How to process sensor data in C?
C++适合处理传感器数据,因为其高性能和低级控制能力。具体步骤包括:1. 数据采集:通过硬件接口获取数据。2. 数据解析:将原始数据转换为可用信息。3. 数据处理:进行滤波和平滑处理。4. 数据存储:保存数据到文件或数据库。5. 实时处理:确保代码的高效性和低延迟。
在C++中处理传感器数据,这是一个既有趣又具有挑战性的任务。你可能会问,"为什么要用C++来处理传感器数据?" 答案很简单:C++的高性能和低级控制能力,使得它成为处理实时数据的理想选择,特别是当你需要直接与硬件交互时。
当我们谈到传感器数据的处理时,C++提供了丰富的工具和库,让你可以高效地从传感器读取数据,对其进行处理,并将结果存储或发送到其他系统。让我们深入探讨一下如何在C++中处理传感器数据。
首先,我们需要了解传感器数据的基本结构。传感器通常会以某种格式输出数据,比如通过串口、I2C、SPI等接口。这些数据可能是原始的,需要我们进行解析和处理。
// 读取传感器数据的示例 #include <iostream> #include <fstream> int main() { std::ifstream sensorFile("sensor_data.txt"); if (!sensorFile.is_open()) { std::cerr << "无法打开传感器数据文件!" << std::endl; return 1; } double temperature; while (sensorFile >> temperature) { std::cout << "当前温度: " << temperature << " °C" << std::endl; } sensorFile.close(); return 0; }
上面的代码展示了如何从一个文件中读取传感器数据。这只是一个简单的示例,实际应用中你可能需要处理来自硬件接口的数据。
处理传感器数据时,我们需要考虑几个关键点:
- 数据采集:如何从传感器获取数据?这可能涉及到硬件接口的编程,比如使用Arduino或Raspberry Pi来读取数据。
- 数据解析:传感器数据通常是原始的,需要进行解析。例如,温度传感器可能会输出一个电压值,你需要将其转换为实际的温度值。
- 数据处理:一旦获取了数据,你可能需要对其进行滤波、平滑处理,或者进行统计分析。
- 数据存储:你可能需要将数据存储到文件或数据库中,以便后续分析。
- 实时处理:对于某些应用,你可能需要实时处理数据,这就要求代码的高效性和低延迟。
在实际应用中,你可能需要使用一些库来简化数据处理过程。例如,Boost库提供了丰富的工具来处理数据,而Eigen库则可以帮助你进行矩阵运算和数据分析。
// 使用Eigen库进行数据处理的示例 #include <iostream> #include <Eigen/Dense> int main() { Eigen::MatrixXd m(2, 2); m << 1, 2, 3, 4; Eigen::MatrixXd result = m * m; std::cout << "结果矩阵:\n" << result << std::endl; return 0; }
处理传感器数据时,我们也需要考虑一些潜在的挑战和陷阱:
- 数据噪声:传感器数据可能会受到噪声的影响,你需要使用滤波技术来减少噪声。
- 数据丢失:在实时系统中,数据可能会丢失,你需要设计容错机制来处理这种情况。
- 性能问题:高频率的数据采集和处理可能会对系统性能造成压力,你需要优化代码以确保实时性。
在性能优化方面,你可以考虑以下策略:
- 多线程处理:使用多线程来并行处理数据,可以提高处理效率。
- 缓存机制:使用缓存来减少频繁的硬件访问,提高数据读取速度。
- 算法优化:选择合适的算法来处理数据,可以显著提高性能。
// 使用多线程处理传感器数据的示例 #include <iostream> #include <thread> #include <vector> #include <chrono> void processSensorData(int sensorId) { std::cout << "处理传感器 " << sensorId << " 的数据..." << std::endl; std::this_thread::sleep_for(std::chrono::seconds(1)); std::cout << "传感器 " << sensorId << " 数据处理完成." << std::endl; } int main() { std::vector<std::thread> threads; for (int i = 0; i < 4; ++i) { threads.emplace_back(processSensorData, i); } for (auto& thread : threads) { thread.join(); } return 0; }
总的来说,C++为传感器数据处理提供了强大的工具和灵活性。通过合理使用库、优化代码和考虑潜在的挑战,你可以构建高效、可靠的传感器数据处理系统。在实际项目中,我发现最关键的是理解传感器的特性和数据的需求,然后根据具体情况进行定制化的处理。这不仅能提高代码的性能,还能确保数据的准确性和实时性。
The above is the detailed content of How to process sensor data in C?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










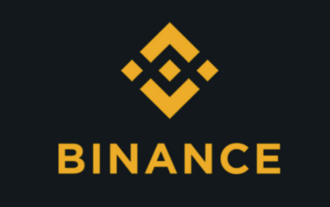
Binance Square is a social media platform provided by Binance Exchange, aiming to provide users with a space to communicate and share information related to cryptocurrencies. This article will explore the functions, reliability and user experience of Binance Plaza in detail to help you better understand this platform.
