Beyond Basics: Advanced Techniques in H5 Code
Advanced tips for H5 include: 1. Use <canvas> to draw complex graphics, 2. Use Web Workers to improve performance, 3. Enhance user experience through Web Storage, 4. Implement responsive design, 5. Use WebRTC to achieve real-time communication, 6. Perform performance optimization and best practices. These tips help developers build more dynamic, interactive and efficient web applications.
introduction
When we talk about the advanced tips of HTML5 (H5), we are not just talking about how to create a simple web page. We are exploring how to leverage the power of H5 to build more dynamic, interactive and efficient network applications. This article aims to lead you beyond the basics and into the advanced realms of H5, revealing techniques and techniques that are often overlooked but very useful. By reading this article, you will learn how to use the features of H5 to enhance your web development skills and achieve more complex user experiences and features.
Review of the core of H5
Before we dive into advanced techniques, let’s quickly review the core features of H5. H5 introduces many new elements and APIs, such as <canvas></canvas>
, <video></video>
, <audio></audio>
, Web Storage, Web Workers, etc., which all provide a solid foundation for modern web applications. Understanding these basic elements and APIs is a prerequisite for mastering advanced skills.
Advanced features of the H5
Using Canvas for complex graphics
The <canvas></canvas>
element of H5 provides a powerful platform that allows us to perform complex graphics drawings in the browser. Through JavaScript, we can manipulate <canvas></canvas>
to create animations, games, data visualizations, etc.
const canvas = document.getElementById('myCanvas'); const ctx = canvas.getContext('2d'); function draw() { ctx.clearRect(0, 0, canvas.width, canvas.height); ctx.beginPath(); ctx.arc(95, 50, 40, 0, 2 * Math.PI); ctx.strokeStyle = 'red'; ctx.stroke(); requestAnimationFrame(draw); } draw();
This simple example shows how to draw a red circle on <canvas>
and continuously refresh the screen to achieve basic animation effects.
Web Workers Improve Performance
When processing large amounts of data or performing complex computations, Web Workers can help us avoid blocking the main thread, thereby improving application responsiveness and performance. By running scripts in the background, Web Workers enables us to perform time-consuming tasks without affecting the user interface.
// main.js const worker = new Worker('worker.js'); worker.postMessage({ type: 'start' }); worker.onmessage = function(e) { console.log('Message received from worker', e.data); }; // worker.js self.onmessage = function(e) { if (e.data.type === 'start') { // Const result = performComplexCalculation(); self.postMessage(result); } };
With this example, we can see how to use Web Workers to perform background calculations and send the results back to the main thread.
Enhance user experience with Web Storage
H5's Web Storage API (including localStorage and sessionStorage) provides a convenient way to store data on the client side, which is very useful for improving the user experience. For example, we can store user preferences, save application status, etc.
// Store data localStorage.setItem('theme', 'dark'); // Get data const theme = localStorage.getItem('theme'); // Delete the data localStorage.removeItem('theme');
With these simple API calls, we can easily store and manage data on the client side, resulting in a more personalized user experience.
Advanced tips in applications
Responsive design and H5
Many new features of H5, such as <picture>
elements, srcset
attributes, etc., make it easier to implement responsive design. We can provide different image resources based on the device's screen size and resolution, thereby optimizing the user experience.
<picture> <source srcset="image-small.jpg" media="(max-width: 600px)"> <source srcset="image-medium.jpg" media="(max-width: 1200px)"> <img src="/static/imghw/default1.png" data-src="image-large.jpg" class="lazy" alt="Responsive Image"> </picture>
This example shows how to use the <picture>
element and srcset
attribute to implement responsive image loading.
Real-time communication using WebRTC
H5's WebRTC API makes real-time audio and video communication possible in the browser. This is very useful for building applications such as video conferencing and online education.
const localVideo = document.getElementById('localVideo'); const remoteVideo = document.getElementById('remoteVideo'); navigator.mediaDevices.getUserMedia({ video: true, audio: true }) .then(stream => { localVideo.srcObject = stream; const peerConnection = new RTCPeerConnection(); peerConnection.addStream(stream); // Perform signaling exchange and connection establishment}) .catch(error => console.error('Error accessing media devices.', error));
This example shows how to use the WebRTC API to get local media streams and prepare for real-time communication.
Performance optimization and best practices
Performance optimization and best practices are the key points we must consider when applying advanced H5 skills. Here are some suggestions:
- Optimize resource loading : Use
<link rel="preload">
and<link rel="prefetch">
to load critical resources in advance, thereby reducing loading time. - Code segmentation and lazy loading : Through dynamic import and modular design of JavaScript, code segmentation and lazy loading are implemented to reduce the initial loading time.
- Avoid blocking rendering : Use asynchronous loading scripts, delay loading non-critical resources and other technologies to avoid blocking page rendering.
// Code splitting and lazy loading example import('./module.js').then(module => { // Use module });
Through these tips and best practices, we can ensure that our H5 applications are not only powerful but also perform well.
Summary and prospect
The advanced skills of H5 open up a new world of web development for us. Through the introduction and examples of this article, we see how to leverage the power of H5 to build more complex and efficient web applications. Whether it is drawing with <canvas></canvas>
, improving performance with Web Workers, or enhancing user experience with Web Storage, these tips provide us with more possibilities.
