C# vs. C : A Comparative Analysis of Programming Languages
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
introduction
In the programming world, choosing the right language is like choosing a weapon on the battlefield. Today we are comparing two heavyweight weapons: C# and C. The purpose of this article is to help you understand the similarities and differences between the two languages so that you can make informed choices in your project. By reading this article, you will learn about the detailed comparison of C# and C in terms of grammar, performance, application scenarios, etc. At the same time, I will share some practical experience, hoping to bring you some unique insights.
Review of basic knowledge
C# and C are both programming languages developed by Microsoft, but they have different design philosophies and uses. C# is a modern programming language based on the .NET framework, mainly used for the development of Windows applications and games; while C is an object-oriented programming language, widely used in system programming, game development and embedded systems. Understanding this background helps us better understand their differences.
The syntax of C# is similar to Java, providing a garbage collection mechanism, making memory management simpler. C provides lower-level memory management capabilities, allowing developers to perform fine-grained control of memory, which is very important in scenarios with extremely high performance requirements.
Core concept or function analysis
Syntax and features
C# and C have certain syntax similarities, but there are also some significant differences. The syntax of C# is more modern, and supports lambda expressions, LINQ and other features, making the code more concise and easy to read. C, on the other hand, retains more C language features and supports template programming, which can improve code flexibility and performance in some cases.
// The lambda expression Func<int, int, int> sum = (a, b) => ab; Console.WriteLine(sum(3, 4)); // Output: 7
// The lambda expression auto sum = [](int a, int b) { return ab; }; std::cout << sum(3, 4) << std::endl; // Output: 7
Memory management
C# automatically manages memory through a garbage collection mechanism, which greatly simplifies the work of developers, but may also lead to performance losses. C requires developers to manually manage memory, which increases complexity, but also provides higher performance and control capabilities.
// Memory management in C# List<int> numbers = new List<int>(); numbers.Add(1); numbers.Add(2); // The garbage collector will automatically recycle the numbers object
// Memory management in C std::vector<int>* numbers = new std::vector<int>(); numbers->push_back(1); numbers->push_back(2); delete numbers; // Memory needs to be released manually
performance
In terms of performance, C is generally better than C# because it can operate hardware resources directly and avoid the overhead of virtual machines. However, C#'s performance is also constantly being optimized, especially driven by .NET Core, which has been able to meet the needs of most applications.
Example of usage
Basic usage
Let's look at some basic code examples to show the basic usage of C# and C.
// Basic usage in C# using System; class Program { static void Main() { Console.WriteLine("Hello, World!"); } }
// Basic usage in C#include <iostream> int main() { std::cout << "Hello, World!" << std::endl; return 0; }
Advanced Usage
Now let's look at some more complex examples to showcase advanced usage of C# and C.
// Advanced usage in C#: Use LINQ using System; using System.Linq; class Program { static void Main() { int[] numbers = { 1, 2, 3, 4, 5 }; var evenNumbers = numbers.Where(n => n % 2 == 0); foreach (var num in evenNumbers) { Console.WriteLine(num); } } }
// Advanced usage in C: Use STL #include <iostream> #include <vector> #include <algorithm> int main() { std::vector<int> numbers = {1, 2, 3, 4, 5}; std::vector<int> evenNumbers; std::copy_if(numbers.begin(), numbers.end(), std::back_inserter(evenNumbers), [](int n) { return n % 2 == 0; }); for (const auto& num : evenNumbers) { std::cout << num << std::endl; } return 0; }
Common Errors and Debugging Tips
When using C# and C, developers may encounter some common errors and debugging problems. Here are some suggestions:
- Null ReferenceException in C# : In C#, you often encounter NullReferenceException. This error can be avoided by using the null condition operator (?.).
// null reference exception handling in C# string name = null; Console.WriteLine(name?.ToUpper()); // Exception will not be thrown
- Memory Leaks in C : In C, memory leaks are a common problem. The risks of manually managing memory can be avoided by using smart pointers.
// Use #include <memory> in C class MyClass { public: void doSomething() { std::cout << "Doing something" << std::endl; } }; int main() { std::shared_ptr<MyClass> ptr = std::make_shared<MyClass>(); ptr->doSomething(); return 0; }
Performance optimization and best practices
In actual projects, how to optimize the performance of C# and C is a key issue. Here are some suggestions:
- Performance optimization in C# : In C#, you can reduce memory allocation by using
struct
instead ofclass
, and useasync/await
to improve the efficiency of asynchronous operations.
// Performance optimization struct Point in C# { public int X; public int Y; } async Task<int> CalculateAsync() { await Task.Delay(1000); return 42; }
- Performance optimization in C : In C, you can use
constexpr
for compile-time calculations and usestd::move
to avoid unnecessary copying.
// Performance optimization in C constexpr int factorial(int n) { return n <= 1 ? 1 : n * factorial(n - 1); } int main() { std::string s1 = "Hello"; std::string s2 = std::move(s1); // Avoid copying return 0; }
Best Practices
Here are some best practices when writing C# and C code:
Code readability : The readability of the code is crucial regardless of the language used. Use meaningful variable and function names, add appropriate comments and documentation.
Code reuse : Reuse existing code as much as possible to avoid repeated writing of similar logic.
Testing : Write unit tests and integration tests to ensure the correctness and stability of the code.
Through the comparison and analysis of this article, I hope you can have a deeper understanding of C# and C and make more appropriate choices in actual projects. Whether it is the modern syntax and automated memory management of C#, or the low-level control and high performance of C, they have their unique advantages and application scenarios. Which language to choose ultimately depends on the specific needs of the project and the team's technology stack.
