


Mastering Byte Slice Manipulation with Go's 'bytes' Package: A Practical Guide
The bytes package in Go is essential for efficient byte slice manipulation, offering functions like Contains, Index, and Replace for searching and modifying binary data. It enhances performance and code readability, making it a vital tool for handling binary data, network protocols, and file I/O operations.
When it comes to working with byte slices in Go, the bytes
package is an invaluable tool. It provides a rich set of functions for manipulating and analyzing byte slices, which are fundamental in Go programming, especially when dealing with binary data, network protocols, or file I/O operations. This guide aims to dive deep into the practical aspects of using the bytes
package, offering insights and examples that will help you master byte slice manipulation.
Let's start by exploring why the bytes
package is essential. Byte slices are ubiquitous in Go because they allow for efficient memory management and are crucial for handling data at the binary level. The bytes
package extends the capabilities of Go's built-in slice type, offering methods to search, compare, and modify byte slices with ease. By the end of this guide, you'll have a solid understanding of how to leverage the bytes
package for your projects, improving both the performance and readability of your code.
Now, let's jump into the world of byte slices and see how the bytes
package can transform your approach to data manipulation.
In Go, byte slices are essentially slices of uint8
, which means they can be used to represent any kind of binary data. Understanding the basics of slices in Go is crucial before diving into the bytes
package. Slices are dynamic, resizable views into arrays, and they're incredibly versatile for handling sequences of data. The bytes
package builds on this foundation, providing functions that make working with binary data more intuitive and efficient.
For instance, the bytes
package includes functions like Contains
, Index
, and Replace
, which are indispensable for searching and modifying byte slices. These functions are optimized for performance, often outperforming manual implementations.
Let's define what the bytes
package does and why it's so powerful. The bytes
package in Go is a collection of utility functions designed specifically for byte slice operations. It's like having a Swiss Army knife for binary data manipulation. The power of the bytes
package lies in its ability to simplify complex operations, making your code cleaner and more maintainable.
Here's a simple example to illustrate the use of the Contains
function from the bytes
package:
package main <p>import ( "bytes" "fmt" )</p><p>func main() { data := []byte("Hello, World!") search := []byte("World")</p><pre class='brush:php;toolbar:false;'>if bytes.Contains(data, search) { fmt.Println("The byte slice contains 'World'") } else { fmt.Println("The byte slice does not contain 'World'") }
}
This example demonstrates how easy it is to check if a byte slice contains a specific sequence of bytes. The Contains
function is straightforward and efficient, making it a go-to tool for such operations.
Now, let's delve into how these functions work under the hood. The Contains
function, for instance, uses a simple linear search algorithm to check for the presence of the search byte slice within the target byte slice. This approach is efficient for small to medium-sized slices but might not be optimal for very large datasets where more sophisticated algorithms might be needed.
Moving on to practical examples, let's explore some common use cases for the bytes
package. One of the most frequent tasks is searching within byte slices. Here's an example using the Index
function to find the position of a substring:
package main <p>import ( "bytes" "fmt" )</p><p>func main() { data := []byte("Hello, World!") search := []byte("World")</p><pre class='brush:php;toolbar:false;'>index := bytes.Index(data, search) if index != -1 { fmt.Printf("Found 'World' at index %d\n", index) } else { fmt.Println("Did not find 'World'") }
}
This example shows how to use Index
to locate the starting position of a byte sequence within another byte slice. It's particularly useful for parsing binary data or processing network packets.
For more advanced scenarios, consider using the Replace
function to modify byte slices. Here's an example that replaces all occurrences of a byte sequence with another:
package main <p>import ( "bytes" "fmt" )</p><p>func main() { data := []byte("Hello, World! Hello, Go!") old := []byte("Hello") new := []byte("Hi")</p><pre class='brush:php;toolbar:false;'>result := bytes.ReplaceAll(data, old, new) fmt.Printf("Modified: %s\n", result)
}
This example demonstrates how ReplaceAll
can be used to perform bulk replacements within a byte slice, which is handy for data transformation tasks.
When working with the bytes
package, it's important to be aware of common pitfalls. One frequent mistake is not handling edge cases properly, such as empty slices or slices with overlapping content. Here's a tip for debugging: always check the return values of functions like Index
and Contains
, as they can return special values like -1
to indicate failure.
