HTML5 Features: The Core of H5
The core features of HTML5 include semantic tags, multimedia support, form enhancement, offline storage and local storage. 1. Semantic tags such as
introduction
The emergence of HTML5 has completely changed the way we build and experience the network. It is not only a new version, but also a brand new way of thinking. Today, we will dive into the core features of HTML5 and reveal how these features make web development more powerful, flexible and efficient. Whether you are a beginner or an experienced developer, after reading this article, you will have a deeper understanding of the core features of HTML5 and be able to better use these features in real-life projects.
A review of the basic HTML
Let's first review the basics of HTML. HTML, full name HyperText Markup Language, is a markup language used to build the structure of a web page. As the fifth version of HTML, HTML5 introduces many new elements and APIs, making web development more intuitive and efficient. Before understanding these new features, we need to know the basic concepts of HTML, such as elements, attributes, tags, etc., which are the basic components of building web pages.
Analysis of the core features of HTML5
Definition and function of semantic labels
HTML5 introduces a series of semantic tags, such as <header></header>
, <footer></footer>
, <nav></nav>
, <article></article>
, etc. The role of these tags is to provide a clearer structure, making web content easier to understand and maintain. Semantic tags not only improve the readability of the code, but also enhance the SEO optimization effect, because search engines can better understand web content.
Let's look at a simple example:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Semantic HTML5 Example</title> </head> <body> <header> <h1 id="Welcome-to-My-Website">Welcome to My Website</h1> </header> <nav> <ul> <li><a href="#home">Home</a></li> <li><a href="#about">About</a></li> </ul> </nav> <article> <h2 id="About-Us">About Us</h2> <p>This is an example of using semantic HTML5 tags.</p> </article> <footer> <p>© 2023 My Website</p> </footer> </body> </html>
This example shows how to use semantic tags to build a simple web structure. Using these tags not only makes the code easier to understand, but also allows assistive technologies such as screen readers to better parse web content.
Multimedia support
A significant feature of HTML5 is its native support for multimedia. With <video>
and <audio>
tags, developers can easily embed video and audio content in web pages without relying on third-party plugins. This not only simplifies the development process, but also improves the user experience.
For example, add a video to a webpage:
<video width="320" height="240" controls> <source src="movie.mp4" type="video/mp4"> <source src="movie.ogg" type="video/ogg"> Your browser does not support the video tag. </video>
This example shows how to use the <video>
tag to embed videos and provide source files in multiple formats to ensure compatibility. It is worth noting that although HTML5's multimedia support is powerful, it also has some challenges, such as different browsers have different levels of support for different formats, so compatibility testing is required in actual applications.
Form enhancement
HTML5 has significantly enhanced the form, introducing new input types and verification properties, such as email
, url
, date
, etc. These new features make form verification simpler and more efficient, reducing dependency on JavaScript.
For example, a simple form:
<form> <label for="email">Email:</label> <input type="email" id="email" name="email" required> <label for="date">Date:</label> <input type="date" id="date" name="date"> <input type="submit" value="Submit"> </form>
This example shows how to create a simple form using new input types and validation properties. Using these new features can greatly simplify the form development process, but it should be noted that different browsers support these features differently, so compatibility testing is required in actual applications.
Offline storage vs. local storage
HTML5 introduces the concepts of offline storage and local storage, allowing web pages to continue working without a network connection. Through Application Cache
, localStorage
and sessionStorage
, developers can store data on users' local devices, improving the performance and user experience of web pages.
For example, use localStorage
to store data:
<script> // Store data localStorage.setItem('username', 'John Doe'); // Get data var username = localStorage.getItem('username'); console.log(username); // Output: John Doe </script>
This example shows how to use localStorage
to store and get data. Using local storage can improve the performance of web pages, but it should be noted that different browsers have different support for local storage and limited storage space, so it needs to be used reasonably in actual applications.
Example of usage
Basic usage
Let's look at some basic usages of HTML5 features:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>HTML5 Basic Example</title> </head> <body> <header> <h1 id="Welcome-to-My-Website">Welcome to My Website</h1> </header> <nav> <ul> <li><a href="#home">Home</a></li> <li><a href="#about">About</a></li> </ul> </nav> <article> <h2 id="About-Us">About Us</h2> <p>This is an example of using HTML5 tags.</p> </article> <footer> <p>© 2023 My Website</p> </footer> <video width="320" height="240" controls> <source src="movie.mp4" type="video/mp4"> <source src="movie.ogg" type="video/ogg"> Your browser does not support the video tag. </video> <form> <label for="email">Email:</label> <input type="email" id="email" name="email" required> <label for="date">Date:</label> <input type="date" id="date" name="date"> <input type="submit" value="Submit"> </form> <script> localStorage.setItem('username', 'John Doe'); var username = localStorage.getItem('username'); console.log(username); // Output: John Doe </script> </body> </html>
This example shows how to use the core features of HTML5 to build a simple web page. Using these features can greatly simplify the development process, but it should be noted that different browsers support these features differently, so compatibility testing is required in actual applications.
Advanced Usage
Let's look at some advanced usages of HTML5 features:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>HTML5 Advanced Example</title> <style> canvas { border: 1px solid black; } </style> </head> <body> <canvas id="myCanvas" width="500" height="300"></canvas> <script> var canvas = document.getElementById('myCanvas'); var ctx = canvas.getContext('2d'); ctx.beginPath(); ctx.arc(95, 50, 40, 0, 2 * Math.PI); ctx.stroke(); </script> <form> <label for="range">Range:</label> <input type="range" id="range" name="range" min="0" max="100" value="50"> <label for="color">Color:</label> <input type="color" id="color" name="color"> <input type="submit" value="Submit"> </form> <script> // Use IndexedDB to store data var request = indexedDB.open('MyDatabase', 1); request.onupgradeneeded = function(event) { var db = event.target.result; var objectStore = db.createObjectStore('users', { keyPath: 'id' }); }; request.onsuccess = function(event) { var db = event.target.result; var transaction = db.transaction(['users'], 'readwrite'); var objectStore = transaction.objectStore('users'); var request = objectStore.add({ id: 1, name: 'John Doe' }); request.onsuccess = function(event) { console.log('Data added successfully'); }; }; </script> </body> </html>
This example shows how to use advanced features of HTML5 such as <canvas>
, new input types, and IndexedDB to build a more complex web page. Using these features can achieve a richer user experience, but it should be noted that different browsers support these features differently, and the use of IndexedDB needs to consider data security and performance issues.
Common Errors and Debugging Tips
There are some common mistakes and challenges you may encounter when using HTML5 features. Here are some common problems and their solutions:
Browser compatibility issues : Different browsers have different support for HTML5 features, which may cause web pages to not be displayed normally on some browsers. The solution is to use feature detection to ensure that the web page works properly on different browsers. For example, use the Modernizr library to detect browser support for HTML5 features.
Form Verification Issues : Although HTML5 form verification is powerful, sometimes verification failure may occur. The solution is to use JavaScript for additional validation to ensure the validity of the form data. For example, use
HTML5 Form Validation API
for custom validation.Multimedia playback problem : Different browsers have different support levels of different video and audio formats, which may cause multimedia to not be played normally. The solution is to provide source files in multiple formats and use the
canPlayType
method to detect the browser's support for different formats. For example:
<video width="320" height="240" controls> <source src="movie.mp4" type="video/mp4"> <source src="movie.ogg" type="video/ogg"> Your browser does not support the video tag. </video> <script> var video = document.querySelector('video'); if (video.canPlayType('video/mp4')) { console.log('MP4 is supported'); } else if (video.canPlayType('video/ogg')) { console.log('OGG is supported'); } else { console.log('No supported format found'); } </script>
This example shows how to use the canPlayType
method to detect the browser's support for different video formats and process it accordingly based on the detection results.
Performance optimization and best practices
Performance optimization and best practices are very important when using HTML5 features. Here are some suggestions:
Using semantic tags : Using semantic tags can improve code readability and SEO optimization effects, and also enable auxiliary technologies such as screen readers to better parse web content.
Optimize multimedia loading : Use the
preload
attribute to control the loading behavior of multimedia to avoid unnecessary waste of resources. For example:
<video width="320" height="240" controls preload="metadata"> <source src="movie.mp4" type="video/mp4"> <source src="movie.ogg" type="video/ogg"> Your browser does not support the video tag. </video>
This example shows how to use the preload
attribute to control the loading behavior of a video to avoid unnecessary waste of resources.
Reasonable use of local storage : When using
localStorage
andsessionStorage
to store data, you need to consider the storage space limitations and data security issues. Avoid storing too much data to avoid affecting the performance of the web page.Use IndexedDB for complex data storage : IndexedDB is suitable for storing large amounts of structured data, but attention should be paid to data security and performance issues. When using IndexedDB, it is necessary to design data structures and indexes reasonably to improve query efficiency.
Perform browser compatibility testing : When using HTML5 features, browser compatibility testing is required to ensure that web pages work normally on different browsers. Use functional detection and progressive enhancement to ensure web page compatibility.
Through these performance optimizations and best practices, the performance and user experience of the web can be greatly improved. I hope this article can help you better understand and apply the core features of HTML5 and achieve better results in actual projects.
The above is the detailed content of HTML5 Features: The Core of H5. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










