Go 'bytes' Package: Compare, Join, Split & More
The "bytes" package in Go is essential because it offers efficient operations on byte slices, crucial for binary data handling, text processing, and network communications. Byte slices are mutable, allowing for performance-enhancing in-place modifications, making this package vital for low-level data manipulation where efficiency is key.
Diving into the Go "bytes" package, let's explore how to use its powerful functions like Compare, Join, Split, and more. Before we dive in, let's tackle a key question:
What makes the "bytes" package essential in Go programming?
The "bytes" package in Go is crucial because it provides efficient operations on byte slices, which are fundamental in handling binary data, text processing, and network communications. Unlike strings, byte slices are mutable, allowing for in-place modifications that can significantly improve performance in certain scenarios. This package is essential for developers working with low-level data manipulation, where every byte counts.
Now, let's delve into the world of the "bytes" package and see how it can transform your Go programming experience.
When I first started working with Go, I was amazed at how the "bytes" package streamlined my data processing tasks. Whether it was parsing binary files or handling network packets, the "bytes" package became my go-to tool. Let's explore some of its key functions and see how they can be applied in real-world scenarios.
Comparing Byte Slices
The Compare
function is a gem when you need to determine the order of two byte slices. It's not just about equality; it's about understanding which slice comes first lexicographically. Here's how you can use it:
package main import ( "bytes" "fmt" ) func main() { slice1 := []byte("apple") slice2 := []byte("banana") result := bytes.Compare(slice1, slice2) if result < 0 { fmt.Println("slice1 comes before slice2") } else if result > 0 { fmt.Println("slice2 comes before slice1") } else { fmt.Println("slice1 and slice2 are equal") } }
This function is particularly useful in sorting algorithms or when you need to maintain a specific order in your data structures. However, be cautious with large slices, as the comparison can be computationally expensive.
Joining Byte Slices
Joining byte slices is a common task, especially when dealing with data that needs to be concatenated. The Join
function in the "bytes" package makes this task straightforward:
package main import ( "bytes" "fmt" ) func main() { slices := [][]byte{[]byte("Hello"), []byte("World")} separator := []byte(" ") result := bytes.Join(slices, separator) fmt.Println(string(result)) // Output: Hello World }
This function is efficient and can handle any number of slices. However, be mindful of the memory allocation, especially with large slices, as it might lead to performance issues.
Splitting Byte Slices
Splitting byte slices is another common operation, particularly when parsing data. The Split
function is incredibly versatile:
package main import ( "bytes" "fmt" ) func main() { data := []byte("one,two,three") separator := []byte(",") result := bytes.Split(data, separator) for _, part := range result { fmt.Println(string(part)) } // Output: // one // two // three }
This function is great for breaking down data into manageable chunks. However, be aware that it creates new slices, which can be memory-intensive for large datasets.
Additional Functions and Tips
The "bytes" package offers many more functions, such as Contains
, Index
, and Replace
, each with its own use case. Here are some tips and insights from my experience:
Contains: Use this to quickly check if a byte slice contains a specific subsequence. It's fast but remember it's case-sensitive.
Index: When you need to find the position of a subsequence,
Index
is your friend. It's efficient but can be slow for very large slices.Replace: This function is great for in-place modifications. However, be cautious with large replacements, as they can lead to significant memory usage.
Performance Considerations
When working with the "bytes" package, performance is a key factor. Here are some insights:
Memory Management: Be mindful of memory allocation, especially with large slices. Functions like
Join
andSplit
can create new slices, which might lead to memory pressure.In-Place Operations: Whenever possible, use in-place operations to minimize memory usage. Functions like
Replace
can be used in-place, which is a significant performance boost.Benchmarking: Always benchmark your code. The "bytes" package is efficient, but the performance can vary based on your specific use case. Use Go's built-in benchmarking tools to fine-tune your code.
Best Practices
From my experience, here are some best practices when using the "bytes" package:
Use Byte Slices for Binary Data: When dealing with binary data, always use byte slices. They are more efficient and allow for in-place modifications.
Avoid Unnecessary Conversions: Try to minimize conversions between strings and byte slices. Each conversion can introduce overhead.
Leverage the Power of Slices: Byte slices are powerful. Use them to your advantage, especially when dealing with large datasets.
In conclusion, the "bytes" package in Go is a powerful tool that can significantly enhance your data processing capabilities. By understanding its functions and applying best practices, you can write more efficient and effective Go code. Whether you're parsing binary files, handling network data, or just manipulating byte slices, the "bytes" package is an essential part of your Go toolkit.