To optimize your use of the bytes
package, consider the following best practices. First, when dealing with large datasets, consider using bytes.Buffer
for efficient in-memory manipulation. Here's an example of using bytes.Buffer
to build a byte slice incrementally:
package main <p>import ( "bytes" "fmt" )</p><p>func main() { var buffer bytes.Buffer buffer.WriteString("Hello, ") buffer.WriteString("World!")</p><pre class='brush:php;toolbar:false;'>result := buffer.Bytes() fmt.Printf("Result: %s\n", result)
}
This approach is more efficient than concatenating byte slices directly, especially for large amounts of data.
Another optimization tip is to use bytes.Equal
for comparing byte slices, as it's optimized for performance and handles edge cases better than manual comparisons. Here's an example:
package main <p>import ( "bytes" "fmt" )</p><p>func main() { slice1 := []byte("Hello") slice2 := []byte("Hello")</p><pre class='brush:php;toolbar:false;'>if bytes.Equal(slice1, slice2) { fmt.Println("The byte slices are equal") } else { fmt.Println("The byte slices are not equal") }
}
This example shows how bytes.Equal
can be used to compare byte slices efficiently, which is crucial for tasks like data validation or checksum verification.
In terms of best practices, always aim for readability and maintainability. Use meaningful variable names and add comments to explain complex operations. For instance, when using bytes.Split
, consider adding a comment to explain why you're splitting the byte slice:
package main <p>import ( "bytes" "fmt" )</p><p>func main() { data := []byte("Hello,World,Go") separator := []byte(",")</p><pre class='brush:php;toolbar:false;'>// Split the byte slice into parts using comma as separator parts := bytes.Split(data, separator) for _, part := range parts { fmt.Printf("Part: %s\n", part) }
}
This example demonstrates how to use bytes.Split
to divide a byte slice into smaller parts, which is useful for parsing CSV-like data or similar formats.
In conclusion, mastering the bytes
package in Go can significantly enhance your ability to manipulate and analyze byte slices. By understanding its functions and applying the best practices outlined here, you'll be well-equipped to handle a wide range of binary data processing tasks. Remember, the key to effective use of the bytes
package is not just knowing the functions but also understanding how to apply them in real-world scenarios to optimize performance and maintainability.
The above is the detailed content of Mastering Byte Slice Manipulation with Go's 'bytes' Package: A Practical Guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










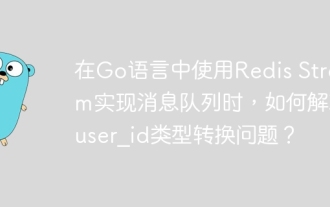
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
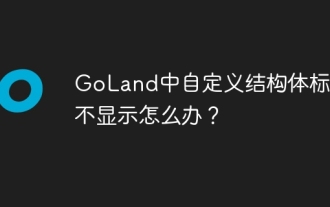
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
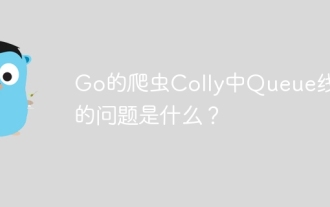
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
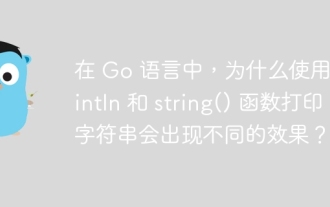
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
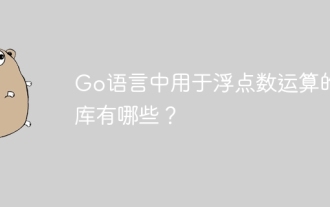
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
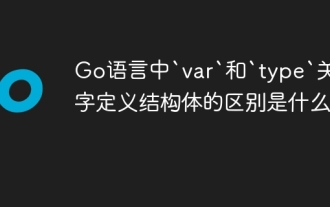
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
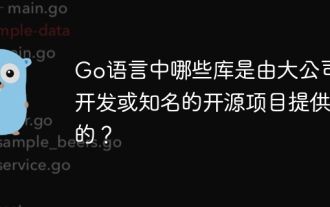
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
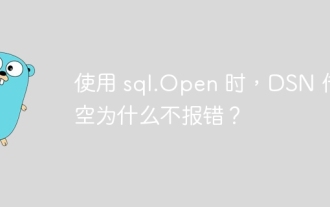
When using sql.Open, why doesn’t the DSN report an error? In Go language, sql.Open...
