How to implement polymorphism in C?
C++中实现多态可以通过虚函数和继承实现。1.定义虚函数和纯虚函数,允许派生类重写或必须实现。2.使用虚析构函数确保正确释放资源。3.使用override关键字明确重写函数。需要注意性能开销和对象切片问题。
在C++中实现多态就像在编程世界中绘制一幅多彩的画卷,它让我们的代码变得灵活而充满活力。让我们从问题的核心出发,深入探讨如何在C++中实现这种强大的特性。
C++中的多态性,简而言之,就是允许不同对象对同一个消息做出不同的反应。这就像在现实生活中,不同的人对同一个问题可能有不同的回答。实现多态的关键在于使用虚函数(virtual functions)和继承(inheritance)。虚函数允许派生类重写基类的函数,从而实现不同的行为。
让我们先看一个简单的例子,感受一下多态的魅力:
#include <iostream> using namespace std; class Shape { public: virtual void draw() { cout << "Drawing a shape" << endl; } virtual ~Shape() {} // 虚析构函数,确保正确释放资源 }; class Circle : public Shape { public: void draw() override { cout << "Drawing a circle" << endl; } }; class Rectangle : public Shape { public: void draw() override { cout << "Drawing a rectangle" << endl; } }; int main() { Shape* shape1 = new Circle(); Shape* shape2 = new Rectangle(); shape1->draw(); // 输出: Drawing a circle shape2->draw(); // 输出: Drawing a rectangle delete shape1; delete shape2; return 0; }
这个例子展示了基本的多态实现。Shape
类定义了一个虚函数draw
,而Circle
和Rectangle
类通过重写这个函数来实现不同的绘制行为。通过指针调用draw
函数时,实际调用的是具体对象的实现,这就是多态的本质。
在实现多态时,有几个关键点需要注意:
-
虚函数和纯虚函数:虚函数允许派生类重写,而纯虚函数(
virtual void func() = 0;
)则要求派生类必须实现它。纯虚函数可以用于定义抽象基类(Abstract Base Class, ABC),这在设计模式中非常常见。 - 虚析构函数:在基类中定义虚析构函数可以确保当通过基类指针删除派生类对象时,派生类的析构函数会被正确调用,避免内存泄漏。
-
override关键字:使用
override
关键字可以明确表示派生类函数是在重写基类函数,这有助于编译器检查和避免错误。
然而,多态也有一些需要注意的陷阱和优化点:
- 性能开销:多态的实现依赖于虚函数表(vtable),这会带来一些额外的内存和运行时开销。虽然在大多数情况下这种开销可以忽略不计,但在性能敏感的应用中需要谨慎使用。
- 对象切片:当通过值传递派生类对象时,会发生对象切片(slicing),导致多态失效。尽量使用指针或引用传递对象。
- 动态绑定:多态的动态绑定特性虽然灵活,但在某些情况下可能会影响代码的可读性和维护性。需要在灵活性和可维护性之间找到平衡。
在实际应用中,多态可以帮助我们设计出更灵活、可扩展的系统。例如,在游戏开发中,不同的敌人类型可以继承自一个基类Enemy
,每个派生类实现自己的attack
方法,这样可以轻松地添加新敌人类型而不需要修改现有代码。
总之,C++中的多态是一把双刃剑,它为我们提供了强大的抽象和扩展能力,但也需要我们谨慎使用,避免潜在的陷阱。通过理解和掌握多态的实现和应用,我们可以编写出更高效、更易维护的代码。
The above is the detailed content of How to implement polymorphism in C?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










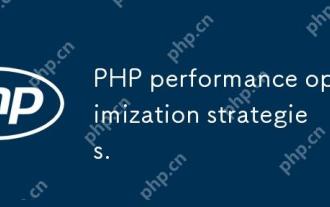
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
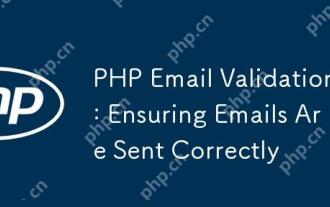
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl

APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
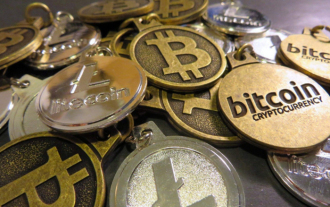
Top 10 virtual currency exchange apps in the currency circle: 1. Binance, 2. OKX, 3. Huobi, 4. Coinbase, 5. Kraken, 6. Bitfinex, 7. Bybit, 8. KuCoin, 9. Gemini, 10. Bitstamp, these platforms are popular for their transaction volume, security and user experience.
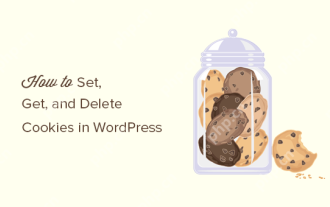
Do you want to know how to use cookies on your WordPress website? Cookies are useful tools for storing temporary information in users’ browsers. You can use this information to enhance the user experience through personalization and behavioral targeting. In this ultimate guide, we will show you how to set, get, and delete WordPresscookies like a professional. Note: This is an advanced tutorial. It requires you to be proficient in HTML, CSS, WordPress websites and PHP. What are cookies? Cookies are created and stored when users visit websites.
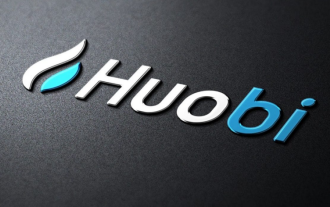
Huobi APKV10.50.0 download guide: 1. Click the direct link in the article; 2. Select the correct download package; 3. Fill in the registration information; 4. Start the Huobi trading process.

Huobi APKV10.50.0 download guide: 1. Click the direct link in the article; 2. Select the correct download package; 3. Fill in the registration information; 4. Start the Huobi trading process.
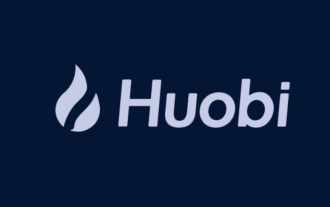
Huobi APKV10.50.0 download guide: 1. Click the direct link in the article; 2. Select the correct download package; 3. Fill in the registration information; 4. Start the Huobi trading process.
