


Python vs. C : A Comparative Analysis of Programming Languages
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
introduction
Do you want to know which is better for your project, Python or C? This article will explore in-depth features, advantages and disadvantages, and applicable scenarios of two popular programming languages, Python and C. By reading this article, you will learn about the grammatical differences, performance, development efficiency and applications in different fields, so as to better choose the programming language that suits you.
Review of basic knowledge
Python is an interpretative, object-oriented programming language known for its concise syntax and a powerful library ecosystem. It is widely used in data science, machine learning, artificial intelligence and other fields. C is a compiled language that emphasizes performance and underlying controls, and is often used in system programming, game development and high-performance computing.
Python's ease of use and rapid development capabilities make it the first choice for beginners, while C has attracted many professional developers due to its powerful control and performance advantages. The learning curves and development experiences of the two are very different, which is also the focus we need to discuss.
Core concept or function analysis
Syntax and ease of use
Python's syntax is concise and clear, emphasizing readability. For example, Python's indentation mechanism makes the code structure clear:
def greet(name): if name: print(f"Hello, {name}!") else: print("Hello, stranger!")
In contrast, C's syntax is more complex and requires manual management of memory and pointers, which can be a challenge for beginners:
#include <iostream> using namespace std; void greet(string name) { if (!name.empty()) { cout << "Hello, " << name << "!" << endl; } else { cout << "Hello, stranger!" << endl; } }
Python's ease of use makes development faster, but may also sacrifice performance in some cases. Although C is complicated in syntax, it provides more control and optimization space.
Performance and efficiency
C is known for its high performance because it is a compiled language that can directly generate machine code. Here is a simple C loop performance example:
#include <iostream> using namespace std; int main() { int sum = 0; for (int i = 0; i < 10000000; i) { sum = i; } cout << "Sum: " << sum << endl; return 0; }
Python, as an interpreted language, runs relatively slowly, but can be optimized by using tools such as Cython or Numba. Here is a similar Python example:
sum = 0 for i in range(100000000): sum = i print(f"Sum: {sum}")
In performance-sensitive applications, C is usually a better choice, but Python's development efficiency and rich library ecosystem are more important in some scenarios.
Example of usage
Data processing and scientific computing
Python has performed well in the fields of data processing and scientific computing, mainly thanks to the support of libraries such as NumPy and Pandas. Here is an example of using Pandas to process data:
import pandas as pd # Read CSV file data = pd.read_csv('data.csv') # Calculate the average value average = data['column_name'].mean() print(f"Average: {average}")
In C, although data processing can be performed, more code needs to be written manually and there is a lack of rich library support like Python.
Game development
C takes the lead in game development because it provides high performance and low latency features. Here is a simple C game loop example:
#include <iostream> using namespace std; class Game { public: void run() { while (true) { update(); render(); } } private: void update() { // Update game status} void render() { // Render the game screen} }; int main() { Game game; game.run(); return 0; }
Although Python can also be used for game development, it usually requires libraries such as Pygame and may not be as good as C in performance.
Common Errors and Debugging Tips
In Python, common errors include indentation errors and type errors. Here is an example of an indentation error:
def example(): print("This will raise an IndentationError")
In C, common errors include memory leaks and pointer errors. Here is an example of a memory leak:
#include <iostream> using namespace std; int main() { int* ptr = new int(10); // Forgot to delete ptr, resulting in memory leak return 0; }
When debugging Python code, you can use the pdb module, while C can use debugging tools such as GDB or Visual Studio.
Performance optimization and best practices
Performance optimization
In Python, you can optimize your code by using list comprehensions, generator expressions, and built-in functions. For example, list comprehensions can significantly improve the execution efficiency of your code:
# Traditional loop squares = [] for i in range(10): squares.append(i**2) # List comprehension squares = [i**2 for i in range(10)]
In C, performance can be improved by using STL containers, algorithms, and optimized compilation options. For example, using std::vector
and std::algorithm
can improve code efficiency:
#include <vector> #include <algorithm> using namespace std; int main() { vector<int> numbers = {1, 2, 3, 4, 5}; // Use std::transform to calculate square transform(numbers.begin(), numbers.end(), numbers.begin(), [](int x) { return x * x; }); return 0; }
Best Practices
In Python, following the PEP 8 style guide can improve the readability and maintainability of your code. For example, function and variable naming should use lowercase letters and underscores:
def calculate_average(numbers): return sum(numbers) / len(numbers)
In C, following Google C Style Guide or C Core Guidelines can help write high-quality code. For example, avoid using raw pointers and try to use smart pointers:
#include <memory> using namespace std; class MyClass { public: void doSomething() { // Use the smart pointer unique_ptr<int> ptr = make_unique<int>(10); } };
in conclusion
Python and C have their own advantages and disadvantages, and which language to choose depends on your project needs and personal preferences. Python excels in data science and rapid prototyping with its ease of use and rich library ecosystem, while C gains an advantage in system programming and game development with its high performance and underlying control capabilities. Hopefully this article will help you better understand the characteristics of these two languages and make informed choices.
The above is the detailed content of Python vs. C : A Comparative Analysis of Programming Languages. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










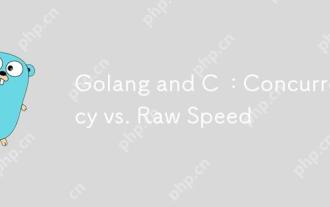
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
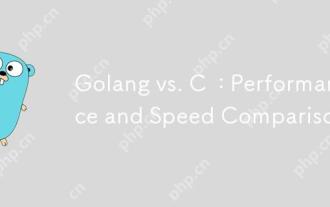
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
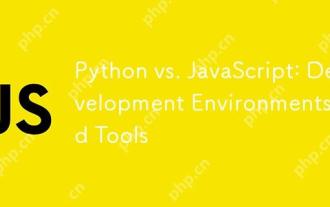
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
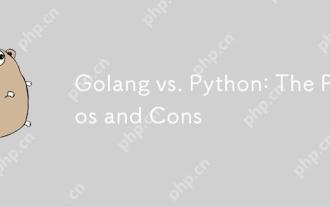
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
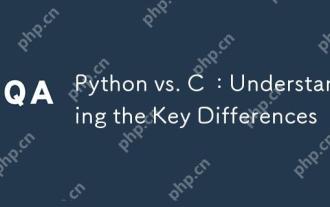
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
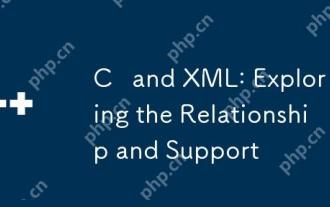
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.

Laravel is suitable for projects that teams are familiar with PHP and require rich features, while Python frameworks depend on project requirements. 1.Laravel provides elegant syntax and rich features, suitable for projects that require rapid development and flexibility. 2. Django is suitable for complex applications because of its "battery inclusion" concept. 3.Flask is suitable for fast prototypes and small projects, providing great flexibility.
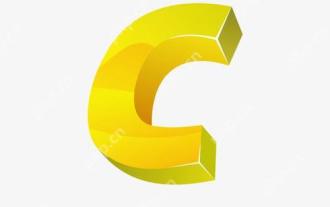
The application of static analysis in C mainly includes discovering memory management problems, checking code logic errors, and improving code security. 1) Static analysis can identify problems such as memory leaks, double releases, and uninitialized pointers. 2) It can detect unused variables, dead code and logical contradictions. 3) Static analysis tools such as Coverity can detect buffer overflow, integer overflow and unsafe API calls to improve code security.
