How do you create a custom type in Go?
How do you create a custom type in Go?
In Go, creating a custom type is a straightforward process that enhances the flexibility and expressiveness of your code. Custom types can be created using several methods, including type aliases, structs, and type definitions. Let's explore each of these methods.
-
Type Alias:
A type alias is a new name for an existing type. You can create a type alias using thetype
keyword followed by the new name and the existing type.type MyInt int
Copy after loginIn this example,
MyInt
is now an alias forint
. This can be useful for readability and to convey additional meaning to the type. Struct:
A struct is a composite data type that groups together zero or more values with different types. You can create a struct type using thestruct
keyword.type Person struct { Name string Age int }
Copy after loginThis defines a new type
Person
that contains fieldsName
andAge
.Type Definition:
A type definition creates a new, distinct type with the same underlying type. It is similar to a type alias but provides more type safety.type MyString string
Copy after loginHere,
MyString
is a new type that has the same underlying type asstring
, but it is considered a different type. This means that you cannot directly assign astring
to aMyString
without a type conversion.
By using these methods, you can create custom types tailored to your specific needs in Go, improving your code's organization and functionality.
What are the benefits of using custom types in Go programming?
Using custom types in Go programming offers several benefits that can significantly enhance your development process and the quality of your code. Here are some key advantages:
- Improved Readability:
Custom types can make your code more readable by providing names that are more descriptive and meaningful. For example,Person
is more informative than a genericstruct
. - Enhanced Type Safety:
With custom types, you can enforce type safety at compile time. For instance, using a distinctMyString
type instead of a regularstring
can prevent unintended type mismatches. - Better Code Organization:
Custom types allow you to organize related data and behaviors into logical units, making your codebase more modular and easier to maintain. - Encapsulation:
By defining methods on custom types, you can encapsulate behavior and data, adhering to the object-oriented programming principles. - Reusability:
Custom types can be reused across different parts of your program, reducing redundancy and improving code efficiency. - Clarity in Documentation:
When using custom types, your documentation becomes clearer and more concise, as the types themselves convey meaning about their purpose and usage.
Can you explain how to use a custom type once it's defined in Go?
Once you have defined a custom type in Go, you can use it in various ways depending on the type of custom type you created. Let's go through some common ways to use custom types.
Using a Type Alias:
If you defined a type alias, you can use it exactly as you would use the underlying type. For instance, if you definedtype MyInt int
, you can useMyInt
in variable declarations, function parameters, or return types.var age MyInt = 30
Copy after loginUsing a Struct:
When you have defined a struct, you can create instances of that struct and access its fields.type Person struct { Name string Age int } person := Person{Name: "Alice", Age: 30} fmt.Println(person.Name) // Output: Alice
Copy after loginYou can also define methods on the struct to encapsulate behavior.
func (p Person) Greet() { fmt.Printf("Hello, my name is %s and I am %d years old.\n", p.Name, p.Age) } person.Greet() // Output: Hello, my name is Alice and I am 30 years old.
Copy after loginUsing a Type Definition:
If you defined a new type liketype MyString string
, you can use it similarly to the underlying type but with type safety. You may need to perform type conversions to assign values.type MyString string var myStr MyString = MyString("Hello") var regularStr string = string(myStr) // Type conversion needed
Copy after login
By using these methods, you can leverage custom types to make your code more expressive and robust.
How do custom types in Go improve code readability and maintainability?
Custom types in Go significantly improve code readability and maintainability in several ways:
-
Descriptive Naming:
Custom types allow you to use names that are more descriptive of the data they represent. For example, usingPerson
instead ofstruct{name string; age int}
makes it immediately clear what the type represents. -
Type Safety:
By defining new types, you can enforce type safety, which helps catch errors at compile time rather than runtime. This reduces the likelihood of bugs and makes the code more maintainable. -
Encapsulation:
Custom types, especially structs, allow you to encapsulate data and behavior. This encapsulation makes it easier to understand and modify the code because related functionality is grouped together. -
Modularity:
Custom types promote modularity by allowing you to break down complex systems into smaller, more manageable parts. This modular approach makes it easier to maintain and extend the codebase. -
Documentation:
Custom types serve as self-documenting code. When someone reads your code, they can quickly understand the purpose and structure of the data without needing extensive comments. -
Consistency:
Using custom types consistently across your codebase helps maintain a uniform style and structure, which is crucial for long-term maintainability. -
Reusability:
Custom types can be reused throughout your program, reducing code duplication and making it easier to update and maintain the code in one place.
By leveraging custom types, you can create more readable, maintainable, and robust Go programs.
The above is the detailed content of How do you create a custom type in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
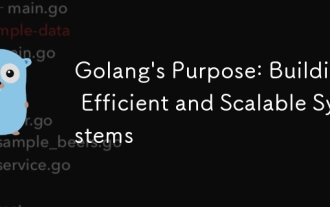
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
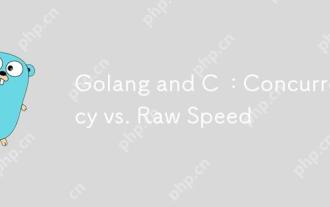
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
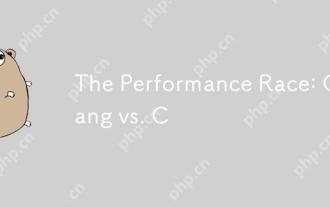
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
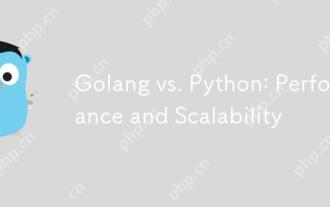
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
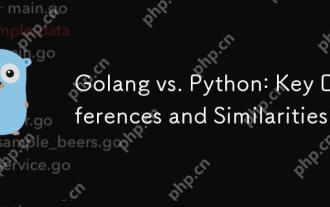
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
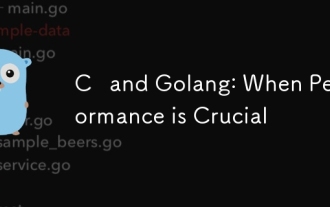
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
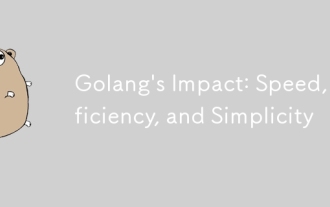
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
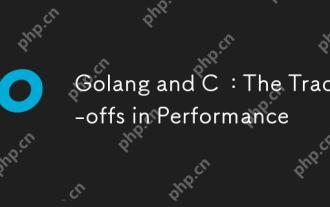
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
