Implicit Interface Implementation in Go: The Power of Duck Typing
Implicit interface implementation in Go embodies duck typing by allowing types to satisfy interfaces without explicit declaration. 1) It promotes flexibility and modularity by focusing on behavior. 2) Challenges include updating method signatures and tracking implementations. 3) Tools like go doc and good documentation practices help manage these issues.
When diving into the world of Go programming, one fascinating aspect you'll encounter is the concept of implicit interface implementation, often referred to as duck typing. So, what exactly is implicit interface implementation in Go, and why does it embody the power of duck typing? In Go, an interface is satisfied implicitly by any type that implements its methods, without needing to explicitly declare that it implements the interface. This approach leverages duck typing, where the type of an object is less important than the methods it implements. This flexibility allows for cleaner, more modular code, but it also introduces some unique challenges and considerations.
Exploring this further, let's delve into the essence of implicit interface implementation in Go and why it's a powerful tool in a developer's arsenal. Go's design philosophy emphasizes simplicity and efficiency, and implicit interface implementation is a perfect embodiment of this ethos. It allows developers to write code that is more focused on behavior rather than on rigid type hierarchies, which can lead to more flexible and maintainable software.
Let's start with a simple example to illustrate how this works:
type Shape interface { Area() float64 } type Circle struct { Radius float64 } func (c Circle) Area() float64 { return 3.14 * c.Radius * c.Radius } type Rectangle struct { Width, Height float64 } func (r Rectangle) Area() float64 { return r.Width * r.Height } func PrintArea(s Shape) { fmt.Printf("Area: %f\n", s.Area()) } func main() { circle := Circle{Radius: 5} rectangle := Rectangle{Width: 3, Height: 4} PrintArea(circle) // Output: Area: 78.500000 PrintArea(rectangle) // Output: Area: 12.000000 }
In this example, Circle
and Rectangle
implicitly implement the Shape
interface because they both have an Area
method. This allows us to pass instances of these types to the PrintArea
function, which expects a Shape
.
The beauty of this approach lies in its simplicity and flexibility. You don't need to explicitly state that Circle
or Rectangle
implements Shape
; the compiler figures it out automatically. This leads to a more dynamic and loosely coupled design, which can be particularly useful in scenarios where you want to add new types without modifying existing code.
However, this power comes with its own set of challenges. One potential pitfall is that if you change the method signature of an interface, all types that implicitly implement it must be updated to match the new signature. This can lead to subtle bugs if not managed carefully. Additionally, because there's no explicit declaration, it can sometimes be harder to track which types implement which interfaces, especially in larger codebases.
In my experience, one effective way to mitigate these issues is to use tools like go doc
or IDEs that can help you navigate the implicit relationships. Additionally, maintaining a good documentation practice, even if it's just in the form of comments, can help other developers understand the implicit implementations.
When considering performance, Go's implicit interface implementation is generally efficient because it's based on static typing. The compiler knows exactly which methods are being called at compile time, which allows for optimizations that might not be possible in dynamically typed languages that also use duck typing.
To wrap up, the power of implicit interface implementation in Go lies in its ability to promote a more flexible and modular approach to programming. By embracing duck typing, Go encourages developers to think in terms of what an object can do, rather than what it is. This can lead to more elegant and maintainable code, but it requires a mindful approach to design and maintenance to avoid potential pitfalls. As with any powerful tool, the key is to use it wisely and understand its implications in the context of your project.
The above is the detailed content of Implicit Interface Implementation in Go: The Power of Duck Typing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










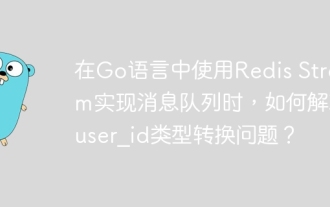
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
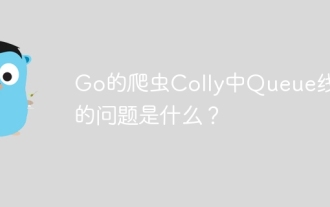
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
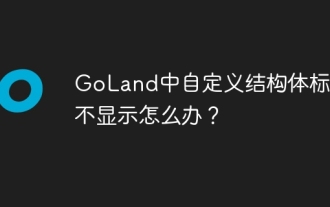
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
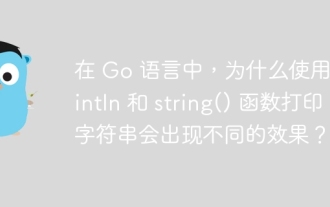
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
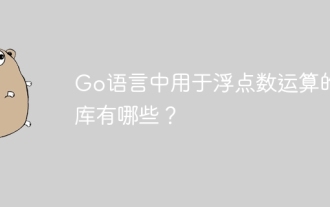
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
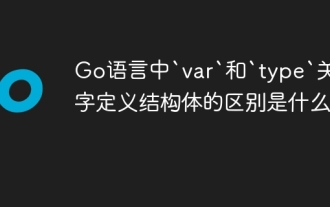
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
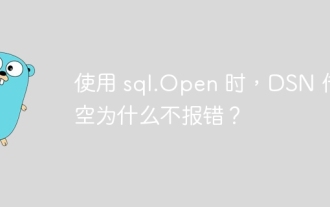
When using sql.Open, why doesn’t the DSN report an error? In Go language, sql.Open...
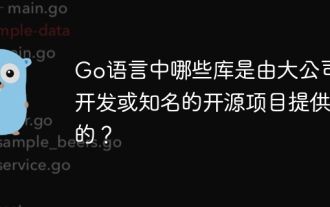
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
