Golang: Concurrency and Performance in Action
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by go run-race; 5. Performance optimization suggests reducing channel usage, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
introduction
In modern software development, efficient utilization of system resources is crucial, and concurrent programming is the key to achieving this goal. Golang, with its concise and powerful concurrency model, has become the preferred language for many developers. This article will take you to gain an in-depth understanding of Golang's concurrency characteristics and explore its practical application in performance optimization. After reading this article, you will master the core concepts of Golang concurrency and learn how to use these technologies to improve the performance of your program in real projects.
Review of basic knowledge
Golang's concurrency model is based on CSP (Communicating Sequential Processes) theory and is implemented through goroutine and channel. goroutine is a lightweight thread that can be easily started with the go
keyword. Channel is a pipeline used for communication between goroutines to ensure the secure transmission of data.
To understand the concurrency characteristics of Golang, you need to first understand the basic concurrency concepts and Golang's runtime. Golang's runtime is responsible for managing goroutine scheduling and resource allocation to ensure efficient concurrent execution.
Core concept or function analysis
Definition and function of goroutine and channel
goroutine is the basic unit of concurrent execution in Golang. It is very simple to start, just add the go
keyword before the function. For example:
func main() { go saysHello() } func saysHello() { fmt.Println("Hello, goroutine!") }
Channel is used for data transmission and synchronization between goroutines. The syntax of defining a channel is as follows:
ch := make(chan int)
Use channel to implement secure communication between goroutines and avoid race conditions.
How it works
Golang's concurrency model manages goroutines through a scheduler in runtime. The scheduler will decide when to switch the execution of the goroutine based on the system resources and the status of the goroutine. This scheduling mechanism makes Golang very efficient in concurrency because it can support concurrent execution of thousands of goroutines without increasing the system burden.
The working principle of a channel is based on a memory queue. When a goroutine sends data to the channel, the data will be put into the queue, waiting for another goroutine to be read from the channel. The blocking feature of channel ensures synchronous data transmission and avoids data competition.
Example of usage
Basic usage
Let's look at a simple concurrency example showing how to use goroutine and channel:
package main import ( "fmt" "time" ) func worker(id int, jobs <-chan int, results chan<- int) { for j := range jobs { fmt.Println("worker", id, "started job", j) time.Sleep(time.Second) fmt.Println("worker", id, "finished job", j) results <- j * 2 } } func main() { jobs := make(chan int, 100) results := make(chan int, 100) for w := 1; w <= 3; w { go worker(w, jobs, results) } for j := 1; j <= 5; j { jobs <- j } close(jobs) for a := 1; a <= 5; a { <-results } }
In this example, we create 3 worker goroutines that receive tasks through the channel and process them, and then send the result back to the main goroutine through another channel.
Advanced Usage
In more complex scenarios, we can use select
statements to handle operations of multiple channels. For example:
package main import ( "fmt" "time" ) func main() { c1 := make(chan string) c2 := make(chan string) go func() { time.Sleep(1 * time.Second) c1 <- "one" }() go func() { time.Sleep(2 * time.Second) c2 <- "two" }() for i := 0; i < 2; i { select { case msg1 := <-c1: fmt.Println("received", msg1) case msg2 := <-c2: fmt.Println("received", msg2) } } }
select
statement allows us to listen to multiple channels at the same time. When any channel has data ready, the corresponding case will be executed.
Common Errors and Debugging Tips
Common errors when using concurrent programming include deadlocks and data races. Deadlocks usually occur when two goroutines are waiting for each other to release resources, while data competition occurs when multiple goroutines access shared data at the same time.
Use the go run -race
command to help detect data races, for example:
package main import ( "fmt" "time" ) func main() { var data int go func() { data }() time.Sleep(1 * time.Second) fmt.Println(data) }
Run go run -race main.go
will detect data races and give detailed reports.
Performance optimization and best practices
In practical applications, Golang's concurrency model can significantly improve the performance of the program, but some optimization techniques and best practices need to be paid attention to.
Performance optimization
Reduce the use of channels : Although channel is the core of Golang concurrent programming, overuse will increase memory overhead. In scenarios where frequent communication is not required, shared memory or other synchronization mechanisms may be considered.
