Redis: A Guide to Key-Value Data Stores
Redis is an open source memory data structure storage used as a database, cache and message broker, suitable for scenarios where fast response and high concurrency are required. 1. Redis uses memory to store data and provides microsecond read and write speed. 2. It supports a variety of data structures, such as strings, lists, collections, etc. 3. Redis realizes data persistence through RDB and AOF mechanisms. 4. Use single-threaded model and multiplexing techniques to handle requests efficiently. 5. Performance optimization strategies include LRU algorithms and cluster mode.
introduction
Redis, this is more than just a name, it is an important tool we often encounter when dealing with high-performance data storage and caching. Today we will explore Redis, a powerful key-value pair data storage system. Whether you're a newbie with Redis or a developer who's already using it but want to dig deeper into its features, this article will take you to see the charm of Redis. We will start with the basics and gradually dive into Redis's advanced usage and performance optimization strategies. After reading this article, you will learn how to effectively utilize Redis to improve your application performance.
Review of basic knowledge
The core of Redis is key-value pair storage, which means it can associate arbitrary types of data with a unique key. Unlike traditional relational databases, Redis is an in-memory database, which gives it a significant advantage in data access speed. Redis not only supports simple string types, but also supports various data structures such as lists, collections, and hash tables. These features make Redis shine in handling caches, session management, real-time analysis and other scenarios.
If you are familiar with NoSQL databases, you may already have some understanding of key-value storage, but Redis is not just a simple key-value storage, it also provides a rich set of commands and functions, such as publish/subscribe mode, transaction support, etc. These functions make Redis more flexible and powerful in practical applications.
Core concept or function analysis
The definition and function of Redis
Redis, abbreviation for Remote Dictionary Server, is an open source memory data structure storage that is used as a database, cache, and message broker. Its function is to provide high-speed data access and operation, suitable for scenarios where fast response and high concurrency are required. The advantage of Redis is its memory storage characteristics, which makes data access faster than traditional disk storage databases.
A simple example of Redis command:
import redis # Connect to Redis server r = redis.Redis(host='localhost', port=6379, db=0) # Set a key-value pair r.set('mykey', 'Hello, Redis!') # Get key value = r.get('mykey') print(value) # Output: b'Hello, Redis!'
This example shows how to use Python's redis library to connect to a Redis server and perform basic setup and fetch operations.
How it works
Redis works based on its memory storage model. The data is stored directly in memory, which allows Redis to read and write operations at microseconds. Redis ensures persistence of data through persistence mechanisms such as RDB and AOF. Although the data is mainly stored in memory, Redis is still able to recover data after restart.
Redis uses a single-threaded model to handle client requests, which seems to be a bottleneck, but in fact, since Redis's I/O operations are non-blocking, the single-threaded model simplifies the design of Redis, allowing it to handle large numbers of requests efficiently. Redis also manages multiple client connections through multiplexing technology, further improving performance.
In terms of performance optimization, Redis provides a variety of strategies, such as using LRU (Least Recently Used) algorithm to manage data in memory to ensure that high-frequency access data will not be easily eliminated. In addition, Redis also supports cluster mode, which can improve the overall performance of the system through horizontal scaling.
Example of usage
Basic usage
The basic usage of Redis mainly revolves around setting and getting key-value pairs. Here is a simple example using Redis that shows how to store and retrieve string data:
import redis # Connect to Redis server r = redis.Redis(host='localhost', port=6379, db=0) # Set a key-value pair r.set('user:name', 'John Doe') # Get key value name = r.get('user:name') print(name) # Output: b'John Doe'
This example shows how to use Redis to store and retrieve user names. With set
and get
commands, we can easily manipulate data in Redis.
