Centralized Error Handling Strategies in Go
Centralized error handling can improve the readability and maintainability of code in Go language. Its implementation methods and advantages include: 1. Separate error handling logic from business logic and simplify code. 2. Ensure the consistency of error handling by centrally handling. 3. Use defer and recover to capture and process panics to enhance program robustness.
The centralized error handling strategy of Go language can greatly improve the readability and maintainability of the code. By adopting this strategy, we can better manage errors, avoid duplicate error checking code, and improve development efficiency. Centralized error handling not only simplifies code, but also ensures consistency of error handling, thereby improving the robustness of the entire application.
Error handling has always been an important topic in Go because the design philosophy of Go emphasizes handling errors explicitly, rather than ignoring them. However, traditional error handling often leads to bloat code and is difficult to maintain. Centralized error handling strategies solve this problem by concentrating error handling logic in one place.
Let's dive into the implementation and advantages of centralized error handling in Go.
First of all, we need to understand the core idea of centralized error handling: separate error handling logic from business logic. This not only makes the code clearer, but also makes it easy to modify error handling policies when needed.
Let's look at a simple example showing how to implement centralized error handling in Go:
package main import ( "fmt" "errors" ) // Centralized error handling function func handleError(err error) { if err != nil { fmt.Printf("An error occurred: %v\n", err) // More error handling logic can be added here, such as logging, sending notifications, etc.} } func main() { // Simulate an operation that may have errors err := someOperation() handleError(err) // Another operation that may go wrong err = anotherOperation() handleError(err) } func someOperation() error { return errors.New("something went wrong") } func anotherOperation() error { return nil }
In this example, we define a handleError
function for centralized handling of errors. The advantage of this method is that if we need to modify the error handling logic, such as adding logging or sending notifications, we only need to modify this function without modifying the code that calls it one by one.
However, centralized error handling also has its challenges and needs to be paid attention to. For example, in some cases, we may need to take different measures based on different error types. At this point, we can extend our handleError
function so that it can handle differently according to the error type:
func handleError(err error) { if err != nil { switch err.Error() { case "something went wrong": fmt.Println("A specific error occurred, taking specific action") default: fmt.Printf("An error occurred: %v\n", err) } } }
This approach allows us to handle different error types differently, but also adds complexity. Therefore, we need to find a balance between simplification and flexibility.
In practical applications, another important aspect of centralized error handling is the propagation and recovery of errors. In Go, we can use defer
and recover
to capture and handle panic, thus implementing more complex error handling strategies:
func main() { defer func() { if r := recover(); r != nil { fmt.Println("Recovered from panic:", r) } }() someFunctionThatMightPanic() } func someFunctionThatMightPanic() { panic("something went terribly wrong") }
This approach allows us to use panics anywhere in the program, and centralized error handling functions can capture and process these panics, ensuring the robustness of the program.
In general, the application of centralized error handling in Go language can greatly improve the maintainability and robustness of the code. However, when implementing this strategy, we need to pay attention to the following points:
- Ensure that the error handling function is flexible enough to handle different error types.
- Consider the propagation and recovery of errors, use
defer
andrecover
to capture panic. - Find a balance between simplification and flexibility and avoid overcomplicating error handling logic.
Through these strategies, we can achieve efficient centralized error handling in Go language, improving code quality and development efficiency.
The above is the detailed content of Centralized Error Handling Strategies in Go. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










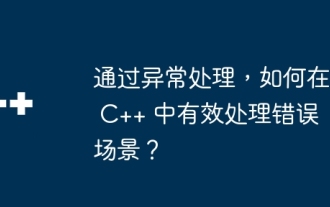
In C++, exception handling handles errors gracefully through try-catch blocks. Common exception types include runtime errors, logic errors, and out-of-bounds errors. Take file opening error handling as an example. When the program fails to open a file, it will throw an exception and print the error message and return the error code through the catch block, thereby handling the error without terminating the program. Exception handling provides advantages such as centralization of error handling, error propagation, and code robustness.
