How to implement firmware updates in C?
在C++中实现固件更新可以通过以下步骤:1. 使用稳定的通信协议传输固件文件;2. 实现bootloader接收并写入固件到闪存;3. 确保更新过程的安全性和可靠性,防止设备变砖。
在C++中实现固件更新是一项既有趣又具有挑战性的任务。让我们从回答这个问题开始:如何在C++中实现固件更新?简单来说,固件更新涉及到从设备接收新固件文件,然后将这些文件写入设备的存储器中,最后重启设备以应用更新。接下来,我将详细展开这个过程,分享一些个人的经验和见解。
实现固件更新的核心在于确保更新过程的安全性和可靠性。首先,我们需要一个稳定的通信协议来传输固件文件,比如使用UART、SPI或网络协议。接着,我们需要在设备上实现一个 bootloader,它负责接收新固件并将其写入到闪存中。最后,我们需要确保在更新过程中,设备不会因为断电或其他意外情况而变砖。
让我们从一个简单的例子开始,展示如何在C++中实现一个基本的固件更新机制:
#include <iostream> #include <fstream> #include <vector> class FirmwareUpdater { private: std::vector<uint8_t> firmwareData; std::string firmwareFilePath; public: FirmwareUpdater(const std::string& filePath) : firmwareFilePath(filePath) {} bool loadFirmware() { std::ifstream file(firmwareFilePath, std::ios::binary); if (!file) { std::cerr << "无法打开固件文件: " << firmwareFilePath << std::endl; return false; } file.seekg(0, std::ios::end); size_t fileSize = file.tellg(); file.seekg(0, std::ios::beg); firmwareData.resize(fileSize); file.read(reinterpret_cast<char*>(firmwareData.data()), fileSize); if (file.fail()) { std::cerr << "读取固件文件失败" << std::endl; return false; } return true; } bool updateFirmware() { if (firmwareData.empty()) { std::cerr << "固件数据为空" << std::endl; return false; } // 这里应该有实际的固件写入逻辑 // 例如:写入到闪存或其他存储介质 std::cout << "正在更新固件..." << std::endl; // 模拟写入操作 for (size_t i = 0; i < firmwareData.size(); ++i) { // 实际写入操作 } std::cout << "固件更新完成" << std::endl; return true; } }; int main() { FirmwareUpdater updater("firmware.bin"); if (updater.loadFirmware()) { if (updater.updateFirmware()) { std::cout << "固件更新成功" << std::endl; } else { std::cout << "固件更新失败" << std::endl; } } else { std::cout << "加载固件失败" << std::endl; } return 0; }
这个例子展示了如何加载固件文件并模拟更新过程。实际的固件更新需要考虑更多的细节,比如如何处理中断、如何确保数据完整性,以及如何在更新过程中保持设备的稳定性。
在实际项目中,我曾经遇到过一些挑战,比如如何在更新过程中处理设备的电源管理。一种方法是使用双银行更新机制,即在设备中保留两个固件存储区域,这样在更新过程中可以随时回滚到旧版本。这种方法虽然增加了硬件成本,但大大提高了更新的安全性。
另一个需要注意的点是固件的验证。通常,我们会使用校验和或数字签名来确保接收到的固件是完整且未被篡改的。在C++中,可以使用SHA-256或其他加密算法来实现这一功能。
性能优化也是一个关键点。固件更新通常涉及大量数据传输和写入操作,因此需要优化传输协议和写入算法。例如,可以使用压缩算法来减少传输的数据量,或者使用DMA(直接内存访问)来提高写入速度。
最后,分享一些最佳实践。在编写固件更新代码时,务必确保代码的可读性和可维护性。使用注释和日志记录来帮助调试和维护。同时,考虑到不同设备的兼容性,代码应该尽可能模块化和可移植。
总之,C++中的固件更新是一个复杂但可控的过程。通过仔细设计和实现,我们可以确保设备的固件更新既安全又高效。希望这些经验和见解能帮助你在自己的项目中实现更好的固件更新机制。
The above is the detailed content of How to implement firmware updates in C?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










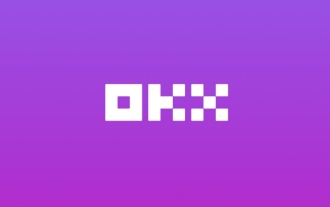
The Ouyi Exchange app supports downloading of Apple mobile phones, visit the official website, click the "Apple Mobile" option, obtain and install it in the App Store, register or log in to conduct cryptocurrency trading.
