How to efficiently convert strings into objects in Vue.js?
Efficiently convert strings to objects in Vue.js. If the string is standard JSON, use JSON.parse(); if the string format is not standardized, it is recommended to use regular expressions to match key-value pairs and parse them according to the value type, but attention should be paid to the complexity of regular expressions and code maintainability. Additionally, for enhanced robustness, an error handling mechanism should be added to deal with invalid strings.
Efficiently convert strings into objects in Vue.js: Don't be blinded by JSON.parse()
When many Vue.js developers encounter the need to convert strings into objects, their first reaction is JSON.parse()
. That's right, it's easy to use, but it's not a panacea. This article will give you an in-depth discussion and tell you when to use JSON.parse()
, when to need more elegant and efficient solutions, and some pitfalls you may have stepped on.
Let's talk about the conclusion first: JSON.parse()
is only applicable to standard JSON strings. If you get a string with a less standard format or a string containing special characters or escape characters, it will give you a headache. Even a simple error can crash your application.
Let's review the basics first. JSON (JavaScript Object Notation) is a lightweight data exchange format that requires strict structure: key-value pairs are enclosed in double quotes, and the values must be numeric, string, boolean, array, or null. Any string that deviates from this specification, JSON.parse()
will ruthlessly throw an error.
Imagine that the string you receive from the backend looks like this: "{name: 'John Doe', age: 30}"
. Note that the key names are not enclosed in double quotes! JSON.parse()
will directly report an error. At this time, you need a more flexible solution.
A more robust solution is to use regular expressions, combined with the eval()
function. I know that many veteran drivers avoid eval()
because it poses security risks. But if your string source is controllable and you are familiar enough with regular expressions, it can help you solve many problems.
<code class="javascript">function stringToObject(str) { // 简单的正则表达式,将键值对提取出来const pairs = str.match(/(\w ):\s*('([^']*)'|(\d )|true|false|null)/g); const obj = {}; if(pairs){ pairs.forEach(pair => { const [key, value] = pair.split(':').map(s => s.trim()); // 处理各种数据类型obj[key] = isNaN(value) ? (value.startsWith("'") ? value.slice(1,-1) : (value === 'true' ? true : (value === 'false' ? false : (value === 'null' ? null : value)))) : parseFloat(value); }); } return obj; } let myString = "{name: 'John Doe', age: 30, isMarried: true, address: null}"; let myObject = stringToObject(myString); console.log(myObject); // Output: { name: 'John Doe', age: 30, isMarried: true, address: null } myString = "{name: 'John Doe', age: 30, skills: ['js', 'vue']}"; //处理数组,需要更复杂的正则表达式myObject = stringToObject(myString); //这部分正则表达式需要改进才能正确处理数组console.log(myObject); //Output: {name: 'John Doe', age: 30, skills: '[\'js\', \'vue\']'} //数组没有被正确解析let invalidString = "{name: 'John Doe', age: 30, skills: ['js', 'vue']} "; //末尾多余空格myObject = stringToObject(invalidString); console.log(myObject); //Output: {name: 'John Doe', age: 30, skills: '[\'js\', \'vue\']'} //数组没有被正确解析</code>
This code uses regular expressions to extract key-value pairs and then parse them according to the type of value. But this is just a simple example, if your string format is more complex, such as containing nested objects or arrays, then you need a more powerful regex to handle. This requires you to have a deep understanding of regular expressions. Remember, complex regular expressions can be difficult to maintain and understand, so weigh the pros and cons.
Additionally, to enhance the robustness of your code, you should add error handling mechanisms, such as checking if the string is empty or if the format is valid. Never let an invalid string cause your application to crash.
Finally, remember, there is no perfect solution. Which option you choose depends on your specific needs and the format of the string. If your string is standard JSON, then JSON.parse()
is the easiest and most efficient way to do it. But if your string format is not standardized, then you need more flexible solutions, such as using regular expressions and eval()
(use with caution). Weigh security and efficiency and choose the one that suits you best. Remember, the readability and maintainability of the code are just as important. Don't write incomprehensible code in order to pursue the so-called "efficiency".
The above is the detailed content of How to efficiently convert strings into objects in Vue.js?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










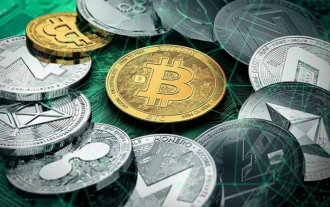
The top ten cryptocurrency exchanges in the world in 2025 include Binance, OKX, Gate.io, Coinbase, Kraken, Huobi, Bitfinex, KuCoin, Bittrex and Poloniex, all of which are known for their high trading volume and security.
