C in Specific Domains: Exploring Its Strongholds
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
introduction
C, the name of this language itself exudes a hardcore atmosphere. As a veteran programming veteran, I have a special emotion for C. It is not just a programming language, but also an art of problem solving. In this challenging programming world, C has a strong position in many specific areas with its powerful performance and flexibility. In this article, we will explore the application and advantages of C in these fields in depth. By reading this article, you will learn about the specific application of C in areas such as game development, embedded systems, financial transactions, and scientific computing, and why it has become the first choice in these areas.
Review of basic knowledge
C is an object-oriented programming language first released by Bjarne Stroustrup in 1983. It combines the low-level operational capabilities of C language with an object-oriented programming paradigm, making it an efficient and flexible programming tool. What makes C powerful is that it offers a rich standard library and powerful performance optimization capabilities, which makes it shine in areas where high performance and low-level control are required.
For example, in game development, C can directly operate hardware to achieve efficient graphics rendering and real-time computing. In embedded systems, C's memory management and hardware control capabilities make it the first choice. In the fields of financial transactions and scientific computing, C's high performance and precise control capabilities have won it a wide range of applications.
Core concept or function analysis
Performance and efficiency of C
C is known for its high performance, which is fully reflected in every particular field. Its compiled language features allow code to be optimized before execution, which is crucial for areas where real-time computing and efficient processing are required.
#include <iostream> int main() { int sum = 0; for (int i = 0; i < 1000000; i) { sum = i; } std::cout << "Sum: " << sum << std::endl; return 0; }
This code demonstrates the efficiency of C in loop calculations. Through compilation optimization, C can unfold loops, reducing branch prediction errors, thereby increasing execution speed.
Memory management and hardware control
C provides strong memory management capabilities, which is particularly important in embedded systems and game development. By manually managing memory, developers can accurately control the resource usage of programs and avoid unnecessary overhead.
#include <iostream> int main() { int* arr = new int[1000]; for (int i = 0; i < 1000; i) { arr[i] = i; } std::cout << "Last element: " << arr[999] << std::endl; delete[] arr; return 0; }
This code shows the dynamic memory allocation and release of C. By manually managing memory, developers can optimize program performance and resource usage according to their needs.
Example of usage
C in game development
In game development, C's performance and flexibility make it the language of choice. Game engines such as Unreal Engine and CryEngine use C as the core development language.
#include <iostream> class GameObject { public: virtual void update() = 0; }; class Player : public GameObject { public: void update() override { std::cout << "Player updated" << std::endl; } }; int main() { Player player; player.update(); return 0; }
This code shows the application of C in game development. With object-oriented programming, developers can easily manage various objects and behaviors in the game.
C in embedded systems
In embedded systems, C's low-level control capabilities and high efficiency make it ideal. Embedded systems are usually limited in resources, and C's memory management and hardware control capabilities can help developers maximize their use of resources.
#include <Arduino.h> void setup() { pinMode(LED_BUILTIN, OUTPUT); } void loop() { digitalWrite(LED_BUILTIN, HIGH); delay(1000); digitalWrite(LED_BUILTIN, LOW); delay(1000); }
This code shows how C is applied on the Arduino platform. By directly operating the hardware, developers can achieve precise control and efficient execution.
C in financial transactions
In the field of financial transactions, C's high performance and precise control capabilities make it the language of choice. Financial transaction systems need to process a large amount of data and perform real-time calculations, and the performance advantages of C are fully reflected here.
#include <iostream> #include <vector> class Trade { public: double price; int quantity; Trade(double p, int q) : price(p), quantity(q) {} }; int main() { std::vector<Trade> trades; trades.push_back(Trade(100.5, 100)); trades.push_back(Trade(101.0, 200)); double totalValue = 0; for (const auto& trade : trades) { totalValue = trade.price * trade.quantity; } std::cout << "Total Value: " << totalValue << std::endl; return 0; }
This code shows the application of C in financial transactions. Through efficient data processing and calculation, C can meet the real-time and accuracy requirements of financial transaction systems.
C in scientific computing
In the field of scientific computing, C's high performance and flexibility make it an important tool. Scientific computing usually involves a large number of data processing and complex algorithms, and the performance advantages of C are fully reflected here.
