The Execution Order of init Functions in Go Packages
Go initializes packages in the order they are imported, then executes init functions within a package in their definition order, and file names determine the order across multiple files. This process can be influenced by dependencies between packages, which may lead to complex initialization sequences and potential issues like circular dependencies or side effects.
When diving into the world of Go programming, understanding the execution order of init
functions within packages is crucial. This isn't just about knowing the sequence; it's about grasping how Go's initialization process can affect your application's behavior and performance. Let's explore this topic in depth, sharing insights and experiences along the way.
In Go, init
functions are special because they run automatically before the main
function. But what happens when you have multiple init
functions across different packages? The order in which these functions execute can be a bit tricky, and it's something that caught me off guard when I first started working with Go.
The key to understanding this lies in Go's package initialization rules. When Go starts up, it initializes packages in a specific order, which can be influenced by import statements and dependencies between packages. Here's how it works:
- Go initializes packages in the order they are imported. If package A imports package B, B will be initialized before A.
- Within a package,
init
functions are executed in the order they are defined in the source file. - If there are multiple source files in a package, the order of initialization is determined by the file names in lexicographical order.
Let's look at a practical example to illustrate this:
// File: main.go package main import ( "fmt" "example/packageA" "example/packageB" ) func main() { fmt.Println("Main function") }
// File: packageA/a.go package packageA import "fmt" func init() { fmt.Println("Init A1") } func init() { fmt.Println("Init A2") }
// File: packageB/b.go package packageB import "fmt" func init() { fmt.Println("Init B1") }
When you run this program, the output will be:
Init B1 Init A1 Init A2 Main function
This order makes sense because packageB
is initialized before packageA
due to the import order in main.go
. Within packageA
, the init
functions are executed in the order they appear in the file.
Now, let's dive deeper into some nuances and potential pitfalls:
- Circular Dependencies: If package A imports package B, and package B imports package A, Go will detect this and throw an error. This is a good thing because it prevents infinite loops during initialization.
- Initialization Order and Side Effects: Be cautious with
init
functions that have side effects, like opening files or establishing network connections. If these operations fail, your program might crash before it even reachesmain
. - Testing and Debugging: When writing tests, remember that
init
functions will run before your test code. This can sometimes lead to unexpected behavior if you're not careful.
Here's a more complex example to show how dependencies can affect initialization order:
// File: main.go package main import ( "fmt" "example/packageA" "example/packageB" ) func main() { fmt.Println("Main function") }
// File: packageA/a.go package packageA import ( "fmt" "example/packageB" ) func init() { fmt.Println("Init A1") packageB.SomeFunction() } func init() { fmt.Println("Init A2") }
// File: packageB/b.go package packageB import "fmt" func init() { fmt.Println("Init B1") } func SomeFunction() { fmt.Println("SomeFunction in B") }
In this case, the output will be:
Init B1 Init A1 SomeFunction in B Init A2 Main function
Notice how SomeFunction
in packageB
is called during the initialization of packageA
. This demonstrates how dependencies can influence the order of execution.
When it comes to optimizing and managing init
functions, here are some tips based on my experience:
-
Minimize Side Effects: Try to keep
init
functions as simple as possible. Avoid operations that could fail or have significant side effects. -
Use
init
for Initialization Only:init
functions should be used for initialization tasks, not for running your application's core logic. -
Be Aware of Dependencies: Always consider the dependencies between packages when writing
init
functions. A change in one package'sinit
function could affect the behavior of another package.
In conclusion, understanding the execution order of init
functions in Go is essential for writing robust and predictable code. By keeping these principles in mind and being mindful of the potential pitfalls, you can harness the power of Go's initialization process to build more efficient and reliable applications.
The above is the detailed content of The Execution Order of init Functions in Go Packages. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










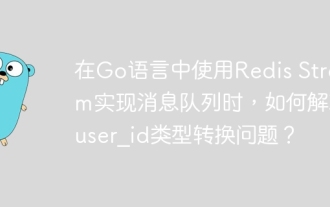
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
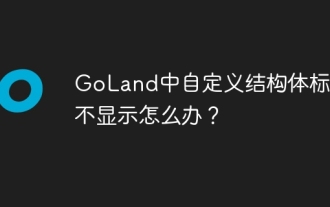
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
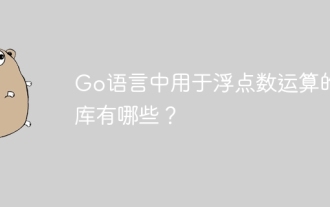
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
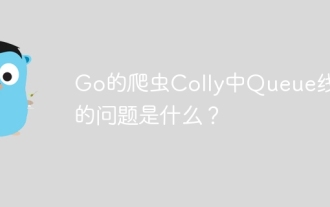
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
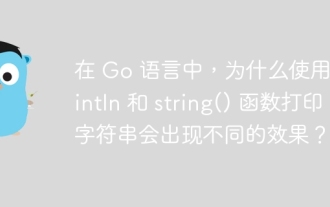
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
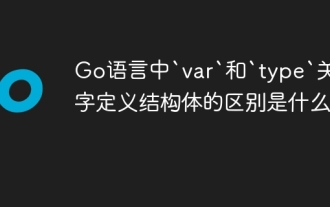
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
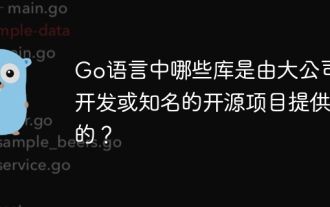
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
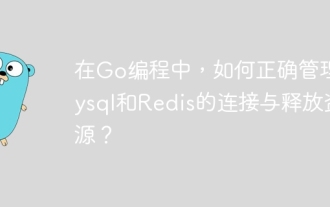
Resource management in Go programming: Mysql and Redis connect and release in learning how to correctly manage resources, especially with databases and caches...
