keeping your data intact for potential recovery or historical analysis. In Laravel, implementing soft deletes is a breeze thanks to the SoftDeletes
trait. But let's dive deeper into how this works, its advantages, and some pitfalls to watch out for.
Laravel's SoftDeletes
trait is designed to make the process of soft deleting records straightforward and efficient. By using this trait, you can easily mark records as deleted without actually removing them from your database. This approach not only helps in maintaining data integrity but also provides a safety net for accidental deletions. But there's more to it than just marking records as deleted. Let's explore how you can leverage SoftDeletes
to its fullest potential, share some personal experiences, and discuss the nuances that can trip you up if you're not careful.
To get started with SoftDeletes
, you need to add a deleted_at
column to your table. This column will store the timestamp when a record is soft deleted. Here's how you can do it:
use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class AddSoftDeletesToUsersTable extends Migration { public function up() { Schema::table('users', function (Blueprint $table) { $table->softDeletes(); }); } public function down() { Schema::table('users', function (Blueprint $table) { $table->dropSoftDeletes(); }); } }
Once you've added the deleted_at
column, you can use the SoftDeletes
trait in your model:
use Illuminate\Database\Eloquent\Model; use Illuminate\Database\Eloquent\SoftDeletes; class User extends Model { use SoftDeletes; protected $dates = ['deleted_at']; }
Now, when you call the delete
method on a model instance, it will set the deleted_at
column to the current timestamp instead of actually deleting the record:
$user = User::find(1); $user->delete(); // This will soft delete the user
One of the coolest things about SoftDeletes
is how seamlessly it integrates with Eloquent queries. By default, soft deleted records are excluded from query results. But if you want to include them, you can use the withTrashed
method:
$users = User::withTrashed()->get(); // This will include soft deleted users
And if you want to see only the soft deleted records, you can use onlyTrashed
:
$deletedUsers = User::onlyTrashed()->get(); // This will show only soft deleted users
Now, let's talk about some of the advantages and potential pitfalls of using SoftDeletes
. On the plus side, it's incredibly useful for maintaining data integrity and providing a safety net for accidental deletions. It's also great for keeping historical data, which can be invaluable for auditing or analytics purposes.
However, there are some things to watch out for. One common issue is forgetting to include soft deleted records in your queries when you actually need them. This can lead to unexpected results or missing data. Another potential pitfall is the impact on performance, especially if you have a large number of soft deleted records. Over time, these can bloat your database and slow down queries.
From personal experience, I've found that it's crucial to have a clear policy on when and how to permanently delete soft deleted records. Without a proper cleanup strategy, your database can become a mess. I once worked on a project where we had to implement a cron job to periodically clean up soft deleted records older than a certain threshold. It was a lifesaver in keeping our database lean and mean.
Another tip I'd like to share is to always consider the impact of soft deletes on your application's logic. For example, if you're building a user management system, you need to decide whether soft deleted users should still be able to log in or if their access should be revoked immediately. These decisions can have significant implications for your application's security and user experience.
In terms of best practices, I recommend always using withTrashed
or onlyTrashed
explicitly when you need to include soft deleted records in your queries. This makes your code more readable and less prone to errors. Also, consider using scopes to simplify your queries and make your code more maintainable. For example:
class User extends Model { use SoftDeletes; protected $dates = ['deleted_at']; public function scopeActive($query) { return $query->whereNull('deleted_at'); } public function scopeInactive($query) { return $query->whereNotNull('deleted_at'); } }
With these scopes, you can easily query for active or inactive users:
$activeUsers = User::active()->get(); $inactiveUsers = User::inactive()->get();
In conclusion, Laravel's SoftDeletes
trait is a powerful tool for managing data in your application. It offers a lot of flexibility and safety, but it's important to use it wisely and be aware of its potential pitfalls. By following best practices and having a clear strategy for managing soft deleted records, you can leverage SoftDeletes
to build more robust and maintainable applications.
The above is the detailed content of Implementing Soft Deletes with Laravel's SoftDeletes Trait. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










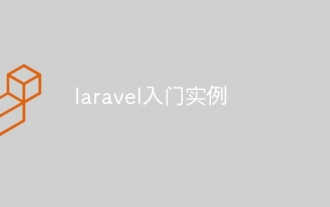
Laravel is a PHP framework for easy building of web applications. It provides a range of powerful features including: Installation: Install the Laravel CLI globally with Composer and create applications in the project directory. Routing: Define the relationship between the URL and the handler in routes/web.php. View: Create a view in resources/views to render the application's interface. Database Integration: Provides out-of-the-box integration with databases such as MySQL and uses migration to create and modify tables. Model and Controller: The model represents the database entity and the controller processes HTTP requests.
