


Learn Go Binary Encoding/Decoding: Working with the 'encoding/binary' Package
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as Big Endian or Little Endian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
The binary encoding and decoding of Go language is a magical realm, and the "encoding/binary" package is like a magic wand in this realm, which allows us to easily manipulate data flow. Today we will unveil its mystery and explore how to use it to process binary data.
In Go language, binary encoding and decoding are one of the core skills of data processing. Whether you are processing network protocols, file formats, or need to efficiently store and transfer data, the "encoding/binary" package is an indispensable tool for you. With this package, we can easily convert data into binary format, or extract data from binary format.
Let's start with a simple example and experience the charm of the "encoding/binary" package:
package main import ( "encoding/binary" "fmt" "bytes" ) func main() { // Create a buffer buf := new(bytes.Buffer) // Write a uint16 value var value uint16 = 0x1234 err := binary.Write(buf, binary.LittleEndian, value) if err != nil { fmt.Println("binary.Write failed:", err) } // Read data in the buffer var result uint16 err = binary.Read(buf, binary.LittleEndian, &result) if err != nil { fmt.Println("binary.Read failed:", err) } fmt.Printf("Original value: 0x%X, Read value: 0x%X\n", value, result) }
This example shows how to use the "encoding/binary" package to write a uint16 value to the buffer and then read it out of the buffer. In this way, we can see the conversion process of data in binary format.
The "encoding/binary" package works in that it provides a set of functions that can convert basic data types in Go (such as int, uint, float, etc.) into binary formats, or read these data types from binary formats. Key functions in the package include binary.Write
and binary.Read
, which are used to write and read data respectively.
When using the "encoding/binary" package, we need to pay attention to the endianness issue. Go supports Big Endian and Little Endian, both of which are used in different systems and protocols. Choosing the correct endianness is crucial for the correct decoding of the data.
Let's look at a more complex example showing how to deal with structures:
package main import ( "encoding/binary" "fmt" "bytes" ) type Point struct { X, Y int32 } func main() { // Create a Point structure p := Point{X: 10, Y: 20} // Create a buffer buf := new(bytes.Buffer) // Write to Point structure err := binary.Write(buf, binary.BigEndian, p) if err != nil { fmt.Println("binary.Write failed:", err) } // Read data in the buffer var result Point err = binary.Read(buf, binary.BigEndian, &result) if err != nil { fmt.Println("binary.Read failed:", err) } fmt.Printf("Original Point: %v, Read Point: %v\n", p, result) }
In this example, we define a Point
structure and write and read it using the "encoding/binary" package. It should be noted that the order of fields in the structure must be consistent when writing and reading, otherwise it will lead to data decoding errors.
In practical applications, you may encounter some common problems and misunderstandings when using the "encoding/binary" package. First of all, the selection is the endianness. If the selection is wrong, it will cause the data decoding to fail. The second is the problem of data alignment. Some systems have strict requirements on data alignment. If you do not pay attention to alignment, it may lead to performance problems or data errors.
In order to optimize performance and avoid pitfalls, we can adopt the following strategies:
- Select the correct endianness: Select large endianness or small endianness according to the specific application scenario and protocol requirements.
- Pay attention to data alignment: When defining structures, consider data alignment to avoid performance problems.
- Using buffers: Using
bytes.Buffer
orio.Reader
/io.Writer
interfaces can improve code flexibility and maintainability. - Error handling: When using the "encoding/binary" package, be sure to perform error handling to ensure the correctness of the data.
Through these strategies, we can better utilize the "encoding/binary" package to handle various binary data needs. Whether it is network programming, file processing, or data storage and transmission, the "encoding/binary" package is a powerful tool in our hands. Hope this article helps you better understand and apply this powerful tool.
The above is the detailed content of Learn Go Binary Encoding/Decoding: Working with the 'encoding/binary' Package. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










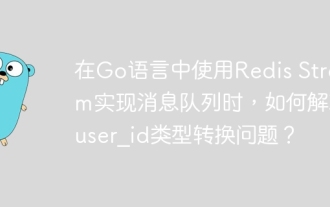
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
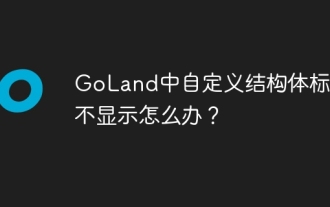
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
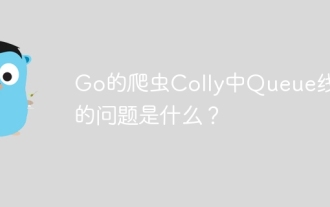
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
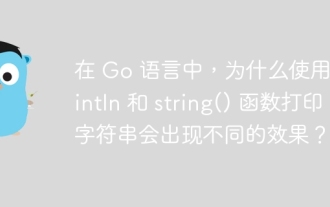
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
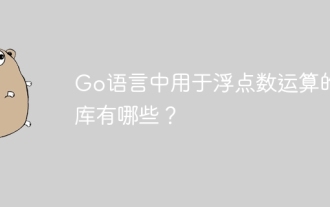
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
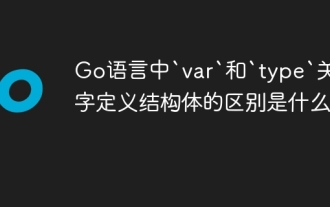
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
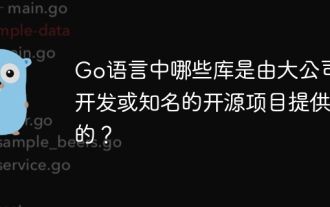
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
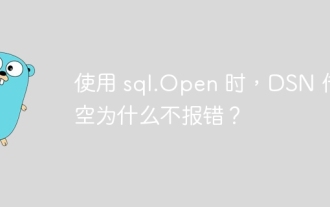
When using sql.Open, why doesn’t the DSN report an error? In Go language, sql.Open...