Advanced Usage
The power of Redis is that it not only supports simple key-value pair storage, but also supports more complex data structures and operations. For example, Redis's List (List) data structure can be used to implement a queue or stack:
import redis r = redis.Redis(host='localhost', port=6379, db=0) # Add element to the right side of the list r.rpush('mylist', 'item1', 'item2', 'item3') # Pop up an element from the left side of the list item = r.lpop('mylist') print(item) # Output: b'item1' # Get the length of the list length = r.llen('mylist') print(length) # Output: 2
This example shows how to implement a simple queue using Redis's list data structure. You can select different commands to operate on the list according to your needs. For example, rpush
is used to add elements, lpop
is used to remove and get elements, and llen
is used to get the list length.
Common Errors and Debugging Tips
When using Redis, you may encounter some common problems, such as connection problems, data consistency problems, etc. Here are some common errors and debugging tips:
Connection issues : Make sure the Redis server is running and the configured port and host address are correct. If the connection fails, you can try to use the
ping
command to test the Redis server's response:import redis try: r = redis.Redis(host='localhost', port=6379, db=0) response = r.ping() print(response) # If the connection is successful, output: True except redis.ConnectionError: print("Cannot connect to Redis server")
Copy after loginData consistency problem : In high concurrency environments, data consistency problems may be encountered. Redis provides transactional support, and can ensure the atomic execution of a set of commands through
MULTI
andEXEC
commands:import redis r = redis.Redis(host='localhost', port=6379, db=0) # Start a transaction pipe = r.pipeline() pipe.multi() # Add the command pipe.set('user:balance', 100) pipe.incrby('user:balance', 50) # Execute transaction pipe.execute()
Copy after loginBy using transactions, you can ensure that
set
andincrby
commands are executed sequentially and are not interrupted by other commands during execution, thus ensuring data consistency.
Performance optimization and best practices
In practical applications, performance optimization of Redis is a key issue. Here are some common performance optimization strategies and best practices:
Using the right data structure : Redis provides a variety of data structures, and choosing the right data structure can significantly improve performance. For example, if you need to implement a ranking list, you can use an ordered set instead of a list:
import redis r = redis.Redis(host='localhost', port=6379, db=0) # Add user score to the ordered set r.zadd('leaderboard', {'user1': 100, 'user2': 90, 'user3': 80}) # Get top three users top_users = r.zrevrange('leaderboard', 0, 2, withscores=True) print(top_users) # Output: [(b'user1', 100.0), (b'user2', 90.0), (b'user3', 80.0)]
Copy after loginThe
zadd
andzrevrange
commands of ordered collections can efficiently manage and query ranking data, avoiding the performance overhead of sorting using lists.Memory Management : Redis's memory usage is an important consideration. You can limit Redis's
maxmemory
parameter to limit its memory usage and select appropriate memory elimination strategies (such as LRU) to manage data in memory:import redis r = redis.Redis(host='localhost', port=6379, db=0) # Set the maximum memory limit to 1GB r.config_set('maxmemory', '1gb') # Set the memory elimination strategy to LRU r.config_set('maxmemory-policy', 'volatile-lru')
Copy after loginBy rationally configuring Redis's memory parameters, you can effectively control the memory usage of Redis to avoid performance problems caused by insufficient memory.
Code readability and maintenance : When using Redis, it is equally important to keep the code readability and maintenance. You can use the encapsulation method provided by Redis's client library to simplify your code while adding appropriate comments and documentation:
import redis # Connect to Redis server r = redis.Redis(host='localhost', port=6379, db=0) def set_user_balance(user_id, balance): """ Set user balance: param user_id: user ID :param balance: user balance """ key = f'user:{user_id}:balance' r.set(key, balance) def get_user_balance(user_id): """ Get user balance: param user_id: user ID :return: User balance """ key = f'user:{user_id}:balance' return r.get(key) # Use example set_user_balance('user1', 100) balance = get_user_balance('user1') print(balance) # Output: b'100'
Copy after loginBy encapsulating Redis operations into functions and adding detailed comments and documentation, the readability and maintenance of the code can be improved, making it easier for team members to understand and maintain the code.
