Go Strings Package: Essential Functions You Need to Know
Go's strings package includes essential functions like Contains, TrimSpace, Split, and ReplaceAll. 1) Contains efficiently checks for substrings. 2) TrimSpace removes whitespace to ensure data integrity. 3) Split parses structured text like CSV. 4) ReplaceAll transforms text according to rules, useful for data sanitization.
Diving into Go's Strings Package: The Functions You Can't Live Without
When you're diving into the world of Go programming, understanding the strings package becomes essential. It's like the Swiss Army Knife for text manipulation in Go. But what makes these functions so indispensable? Let's explore the core of Go's strings package and why you should care about them.
Let's start by appreciating the elegance of Go's approach to string handling. The strings package is not just a collection of functions; it's a gateway to mastering text processing in Go. From simple operations like trimming whitespace to complex pattern matching with regular expressions, these functions form the backbone of text manipulation in Go applications.
Consider the Contains
function, for example. It's not just about checking if a string is within another; it's about efficiently searching through text, which can be a performance bottleneck in larger applications. Here's a quick example to illustrate:
package main import ( "fmt" "strings" ) func main() { text := "Hello, World!" substring := "World" if strings.Contains(text, substring) { fmt.Println(substring, "is present in", text) } else { fmt.Println(substring, "is not present in", text) } }
This snippet shows how Contains
can be used to check for the presence of a substring. It's straightforward, but the underlying implementation is optimized for performance, which is crucial when dealing with large texts or frequent searches.
Moving on to TrimSpace
, this function is your go-to for cleaning up user inputs or preparing strings for further processing. It's not just about removing spaces; it's about ensuring data integrity and consistency, which is vital in applications dealing with user-generated content.
package main import ( "fmt" "strings" ) func main() { dirtyString := " Hello, World! " cleanString := strings.TrimSpace(dirtyString) fmt.Println("Original:", dirtyString) fmt.Println("Cleaned:", cleanString) }
This example demonstrates how TrimSpace
can transform a string by removing leading and trailing whitespace. It's simple yet powerful, especially in scenarios where data cleanliness is paramount.
Now, let's talk about Split
. This function is a powerhouse for parsing and processing structured text. Whether you're dealing with CSV files, log entries, or any delimited data, Split
is your friend. Here's how you might use it:
package main import ( "fmt" "strings" ) func main() { csvData := "name,age,city" fields := strings.Split(csvData, ",") fmt.Println("Fields:", fields) }
This code showcases Split
in action, turning a CSV-like string into an array of fields. It's essential for data processing tasks, where you need to break down text into manageable parts.
But what about when you're dealing with more complex patterns? That's where ReplaceAll
comes into play. It's not just about replacing text; it's about transforming data according to specific rules, which is crucial for tasks like data sanitization or formatting.
package main import ( "fmt" "strings" ) func main() { original := "The quick brown fox jumps over the lazy dog." modified := strings.ReplaceAll(original, "quick", "slow") fmt.Println("Original:", original) fmt.Println("Modified:", modified) }
This example shows ReplaceAll
at work, replacing all occurrences of "quick" with "slow". It's a simple yet powerful tool for text transformation.
However, while these functions are incredibly useful, there are nuances to consider. For instance, Contains
might not be the best choice for large texts or frequent searches due to its linear time complexity. In such cases, using a more sophisticated search algorithm or leveraging Go's built-in bytes
package for byte-level operations could yield better performance.
Similarly, Split
is great for simple delimiters, but when dealing with more complex formats like JSON or XML, you might need to turn to specialized libraries to handle parsing correctly. And while ReplaceAll
is versatile, it can be inefficient for large texts or when dealing with numerous replacements, where a more tailored approach might be necessary.
In terms of best practices, always consider the performance implications of these functions, especially in high-throughput applications. For instance, if you're frequently trimming spaces from user inputs, consider doing so at the point of input rather than repeatedly in your logic. Also, when using Split
, be mindful of the delimiter; incorrect choices can lead to unexpected results or data loss.
In conclusion, the Go strings package is a treasure trove of functions that can significantly enhance your text processing capabilities. By understanding and leveraging these functions effectively, you can write more efficient, readable, and maintainable Go code. Just remember to consider the context and performance implications of each function to truly master text manipulation in Go.
The above is the detailed content of Go Strings Package: Essential Functions You Need to Know. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










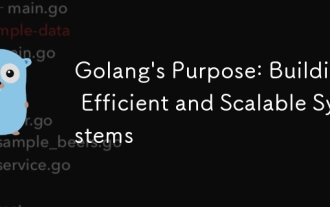
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
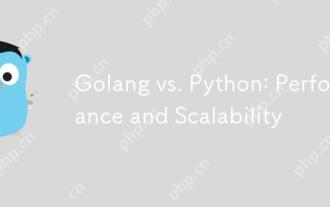
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
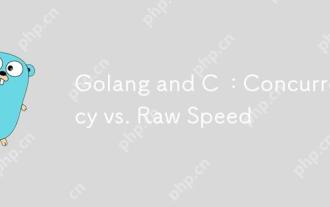
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
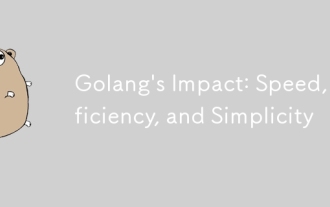
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
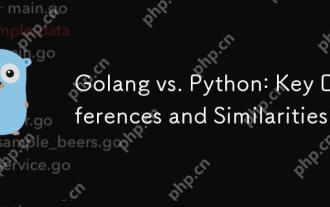
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
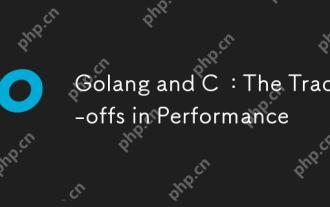
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
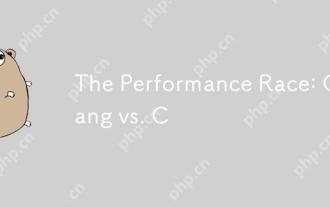
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
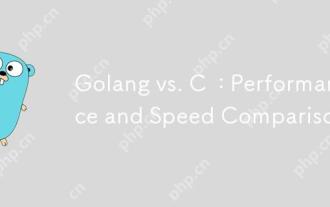
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
