


Describe a scenario where using sessions is essential in a web application.
Sessions are vital in web applications, especially for e-commerce platforms. They maintain user data across requests, crucial for shopping carts, authentication, and personalization. In Flask, sessions can be implemented using simple code to manage user logins and data persistence.
Sessions are absolutely vital in web applications, and I'll dive into a scenario where they make all the difference.
Imagine you're building an e-commerce platform. Users are constantly navigating through product listings, adding items to their carts, and eventually checking out. Now, without sessions, every time a user clicks to another page or refreshes, they'd lose their shopping cart contents. Not ideal, right? Sessions come to the rescue by maintaining a persistent state across multiple requests.
Let's dig deeper into how sessions can be implemented and why they're so crucial.
In an e-commerce setting, sessions are essential for keeping track of user activities like:
- Shopping Cart: As users browse and add items to their cart, the session stores this information, ensuring the cart remains intact no matter how many pages they visit.
- User Authentication: Once a user logs in, the session keeps them authenticated, so they don't have to re-enter their credentials on every page.
- Personalization: Sessions can store user preferences, such as language settings or recently viewed items, enhancing the user experience.
Here's a quick look at how you might implement a session in Python using Flask:
from flask import Flask, session, redirect, url_for, request app = Flask(__name__) app.secret_key = 'your_secret_key_here' # This is needed for session security @app.route('/') def index(): if 'username' in session: return f'Logged in as {session["username"]}' return 'You are not logged in' @app.route('/login', methods=['GET', 'POST']) def login(): if request.method == 'POST': session['username'] = request.form['username'] return redirect(url_for('index')) return ''' <form method="post"> <p><input type=text name=username> <p><input type=submit value=Login> </form> ''' @app.route('/logout') def logout(): # remove the username from the session if it's there session.pop('username', None) return redirect(url_for('index'))
This code snippet demonstrates the basics of session management in Flask. It's simple, yet effective for our e-commerce scenario.
Now, let's talk about the pros and cons of using sessions and some potential pitfalls:
Advantages:
- State Persistence: Sessions keep user data across multiple requests, which is crucial for maintaining things like shopping carts.
- Security: When properly configured, sessions can provide a secure way to manage user authentication.
- User Experience: They enable personalization, making the user's journey through the site more enjoyable.
Disadvantages:
- Server Load: Sessions can increase server load, especially if you're dealing with a high number of concurrent users.
- Scalability: In a distributed system, managing sessions across multiple servers can be tricky.
Pitfalls to Watch Out For:
- Session Hijacking: If not secured properly, sessions can be vulnerable to attacks where an attacker gains access to a user's session ID.
- Session Expiration: You need to manage session timeouts to prevent users from staying logged in indefinitely.
- Data Overload: Storing too much data in sessions can lead to performance issues.
In my experience, one of the best practices is to use a combination of cookies and server-side sessions. Cookies can store non-sensitive data, while the server manages sensitive session data. This approach helps balance security and performance.
For instance, in a large e-commerce platform I worked on, we used Redis as a session store to handle high concurrency and ensure scalability. It worked wonders, but setting it up required careful consideration of session serialization and deserialization.
To wrap up, sessions are indispensable for any web application requiring state management, especially in scenarios like e-commerce where user data persistence is key. Just be mindful of the potential drawbacks and implement best practices to ensure a secure and smooth user experience.
The above is the detailed content of Describe a scenario where using sessions is essential in a web application.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
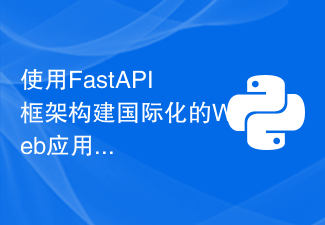
Use the FastAPI framework to build international Web applications. FastAPI is a high-performance Python Web framework that combines Python type annotations and high-performance asynchronous support to make developing Web applications simpler, faster, and more reliable. When building an international Web application, FastAPI provides convenient tools and concepts that can make the application easily support multiple languages. Below I will give a specific code example to introduce how to use the FastAPI framework to build
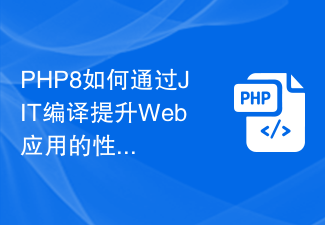
How does PHP8 improve the performance of web applications through JIT compilation? With the continuous development of Web applications and the increase in demand, improving the performance of Web applications has become one of the focuses of developers. As a commonly used server-side scripting language, PHP has always been loved by developers. The JIT (just-in-time compilation) compiler was introduced in PHP8, providing developers with a new performance optimization solution. This article will discuss in detail how PHP8 can improve the performance of web applications through JIT compilation, and provide specific code examples.