Set the number of goroutines reasonably : Too many goroutines will increase scheduling overhead and affect performance. The number of goroutines can be set reasonably according to actual needs, and the number of CPUs that are executed concurrently can be controlled through
runtime.GOMAXPROCS
.Use sync.Pool : In high concurrency scenarios, frequent memory allocation and release will affect performance. Using
sync.Pool
can reduce the pressure of garbage collection and improve the efficiency of the program.
For example, use sync.Pool
to manage temporary objects:
package main import ( "fmt" "sync" ) type MyStruct struct { Value int } var pool = sync.Pool{ New: func() interface{} { return &MyStruct{} }, } func main() { obj := pool.Get().(*MyStruct) obj.Value = 42 fmt.Println(obj.Value) pool.Put(obj) }
Best Practices
Code readability : Concurrent code is often more difficult to understand than sequential code, so it is very important to keep the code readability. Use clear naming and appropriate annotations to help other developers understand and maintain the code.
Error handling : In concurrent programming, error handling becomes more complicated. Use
recover
andpanic
to catch and handle exceptions in goroutines to avoid program crashes.Testing and debugging : Special attention is required for testing and debugging of concurrent programs. Use
go test -race
to detect data race, whilego test -cpu
can simulate performance under different CPU counts.
Through the above strategies and techniques, you can better utilize concurrency features in Golang and improve the performance and reliability of your program. Hopefully this article can provide valuable guidance and inspiration for you to use Golang concurrent programming in your actual project.
The above is the detailed content of Golang: Concurrency and Performance in Action. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
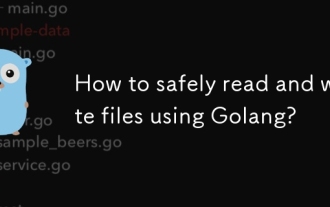
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
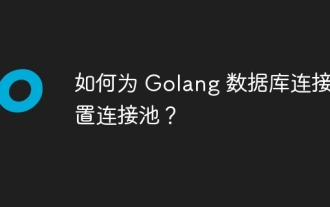
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
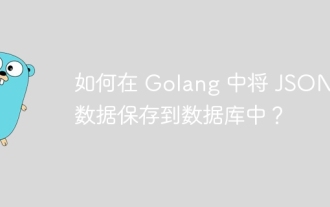
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
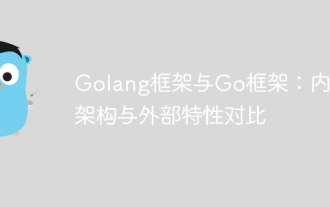
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
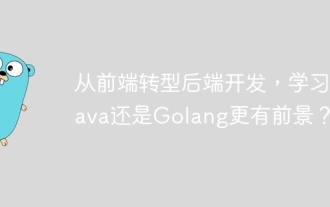
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
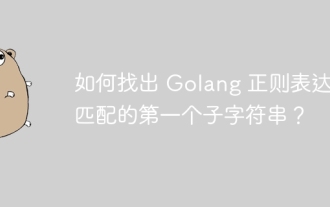
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
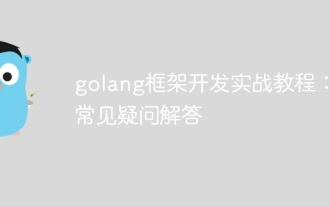
Go framework development FAQ: Framework selection: Depends on application requirements and developer preferences, such as Gin (API), Echo (extensible), Beego (ORM), Iris (performance). Installation and use: Use the gomod command to install, import the framework and use it. Database interaction: Use ORM libraries, such as gorm, to establish database connections and operations. Authentication and authorization: Use session management and authentication middleware such as gin-contrib/sessions. Practical case: Use the Gin framework to build a simple blog API that provides POST, GET and other functions.
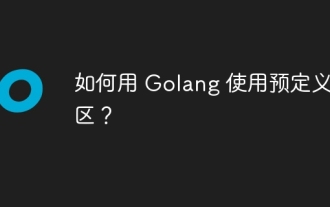
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