The above is the detailed content of Go 'bytes' Package: Compare, Join, Split & More. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










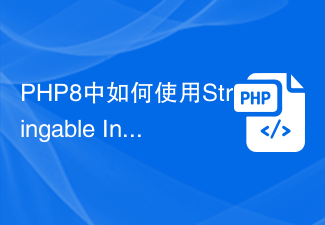
How to use StringableInterface to handle string operations more conveniently in PHP8? PHP8 is the latest version of the PHP language and brings many new features and improvements. One of the improvements that developers are excited about is the addition of StringableInterface. StringableInterface is an interface for handling string operations, which provides a more convenient way to handle and operate strings. This article will detail how to use
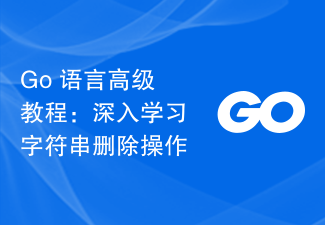
Go language is a very popular programming language, and its powerful features make it favored by many developers. String operations are one of the most common operations in programming, and in the Go language, string deletion operations are also very common. This article will delve into the string deletion operation in the Go language and use specific code examples to help you better understand and master this knowledge point. String deletion operation In the Go language, we usually use the strings package to perform string operations, including deletion operations.
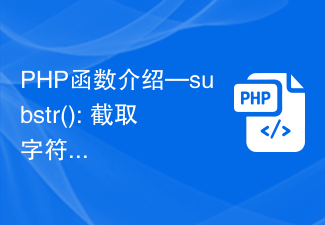
PHP function introduction—substr(): intercepting part of a string In PHP, string processing is a very common operation. For a string, sometimes we need to intercept part of the content for processing. At this time, PHP provides a very practical function-substr(). The substr() function can intercept a part of a string. The specific usage is as follows: stringsubstr(string$string,int
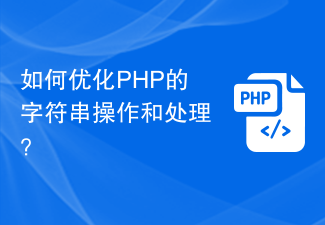
How to optimize PHP's string manipulation and processing? In web development, string manipulation and processing are very common and important parts. For PHP, string optimization can improve program performance and response speed. This article will introduce some methods to optimize PHP string manipulation and processing. Avoid unnecessary string concatenation operations String concatenation operations (using the "." operator) can lead to poor performance, especially within loops. For example, the following code: $str="";for($
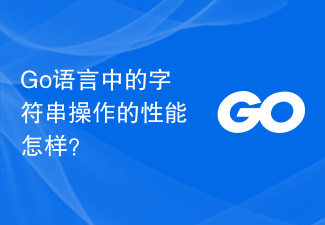
What is the performance of string operations in Go language? In program development, string processing is inevitable, especially in Web development, string processing occurs frequently. Therefore, the performance of string operations is obviously a matter of great concern to developers. So, what is the performance of string operations in Go language? This article will discuss the performance of string operations in Go language from the following aspects. Basic operations Strings in Go language are immutable, that is, once created, the characters in them cannot be modified.
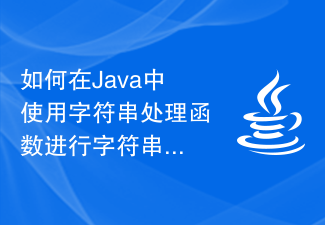
How to use string processing functions for string operations in Java In Java, string is a very common data type. They are used to store and manipulate text data. When processing strings, we often need to perform various operations, such as splicing, search, replacement, cropping, etc. To facilitate processing of strings, Java provides many built-in string processing functions. This article will introduce some commonly used string processing functions and provide specific code examples for reference. String concatenation String concatenation is the concatenation of two or more strings

How to use string processing functions in Java for string manipulation and processing In Java development, string is a very common and important data type. We often need to perform various operations and processing on strings, such as obtaining substrings, concatenating strings, converting case, replacing strings, etc. In order to simplify the process of string manipulation, Java provides many built-in string processing functions. This article will introduce some commonly used string processing functions and give corresponding code examples. Get the string length: use the string object
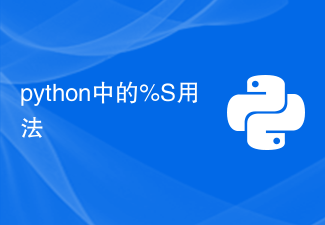
Detailed explanation and code examples of %S usage in Python. In Python, %S is a string formatting method used to insert specified data values into a string. The following will introduce the usage of %S in detail and give specific code examples. Basic usage of %S: %S is used to convert any type of data into a string and insert it into the placeholder in the string. In strings, placeholders are represented by %S. When the Python interpreter encounters %S, it replaces it with the string form of the corresponding data value. Example 1: nam