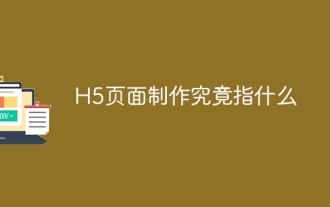
H5 page production refers to the creation of cross-platform compatible web pages using technologies such as HTML5, CSS3 and JavaScript. Its core lies in the browser's parsing code, rendering structure, style and interactive functions. Common technologies include animation effects, responsive design, and data interaction. To avoid errors, developers should be debugged; performance optimization and best practices include image format optimization, request reduction and code specifications, etc. to improve loading speed and code quality.
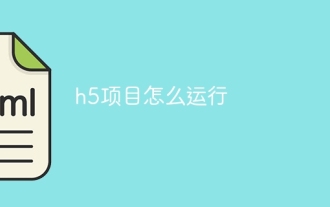
Running the H5 project requires the following steps: installing necessary tools such as web server, Node.js, development tools, etc. Build a development environment, create project folders, initialize projects, and write code. Start the development server and run the command using the command line. Preview the project in your browser and enter the development server URL. Publish projects, optimize code, deploy projects, and set up web server configuration.
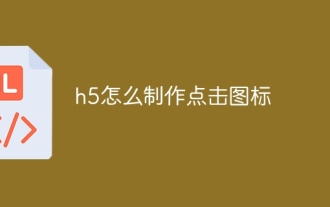
The steps to create an H5 click icon include: preparing a square source image in the image editing software. Add interactivity in the H5 editor and set the click event. Create a hotspot that covers the entire icon. Set the action of click events, such as jumping to the page or triggering animation. Export H5 documents as HTML, CSS, and JavaScript files. Deploy the exported files to a website or other platform.