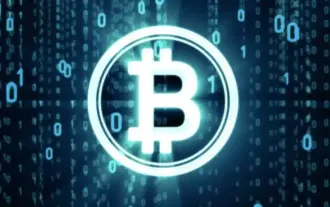
The top ten digital currency exchanges such as Binance, OKX, gate.io have improved their systems, efficient diversified transactions and strict security measures.
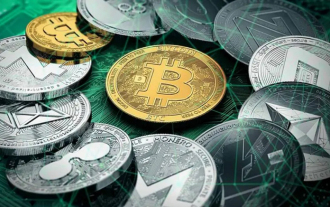
The top ten cryptocurrency trading platforms in the world include Binance, OKX, Gate.io, Coinbase, Kraken, Huobi Global, Bitfinex, Bittrex, KuCoin and Poloniex, all of which provide a variety of trading methods and powerful security measures.
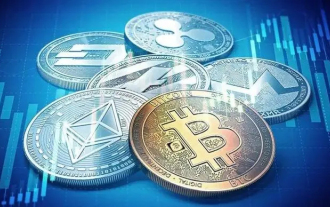
Currently ranked among the top ten virtual currency exchanges: 1. Binance, 2. OKX, 3. Gate.io, 4. Coin library, 5. Siren, 6. Huobi Global Station, 7. Bybit, 8. Kucoin, 9. Bitcoin, 10. bit stamp.
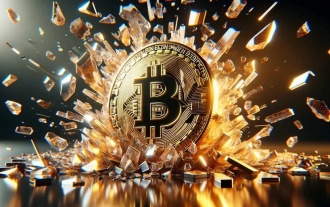
Bitcoin’s price ranges from $20,000 to $30,000. 1. Bitcoin’s price has fluctuated dramatically since 2009, reaching nearly $20,000 in 2017 and nearly $60,000 in 2021. 2. Prices are affected by factors such as market demand, supply, and macroeconomic environment. 3. Get real-time prices through exchanges, mobile apps and websites. 4. Bitcoin price is highly volatile, driven by market sentiment and external factors. 5. It has a certain relationship with traditional financial markets and is affected by global stock markets, the strength of the US dollar, etc. 6. The long-term trend is bullish, but risks need to be assessed with caution.
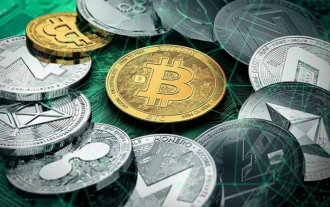
The top ten cryptocurrency exchanges in the world in 2025 include Binance, OKX, Gate.io, Coinbase, Kraken, Huobi, Bitfinex, KuCoin, Bittrex and Poloniex, all of which are known for their high trading volume and security.
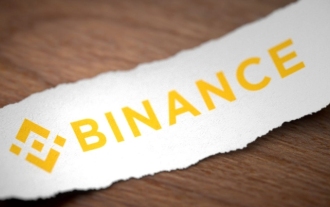
Visit Binance official website and check HTTPS and green lock logos to avoid phishing websites, and official applications can also be accessed safely.
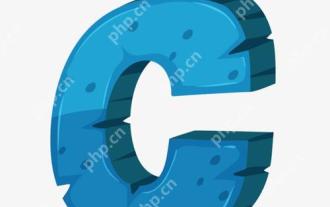
ABI compatibility in C refers to whether binary code generated by different compilers or versions can be compatible without recompilation. 1. Function calling conventions, 2. Name modification, 3. Virtual function table layout, 4. Structure and class layout are the main aspects involved.