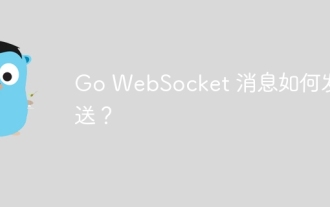
In Go, WebSocket messages can be sent using the gorilla/websocket package. Specific steps: Establish a WebSocket connection. Send a text message: Call WriteMessage(websocket.TextMessage,[]byte("Message")). Send a binary message: call WriteMessage(websocket.BinaryMessage,[]byte{1,2,3}).
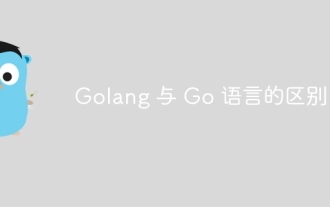
Go and the Go language are different entities with different characteristics. Go (also known as Golang) is known for its concurrency, fast compilation speed, memory management, and cross-platform advantages. Disadvantages of the Go language include a less rich ecosystem than other languages, a stricter syntax, and a lack of dynamic typing.
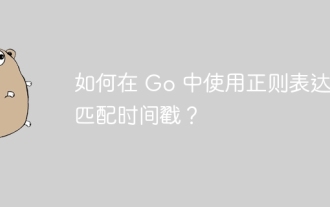
In Go, you can use regular expressions to match timestamps: compile a regular expression string, such as the one used to match ISO8601 timestamps: ^\d{4}-\d{2}-\d{2}T \d{2}:\d{2}:\d{2}(\.\d+)?(Z|[+-][0-9]{2}:[0-9]{2})$ . Use the regexp.MatchString function to check if a string matches a regular expression.
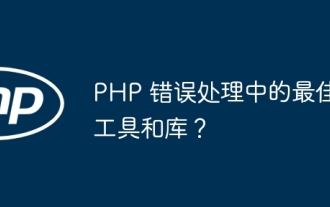
The best error handling tools and libraries in PHP include: Built-in methods: set_error_handler() and error_get_last() Third-party toolkits: Whoops (debugging and error formatting) Third-party services: Sentry (error reporting and monitoring) Third-party libraries: PHP-error-handler (custom error logging and stack traces) and Monolog (error logging handler)
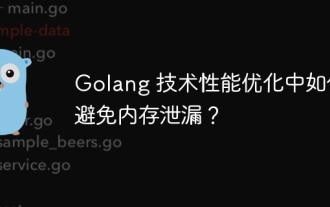
Memory leaks can cause Go program memory to continuously increase by: closing resources that are no longer in use, such as files, network connections, and database connections. Use weak references to prevent memory leaks and target objects for garbage collection when they are no longer strongly referenced. Using go coroutine, the coroutine stack memory will be automatically released when exiting to avoid memory leaks.
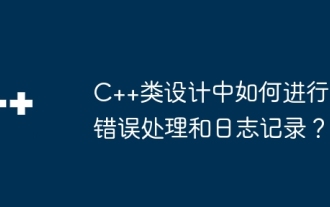
Error handling and logging in C++ class design include: Exception handling: catching and handling exceptions, using custom exception classes to provide specific error information. Error code: Use an integer or enumeration to represent the error condition and return it in the return value. Assertion: Verify pre- and post-conditions, and throw an exception if they are not met. C++ library logging: basic logging using std::cerr and std::clog. External logging libraries: Integrate third-party libraries for advanced features such as level filtering and log file rotation. Custom log class: Create your own log class, abstract the underlying mechanism, and provide a common interface to record different levels of information.
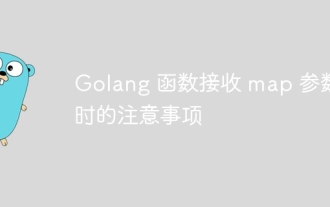
When passing a map to a function in Go, a copy will be created by default, and modifications to the copy will not affect the original map. If you need to modify the original map, you can pass it through a pointer. Empty maps need to be handled with care, because they are technically nil pointers, and passing an empty map to a function that expects a non-empty map will cause an error.