H5 pop-up window creation steps: 1. Determine the triggering method (click, time, exit, scroll); 2. Design content (title, text, action button); 3. Set style (size, color, font, background); 4. Implement code (HTML, CSS, JavaScript); 5. Test and deployment.
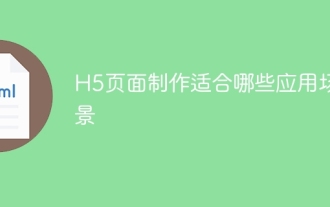
H5 (HTML5) is suitable for lightweight applications, such as marketing campaign pages, product display pages and corporate promotion micro-websites. Its advantages lie in cross-platformity and rich interactivity, but its limitations lie in complex interactions and animations, local resource access and offline capabilities.

H5referstoHTML5,apivotaltechnologyinwebdevelopment.1)HTML5introducesnewelementsandAPIsforrich,dynamicwebapplications.2)Itsupportsmultimediawithoutplugins,enhancinguserexperienceacrossdevices.3)SemanticelementsimprovecontentstructureandSEO.4)H5'srespo

"h5" and "HTML5" are the same in most cases, but they may have different meanings in certain specific scenarios. 1. "HTML5" is a W3C-defined standard that contains new tags and APIs. 2. "h5" is usually the abbreviation of HTML5, but in mobile development, it may refer to a framework based on HTML5. Understanding these differences helps to use these terms accurately in your project.

H5 improves web page accessibility and SEO effects through semantic elements and ARIA attributes. 1. Use, etc. to organize the content structure and improve SEO. 2. ARIA attributes such as aria-label enhance accessibility, and assistive technology users can use web pages smoothly.