In the future, with the continuous development of H5 and the introduction of new features, we can expect more advanced techniques and application scenarios. I hope this article can inspire you and allow you to continue to explore and innovate on the road of web development.
The above is the detailed content of Beyond Basics: Advanced Techniques in H5 Code. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










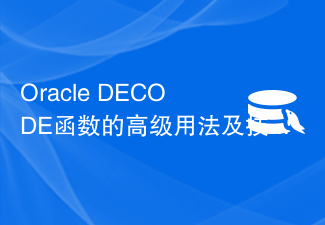
The DECODE function in Oracle database is a very commonly used function, which can select among a set of values based on the result value of an expression. The syntax of the DECODE function is as follows: DECODE(expression, search_value1, result1, search_value2, result2,..., default_result) where expression is the expression to be compared, s
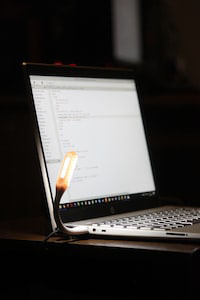
1. Message routing uses JMSSelectors to filter messages: Use JMSSelectors to filter incoming messages based on message attributes and only process relevant messages. Create a custom message router: Extend ActiveMQ's routing capabilities to send messages to specific destinations by writing a custom router. Configure polling load balancing: evenly distribute incoming messages to multiple message consumers to improve processing capabilities. 2. Persistence enables persistent sessions: ensuring that even if the application or server fails, messages can be stored persistently to avoid loss. Configure the Dead Letter Queue (DLQ): Move messages that fail to be processed to the DLQ for reprocessing or analysis. Using Journal Storage: Improve performance of persistent messages, reduce
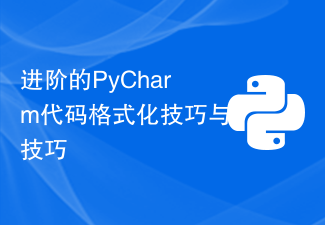
Advanced tips and tricks for PyCharm code formatting Introduction: PyCharm is a popular Python integrated development environment (IDE) that provides a wealth of features and tools to help developers improve development efficiency. One of them is code formatting. Code formatting can make your code cleaner and easier to read, reducing errors and debugging time. This article will introduce some advanced tips and techniques for code formatting in PyCharm and provide specific code examples. Tip 1: Use the automatic formatting shortcut key PyCharm
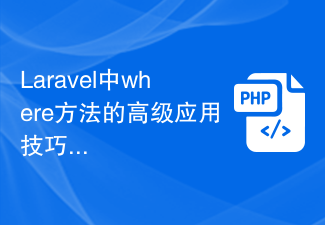
Advanced application tips for where method in Laravel Laravel is a popular PHP development framework that provides many convenient methods to operate the database. Among them, the where method is one of the important methods used to filter database records. In actual development, we often use the where method to query data that meets the conditions. In addition to basic usage, the where method also has some advanced application skills. Here we will share some specific code examples with you. 1.

Best practices for H5 code include: 1. Use correct DOCTYPE declarations and character encoding; 2. Use semantic tags; 3. Reduce HTTP requests; 4. Use asynchronous loading; 5. Optimize images. These practices can improve the efficiency, maintainability and user experience of web pages.
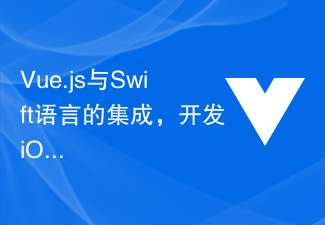
Integration of Vue.js and Swift language, advanced tips for developing iOS applications In modern mobile application development, using the combination of Vue.js and Swift language can provide rich functionality and excellent user experience. Vue.js is a popular JavaScript framework for building user interfaces, while Swift is a powerful and intuitive programming language for developing iOS apps. This article will introduce how to integrate Vue.js in iOS and show some advanced techniques to develop more
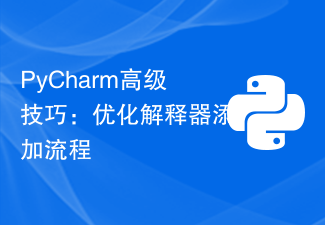
PyCharm is a powerful Python integrated development environment that provides a wealth of functions and tools to facilitate developers to write, debug and manage Python code. Among them, optimizing the interpreter addition process is an advanced technique in PyCharm, which can help developers manage interpreters more effectively and improve development efficiency. In this article, we will introduce how to optimize the interpreter addition process in PyCharm and provide specific code examples. 1. Background introduction In PyCharm, the interpreter is
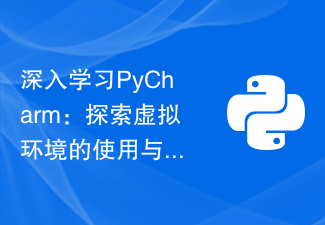
1. The concept and function of PyCharm virtual environment PyCharm is a powerful Python integrated development environment designed to improve developer efficiency and code quality. The virtual environment is a very important concept in PyCharm. It can isolate the dependencies between different projects and ensure that the development environments between projects do not interfere with each other. Creating virtual environments in PyCharm can help developers better manage project dependencies, avoid package conflicts, and better deploy projects to different environments. 2. PyCha