#include <iostream> #include <vector> #include <cmath> double calculatePi(int iterations) { double pi = 0.0; for (int i = 0; i < iterations; i) { double x = (i 0.5) / iterations; pi = 4.0 / (1.0 x * x); } return pi / iterations; } int main() { int iterations = 1000000; double pi = calculatePi(iterations); std::cout << "Pi: " << pi << std::endl; return 0; }
This code shows the application of C in scientific computing. Through efficient algorithm implementation and data processing, C can meet the high-performance needs of scientific computing.
Performance optimization and best practices
Performance optimization and best practices are critical when using C. Here are some suggestions and experience sharing:
Memory Management : Manually managing memory can significantly improve performance in embedded systems and game development. Using smart pointers can reduce the risk of memory leaks, but it needs to be aware of its performance overhead.
Compilation optimization : Using compiler optimization options can significantly improve the execution efficiency of your code. For example, the
-O3
option enables the highest level of optimization.Parallel computing : In scientific computing and financial transactions, the use of multi-threading and parallel computing can significantly improve performance.
std::thread
andstd::async
introduced in C 11 provide powerful parallel programming support.Code readability : Although C provides strong performance optimization capabilities, keeping the code readability and maintainability is just as important. Using clear naming and annotations can help team members better understand and maintain code.
Avoid common errors : Common errors when using C include memory leaks, uninitialized variables, and out-of-bounds access. Using tools such as Valgrind and AddressSanitizer can help detect and fix these problems.
Overall, C's application in specific fields demonstrates its powerful performance and flexibility. By deeply understanding the characteristics and best practices of C, developers can give full play to their strengths and solve various complex programming problems.
The above is the detailed content of C in Specific Domains: Exploring Its Strongholds. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










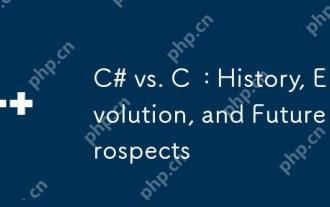
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
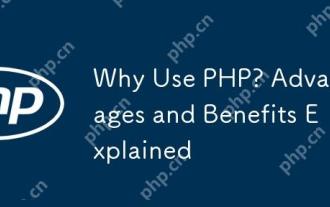
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.
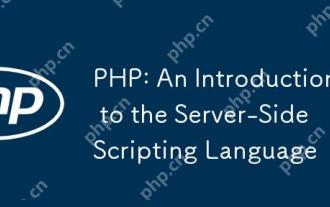
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
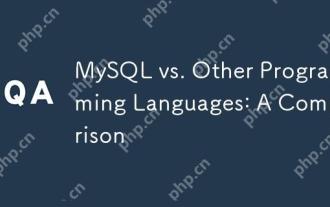
Compared with other programming languages, MySQL is mainly used to store and manage data, while other languages such as Python, Java, and C are used for logical processing and application development. MySQL is known for its high performance, scalability and cross-platform support, suitable for data management needs, while other languages have advantages in their respective fields such as data analytics, enterprise applications, and system programming.
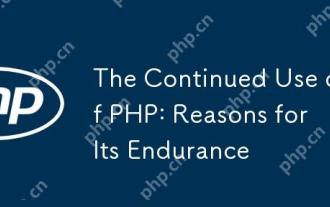
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
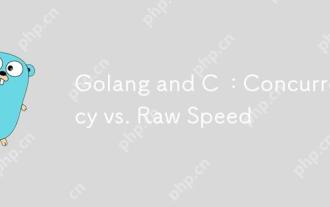
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
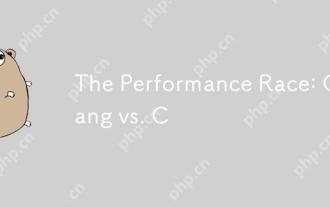
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
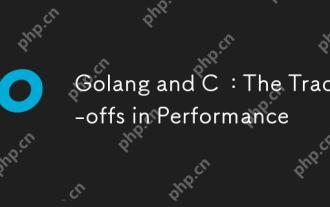
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
