Demonstrating Reusable React Components in a Form
The cornerstone of React applications are components. It is almost impossible to build a React application without using components. The widespread use of components has even led to some third-party packages providing components that can be used for integration capabilities.
These third-party components are usually reusable . The difference between them and components that may already be available in your application is specificity .
I mean: Suppose you run a company that sells Polo shirts, you can do this:
- Produce Polo shirts that have been printed with patterns, or
- Let the buyers choose the pattern they want.
Some basic elements will remain consistent, for example, all Polo shirts should be short-sleeved. But users can choose different styles of shirts, such as colors and sizes. In this case, a short-sleeved Polo shirt is a good React component: it is the same item with different variations.
Now let's say you are developing a login form. Like Polo shirts, forms have consistent characteristics, but we are not focusing on size and color changes, but input fields, submit buttons, and maybe even forget password links. This can componentize and implement various changes in inputs, buttons, links, etc.
Example of input elements
Let's look at it from the perspective of creating input fields for the form. Typical text inputs in the React component look like this:
class Form extends React.Component { constructor(props) { super(props); this.state = { username: '' }; } handleChange = (event) => { this.setState({ username: event.target.value }); }; render() { Return (
In order for this input element to be reusable elsewhere and in the project, we have to extract it into its own component. Let's name it FormInput.
import React from 'react'; import PropTypes from 'prop-types'; const FormInput = ({ name, type, placeholder, onChange, className, value, error, children, label, ...props }) => { Return ( <div> <label htmlfor="{name}">{label}</label> <input type="{type}" name="{name}" placeholder="{placeholder}" onchange="{onChange}" classname="{className}" value="{value}"> {error &&<p> {error}</p> } </div> ); }; FormInput.defaultProps = { type: "text", className: "" }; FormInput.propTypes = { name: PropTypes.string.isRequired, type: PropTypes.oneOf(['text', 'number', 'password']), placeholder: PropTypes.string.isRequired, className: PropTypes.string, value: PropTypes.any, onChange: PropTypes.func.isRequired, label: PropTypes.string.isRequired // Added required label prop }; export default FormInput;
This component accepts certain props, such as the properties we need to create input using valid tags, including placeholders, values, and names. We set the input element in the render function and set the property value to props passed to the component. We even bind the input to the tag to make sure they are always together. You can see that we are not making assumptions by predefined anything. Our goal is to make sure that the component can be used in as many scenarios as possible.
This makes up a good component because it enforces good markup (what Brad Frost calls it "dumb React"), which suggests that not every component must be some kind of highly complex functionality. That being said, if we are talking about something very basic, like static headers, then using React components might be a bit overkill. The possible criterion for making something as a reusable component is when you need to use the same functionality in other parts of your application. If the component is used only once, a "reusable" component is usually not required.
We can use our input component in another component LoginPage.
import React, { Component } from 'react'; import FormInput from './FormInput'; // Import FormInput component class LoginPage extends Component { state = { user: { username: "", password: "" }, errors: {}, submitted: false }; handleChange = event => { const { user } = this.state; user[event.target.name] = event.target.value; this.setState({ user }); }; onSubmit = (event) => { event.preventDefault(); // Prevent default form submission const { user } = this.state; let errors = {}; if (!user.username) { errors.username = "Enter your username!"; } if (user.password.length {submitted ? ( <p>Welcome onboard, {user.username}!</p> ) : ( <div> <h3 id="Login">Login!</h3> <forminput name="username" label="Username" placeholder="Enter your username" value="{user.username}" onchange="{this.handleChange}" error="{errors.username}"></forminput> <forminput name="password" type="password" label="Password" placeholder="Enter your password" value="{user.password}" onchange="{this.handleChange}" error="{errors.password}"></forminput> <button type="submit">Login</button> </div> )} ); } } export default LoginPage;
Have you seen how LoginPage uses FormInput twice? We use it as text input for both username and password. If we want to change the functionality of the input, we can make these changes in the FormInput component file we created, and these changes will be applied to each instance of using the input component. This is the basic advantage of having reusable components: you don't have to repeat yourself .