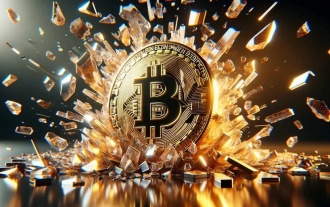
Bitcoin’s price ranges from $20,000 to $30,000. 1. Bitcoin’s price has fluctuated dramatically since 2009, reaching nearly $20,000 in 2017 and nearly $60,000 in 2021. 2. Prices are affected by factors such as market demand, supply, and macroeconomic environment. 3. Get real-time prices through exchanges, mobile apps and websites. 4. Bitcoin price is highly volatile, driven by market sentiment and external factors. 5. It has a certain relationship with traditional financial markets and is affected by global stock markets, the strength of the US dollar, etc. 6. The long-term trend is bullish, but risks need to be assessed with caution.
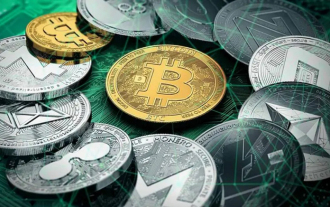
The top ten cryptocurrency trading platforms in the world include Binance, OKX, Gate.io, Coinbase, Kraken, Huobi Global, Bitfinex, Bittrex, KuCoin and Poloniex, all of which provide a variety of trading methods and powerful security measures.
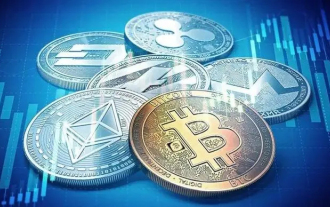
Currently ranked among the top ten virtual currency exchanges: 1. Binance, 2. OKX, 3. Gate.io, 4. Coin library, 5. Siren, 6. Huobi Global Station, 7. Bybit, 8. Kucoin, 9. Bitcoin, 10. bit stamp.
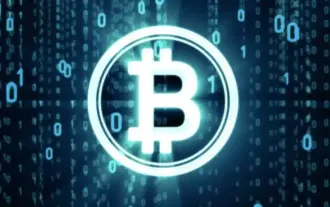
The top ten digital currency exchanges such as Binance, OKX, gate.io have improved their systems, efficient diversified transactions and strict security measures.
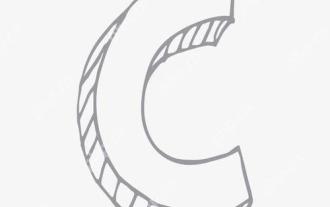
Using the chrono library in C can allow you to control time and time intervals more accurately. Let's explore the charm of this library. C's chrono library is part of the standard library, which provides a modern way to deal with time and time intervals. For programmers who have suffered from time.h and ctime, chrono is undoubtedly a boon. It not only improves the readability and maintainability of the code, but also provides higher accuracy and flexibility. Let's start with the basics. The chrono library mainly includes the following key components: std::chrono::system_clock: represents the system clock, used to obtain the current time. std::chron
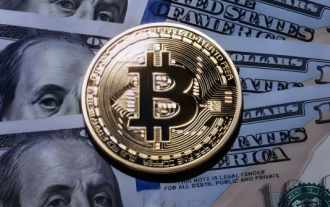
The built-in quantization tools on the exchange include: 1. Binance: Provides Binance Futures quantitative module, low handling fees, and supports AI-assisted transactions. 2. OKX (Ouyi): Supports multi-account management and intelligent order routing, and provides institutional-level risk control. The independent quantitative strategy platforms include: 3. 3Commas: drag-and-drop strategy generator, suitable for multi-platform hedging arbitrage. 4. Quadency: Professional-level algorithm strategy library, supporting customized risk thresholds. 5. Pionex: Built-in 16 preset strategy, low transaction fee. Vertical domain tools include: 6. Cryptohopper: cloud-based quantitative platform, supporting 150 technical indicators. 7. Bitsgap:
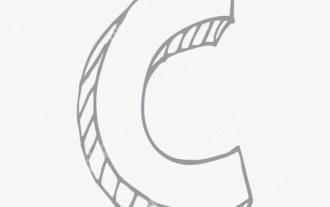
Handling high DPI display in C can be achieved through the following steps: 1) Understand DPI and scaling, use the operating system API to obtain DPI information and adjust the graphics output; 2) Handle cross-platform compatibility, use cross-platform graphics libraries such as SDL or Qt; 3) Perform performance optimization, improve performance through cache, hardware acceleration, and dynamic adjustment of the details level; 4) Solve common problems, such as blurred text and interface elements are too small, and solve by correctly applying DPI scaling.