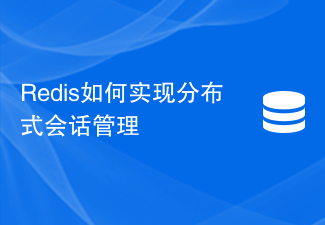
How Redis implements distributed session management requires specific code examples. Distributed session management is one of the hot topics on the Internet today. In the face of high concurrency and large data volumes, traditional session management methods are gradually becoming inadequate. As a high-performance key-value database, Redis provides a distributed session management solution. This article will introduce how to use Redis to implement distributed session management and give specific code examples. 1. Introduction to Redis as a distributed session storage. The traditional session management method is to store session information.
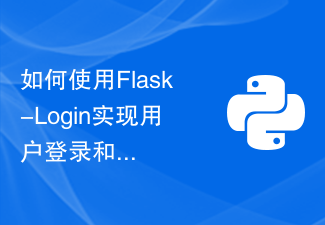
How to use Flask-Login to implement user login and session management Introduction: Flask-Login is a user authentication plug-in for the Flask framework, through which we can easily implement user login and session management functions. This article will introduce how to use Flask-Login for user login and session management, and provide corresponding code examples. 1. Preparation Before using Flask-Login, we need to install it in the Flask project. You can use pip with the following command
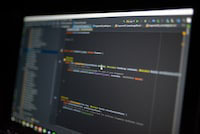
This article will explain in detail about starting a new or restoring an existing session in PHP. The editor thinks it is very practical, so I share it with you as a reference. I hope you can gain something after reading this article. PHP Session Management: Start a New Session or Resume an Existing Session Introduction Session management is crucial in PHP, it allows you to store and access user data during the user session. This article details how to start a new session or resume an existing session in PHP. Start a new session The function session_start() checks whether a session exists, if not, it creates a new session. It can also read session data and convert it
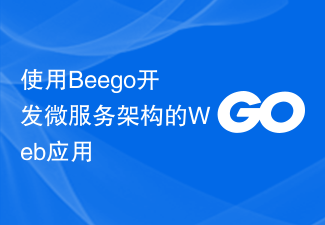
With the development of the Internet and the popularity of applications, the demand for Web applications has also continued to grow. In order to meet the needs of a large number of users, traditional web applications often face performance bottlenecks and scalability issues. In response to these problems, microservice architecture has gradually become a trend and solution for web application development. In the microservice architecture, the Beego framework has become the first choice of many developers. Its efficiency, flexibility, and ease of use are deeply loved by developers. This article will introduce the use of Beego framework to develop web applications with microservice architecture.
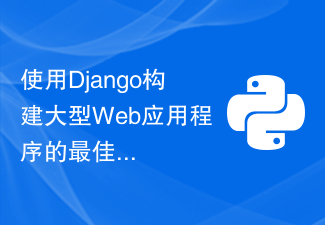
With the popularity and development of the Internet, Web applications have become an indispensable and important part of today's society. For large-scale web applications, an efficient, scalable, and maintainable framework is essential. Under such circumstances, Django has become a popular web framework because it adopts many best practices to help developers quickly build high-quality web applications. In this article, we will introduce some best practices for building large-scale web applications using Django.

In-depth study of the underlying development principles of PHP: session management and state retention methods Preface In modern web development, session management and state retention are very important parts. Whether it is the maintenance of user login status or the maintenance of shopping cart and other status, session management and status maintenance technology are required. In the underlying development of PHP, we need to understand the principles and methods of session management and state retention in order to better design and tune our web applications. The basic session of session management refers to the client and server