Redis is a powerful and flexible key-value storage system. By mastering its basic knowledge and advanced usage, it can fully utilize its performance advantages in practical applications. I hope this article can provide you with valuable guidance and inspiration and help you to be at ease in the process of using Redis.
The above is the detailed content of Redis: A Guide to Key-Value Data Stores. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










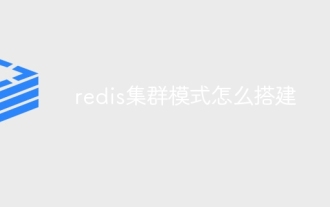
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make
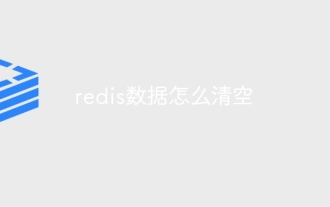
How to clear Redis data: Use the FLUSHALL command to clear all key values. Use the FLUSHDB command to clear the key value of the currently selected database. Use SELECT to switch databases, and then use FLUSHDB to clear multiple databases. Use the DEL command to delete a specific key. Use the redis-cli tool to clear the data.
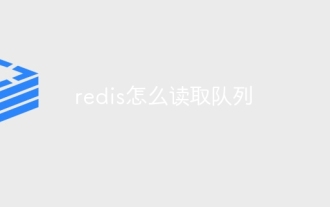
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
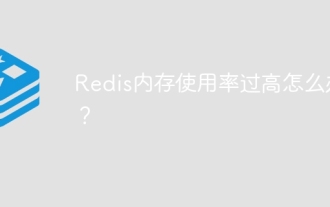
Redis memory soaring includes: too large data volume, improper data structure selection, configuration problems (such as maxmemory settings too small), and memory leaks. Solutions include: deletion of expired data, use compression technology, selecting appropriate structures, adjusting configuration parameters, checking for memory leaks in the code, and regularly monitoring memory usage.

Redis uses a single threaded architecture to provide high performance, simplicity, and consistency. It utilizes I/O multiplexing, event loops, non-blocking I/O, and shared memory to improve concurrency, but with limitations of concurrency limitations, single point of failure, and unsuitable for write-intensive workloads.
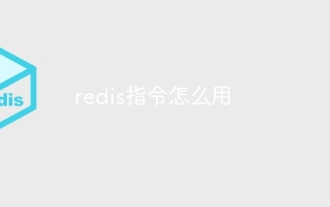
Using the Redis directive requires the following steps: Open the Redis client. Enter the command (verb key value). Provides the required parameters (varies from instruction to instruction). Press Enter to execute the command. Redis returns a response indicating the result of the operation (usually OK or -ERR).
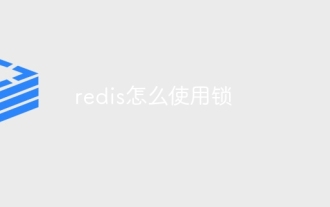
Using Redis to lock operations requires obtaining the lock through the SETNX command, and then using the EXPIRE command to set the expiration time. The specific steps are: (1) Use the SETNX command to try to set a key-value pair; (2) Use the EXPIRE command to set the expiration time for the lock; (3) Use the DEL command to delete the lock when the lock is no longer needed.
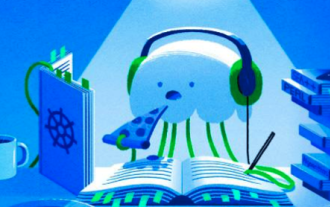
Effective monitoring of Redis databases is critical to maintaining optimal performance, identifying potential bottlenecks, and ensuring overall system reliability. Redis Exporter Service is a powerful utility designed to monitor Redis databases using Prometheus. This tutorial will guide you through the complete setup and configuration of Redis Exporter Service, ensuring you seamlessly build monitoring solutions. By studying this tutorial, you will achieve fully operational monitoring settings
