What are the alternatives of the 'strings' package in GO?
Go offers alternatives to the strings package for string manipulation: 1) The regexp package for complex pattern matching, 2) The strconv package for numeric conversions, and 3) External libraries like strutil for specialized operations. These options cater to different needs, enhancing your toolkit for efficient string handling in Go.
When it comes to string manipulation in Go, the strings
package is a go-to for many developers. But what if you're looking for alternatives or need something more specialized? Let's dive into the world of string handling in Go and explore some alternatives to the strings
package.
In Go, the strings
package is incredibly versatile, offering functions for searching, replacing, comparing, and splitting strings. However, there are other packages and techniques that can be just as useful, depending on your specific needs. Let's explore some of these alternatives and see how they can enhance your string manipulation toolkit.
One of the most powerful alternatives is the regexp
package. While strings
provides basic string operations, regexp
allows you to perform complex pattern matching and text processing. Here's a quick example of how you might use regexp
to find all email addresses in a string:
package main import ( "fmt" "regexp" ) func main() { text := "Contact us at support@example.com or sales@example.com" re := regexp.MustCompile(`[a-zA-Z0-9._% -] @[a-zA-Z0-9.-] \.[a-zA-Z]{2,}`) matches := re.FindAllString(text, -1) fmt.Println(matches) }
This code uses a regular expression to find all email addresses in the given text. The regexp
package is particularly useful when you need to perform more sophisticated text processing than what strings
can offer.
Another alternative is the strconv
package, which is essential for converting strings to and from numeric types. While not directly a string manipulation package, it's often used in conjunction with string operations. Here's an example of converting a string to an integer:
package main import ( "fmt" "strconv" ) func main() { str := "42" num, err := strconv.Atoi(str) if err != nil { fmt.Println("Error:", err) } else { fmt.Println("Number:", num) } }
This example shows how strconv
can be used to convert a string to an integer, which is a common operation in many applications.
For more advanced string manipulation, you might consider using external libraries like github.com/gookit/goutil/strutil
. This package provides additional string utilities that can be useful for tasks like trimming, padding, and formatting strings. Here's an example of using strutil
to trim whitespace from a string:
package main import ( "fmt" "github.com/gookit/goutil/strutil" ) func main() { str := " Hello, World! " trimmed := strutil.Trim(str) fmt.Println(trimmed) // Output: Hello, World! }
This example demonstrates how strutil
can be used to trim whitespace from a string, which can be more convenient than using the strings
package for certain operations.
When considering alternatives to the strings
package, it's important to think about the specific needs of your project. Here are some insights and considerations:
-
Performance: The
strings
package is highly optimized and built into the Go standard library, making it generally faster than external libraries. However, for complex operations like regular expressions,regexp
might be more efficient. -
Complexity: If your string manipulation needs are simple, sticking with
strings
is usually the best choice. But if you need more advanced features,regexp
or external libraries might be more suitable. -
Learning Curve: The
strings
package is straightforward to use, whileregexp
requires understanding regular expressions, which can be a steeper learning curve. External libraries might also have their own learning curve. -
Maintenance: Using external libraries can introduce dependencies that need to be managed and updated. The
strings
andregexp
packages, being part of the standard library, don't have this issue.
In my experience, I've found that a combination of the strings
and regexp
packages covers most of my string manipulation needs. However, for specific projects, I've used external libraries like strutil
to handle more specialized tasks. The key is to evaluate your project's requirements and choose the tools that best fit those needs.
In conclusion, while the strings
package is a powerful tool for string manipulation in Go, there are several alternatives that can be just as useful depending on your specific needs. Whether you're dealing with complex pattern matching, numeric conversions, or specialized string operations, Go offers a range of options to help you get the job done efficiently and effectively.
The above is the detailed content of What are the alternatives of the 'strings' package in GO?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










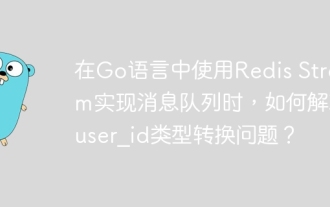
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
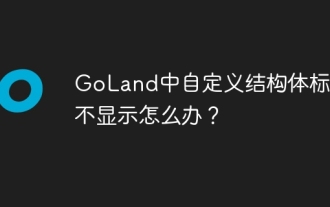
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
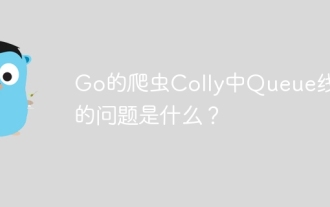
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
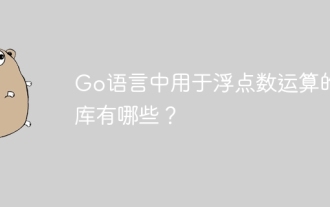
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
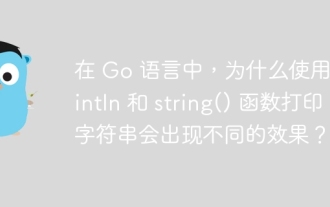
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
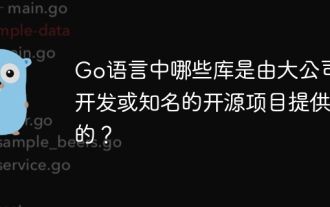
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
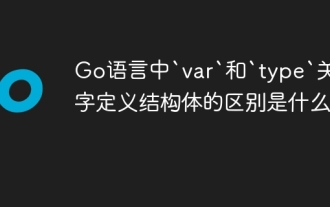
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
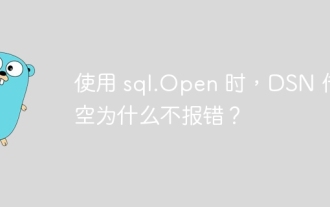
When using sql.Open, why doesn’t the DSN report an error? In Go language, sql.Open...