The above is the detailed content of C# vs. C : A Comparative Analysis of Programming Languages. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










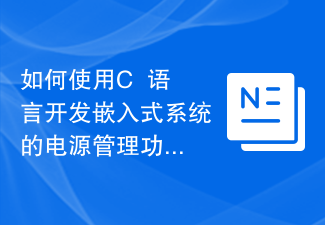
How to use C++ language to develop the power management function of embedded systems. Embedded systems refer to computer systems that run on specific hardware platforms and are designed for specific application fields. The power management function is an indispensable part of the embedded system. It is responsible for managing the system's power supply, power consumption control, power status management and other tasks. This article will introduce how to use C++ language to develop the power management function of embedded systems, with code examples. 1. Basic Principles of Power Management Functions The main goal of power management functions is to minimize system
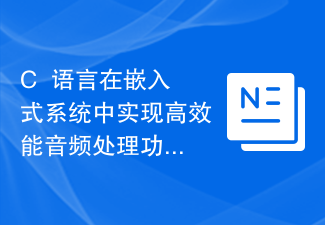
C++ language implements high-performance audio processing functions in embedded systems. Introduction: With the development of technology, the application range of embedded systems is becoming more and more extensive, especially in the fields of Internet of Things, smart home and other fields. Audio processing plays an important role in many embedded systems, such as speech recognition, audio playback, etc. This article will introduce how to use C++ language to implement high-performance audio processing functions in embedded systems and give code examples. 1. Choose a suitable embedded platform. Hardware resources in embedded systems are limited. Choose an embedded system suitable for audio processing.
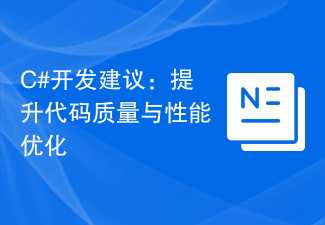
C# development suggestions: Improve code quality and performance optimization In today's software development industry, C#, as a widely used programming language, is used to develop various types of applications, involving projects of various sizes. However, as the scale of projects expands and the functional complexity of software applications increases, developers are often faced with the challenge of improving code quality and performance optimization when developing C# applications. In order to solve these problems, this article will introduce some best practices and suggestions in C# development to help developers improve code quality and performance optimization. 1

C++ language method for realizing high-efficiency IoT communication functions in embedded systems With the rapid development of IoT technology, embedded systems play a key role in realizing high-efficiency IoT communication functions. As an object-oriented high-level programming language, the C++ language has rich features and powerful functions and is widely used in the development of embedded systems. This article will introduce the method of using C++ language to implement high-performance IoT communication functions in embedded systems, and give corresponding code examples. 1. Select the appropriate communication protocol in embedded systems
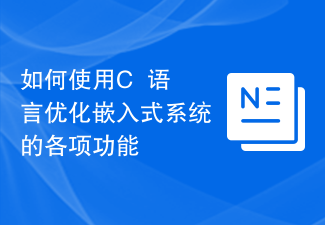
How to use C++ language to optimize various functions of an embedded system. An embedded system is a computer system designed and manufactured specifically for specific tasks. It usually has the characteristics of high real-time requirements, low power consumption, and limited resources. In the development process of embedded systems, how to optimize various functions has become a key task. This article will introduce how to use C++ language to optimize various functions of embedded systems and illustrate it through code examples. 1. Using C++ for memory management optimization In embedded systems, memory management is very important. C++
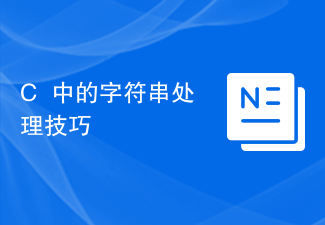
In C++, string is a very common data type. Moreover, string processing is also a very important link in programming. This article will introduce some commonly used string processing techniques in C++, hoping to be helpful to readers. 1. String class in C++ In C++, string is a class contained in the header file <string>. There are many member functions in the string class. Here are some commonly used member functions: 1. append() function
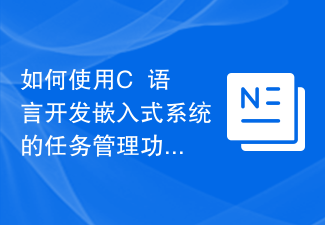
How to use C++ language to develop the task management function of embedded systems. Embedded systems play an important role in modern technology and are widely used in automobiles, smart homes, industrial automation and other fields. Task management is a very important feature for software engineers developing embedded systems. This article will introduce how to use C++ language to develop the task management function of embedded systems and give corresponding code examples. The main goal of task management is to reasonably allocate processor resources and realize the scheduling and execution of each task. in embedded systems
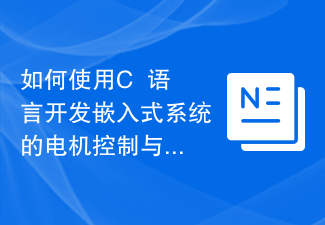
How to use C++ language to develop motor control and drive functions of embedded systems. Embedded systems have been widely used in various industries, especially in the field of motor control and drive. As a high-level programming language, C++ provides a wealth of features and functions, making it easier for developers to develop motor control and drive functions for embedded systems. This article will introduce in detail how to use C++ language to develop the motor control and drive functions of embedded systems, and provide code examples. Hardware Connectivity in Developing Embedded Systems for Motor Control