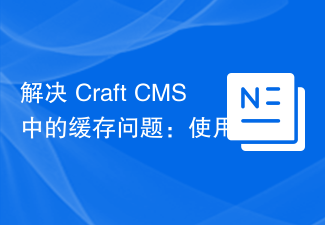
When developing websites using CraftCMS, you often encounter resource file caching problems, especially when you frequently update CSS and JavaScript files, old versions of files may still be cached by the browser, causing users to not see the latest changes in time. This problem not only affects the user experience, but also increases the difficulty of development and debugging. Recently, I encountered similar troubles in my project, and after some exploration, I found the plugin wiejeben/craft-laravel-mix, which perfectly solved my caching problem.
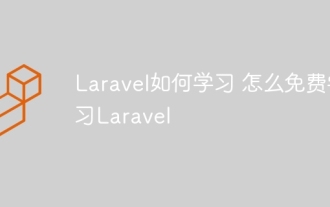
Want to learn the Laravel framework, but suffer from no resources or economic pressure? This article provides you with free learning of Laravel, teaching you how to use resources such as online platforms, documents and community forums to lay a solid foundation for your PHP development journey from getting started to master.
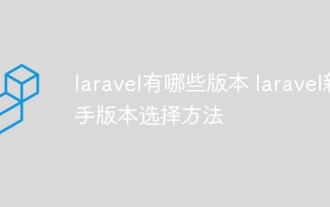
In the Laravel framework version selection guide for beginners, this article dives into the version differences of Laravel, designed to assist beginners in making informed choices among many versions. We will focus on the key features of each release, compare their pros and cons, and provide useful advice to help beginners choose the most suitable version of Laravel based on their skill level and project requirements. For beginners, choosing a suitable version of Laravel is crucial because it can significantly impact their learning curve and overall development experience.
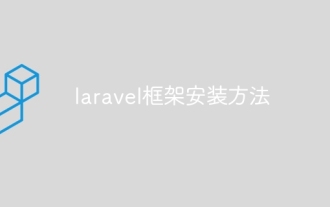
Article summary: This article provides detailed step-by-step instructions to guide readers on how to easily install the Laravel framework. Laravel is a powerful PHP framework that speeds up the development process of web applications. This tutorial covers the installation process from system requirements to configuring databases and setting up routing. By following these steps, readers can quickly and efficiently lay a solid foundation for their Laravel project.
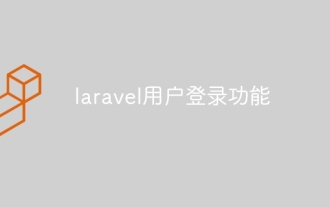
Laravel provides a comprehensive Auth framework for implementing user login functions, including: Defining user models (Eloquent model), creating login forms (Blade template engine), writing login controllers (inheriting Auth\LoginController), verifying login requests (Auth::attempt) Redirecting after login is successful (redirect) considering security factors: hash passwords, anti-CSRF protection, rate limiting and security headers. In addition, the Auth framework also provides functions such as resetting passwords, registering and verifying emails. For details, please refer to the Laravel documentation: https://laravel.com/doc
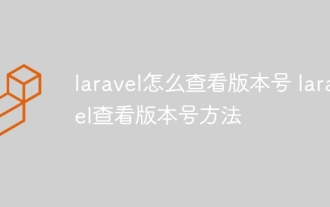
The Laravel framework has built-in methods to easily view its version number to meet the different needs of developers. This article will explore these methods, including using the Composer command line tool, accessing .env files, or obtaining version information through PHP code. These methods are essential for maintaining and managing versioning of Laravel applications.
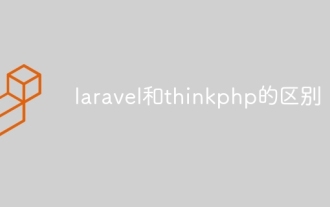
Laravel and ThinkPHP are both popular PHP frameworks and have their own advantages and disadvantages in development. This article will compare the two in depth, highlighting their architecture, features, and performance differences to help developers make informed choices based on their specific project needs.