Even the error is displayed from the FormInput component.
The onSubmit function first verifies the user object we get from the form to make sure it conforms to the structure in which the username has a value. Note that we can even extend the functionality of the input, as we did, checking if the password contains at least eight characters.
If you look at the code, you will see that there is a Button component in it. This is different from an HTML element, but rather a different component that takes props that define the type of button we want (submit, reset, button), its class name, what to do when clicked, and the label. We can integrate many other button properties to enforce any standard you want.
const Button = ({ type, className, onClick, label, ...props }) => ( <button type="{type}" classname="{className}" onclick="{onClick}"> {label} </button> ); export default Button;
This is the final login form after putting all the components together.
Want to try it yourself? Try using reusable<input>
element. If this is too difficult, you can<input>
The element starts, then maybe a checkbox, and then jumps to<select></select>
. The key idea is to make it universal. I would love to see your achievements, so please link your work in the comment section!
The above is the detailed content of Demonstrating Reusable React Components in a Form. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










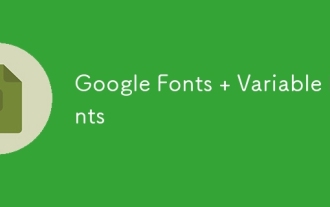
I see Google Fonts rolled out a new design (Tweet). Compared to the last big redesign, this feels much more iterative. I can barely tell the difference
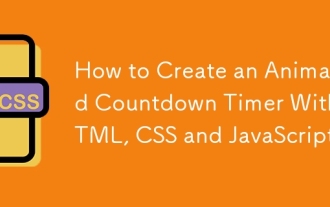
Have you ever needed a countdown timer on a project? For something like that, it might be natural to reach for a plugin, but it’s actually a lot more
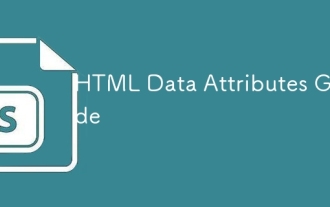
Everything you ever wanted to know about data attributes in HTML, CSS, and JavaScript.
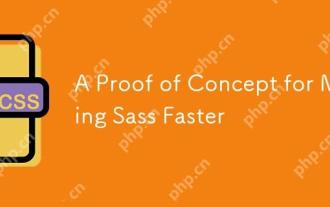
At the start of a new project, Sass compilation happens in the blink of an eye. This feels great, especially when it’s paired with Browsersync, which reloads
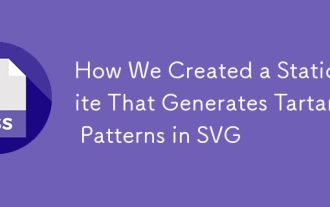
Tartan is a patterned cloth that’s typically associated with Scotland, particularly their fashionable kilts. On tartanify.com, we gathered over 5,000 tartan
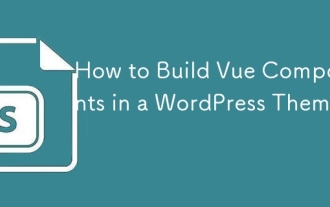
The inline-template directive allows us to build rich Vue components as a progressive enhancement over existing WordPress markup.
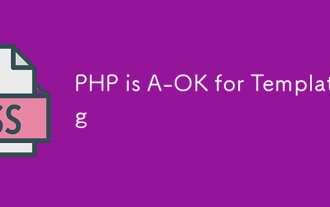
PHP templating often gets a bad rap for facilitating subpar code — but that doesn't have to be the case. Let’s look at how PHP projects can enforce a basic
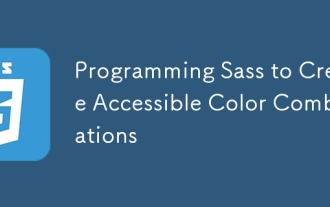
We are always looking to make the web more accessible. Color contrast is just math, so Sass can help cover edge cases that designers might have missed.