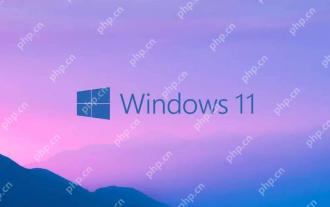
Starting the rollback function on Windows 11 must be performed within 10 days after the upgrade. The steps are as follows: 1. Open "Settings", 2. Enter "System", 3. Find the "Recover" option, 4. Start rollback, 5. Confirm the rollback. After rollback, you need to pay attention to data backup, software compatibility and driver updates.
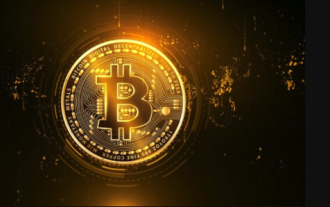
In the field of cryptocurrency trading, the security of exchanges has always been the focus of users. In 2025, after years of development and evolution, some exchanges stand out with their outstanding security measures and user experience. This article will introduce the five most secure exchanges in 2025 and provide practical guides on how to avoid Black U (hacker attacks users) to ensure your funds are 100% secure.
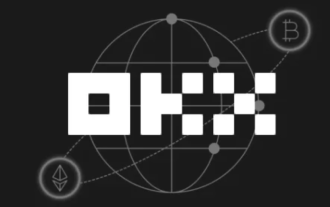
The latest download tutorial for Ouyi OKX6.118.0 version: 1. Click on the quick link in the article; 2. Click on the download (if you are a web user, please register the information first). The latest Android version v6.118.0 optimizes some functions and experiences to make trading easier. Update the app now to experience a more extreme trading experience.
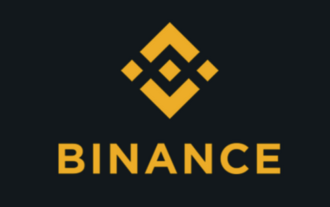
As the world's leading cryptocurrency exchange, Binance is always committed to providing users with a safe and convenient trading experience. Over time, Binance has continuously optimized its platform features and user interface to meet the changing needs of users. In 2025, Binance launched a new login portal aimed at further improving the user experience.
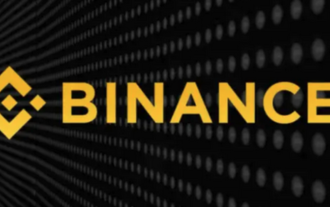
As the world's leading cryptocurrency exchange, Binance is always committed to providing users with a safe and convenient trading experience. Over time, Binance has continuously optimized its platform features and user interface to meet the changing needs of users. In 2025, Binance launched a new login portal aimed at further improving the user experience.
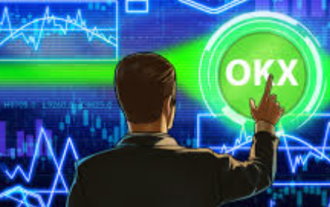
In the cryptocurrency market, choosing a reliable trading platform is crucial. As a world-renowned digital asset exchange, the OK trading platform has attracted a large number of novice users in mainland China. This guide will introduce in detail how to register and use it on the OK trading platform to help novice users get started quickly.
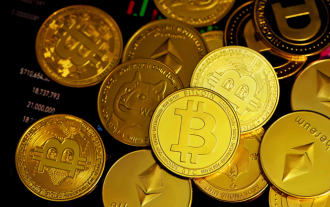
The initial issuance price of XRP is $0.005, set on April 1, 2012, aiming to attract more users and investors to participate in its ecosystem.
