Why does the onChange event in React fire multiple times when input?
OnChange event triggers multiple times in React: In-depth discussion
In React development, the onChange
event of the input box is sometimes accidentally triggered multiple times. This article will analyze this problem in depth and provide a solution.
Problem description
A simple React component that manages state using useState
hook and updates the state and prints in onChange
event in the input box. However, when entering a character, the console prints twice. This phenomenon is especially obvious when using object type states, but does not occur when using primitive type states.
Sample code snippet (problem code):
import React, { useState } from "react"; export default function Child() { const [state, setState] = useState({}); const onChange = (event) => { setState({ ...state, value: event.target.value }); console.log("onChange triggered", state); }; Return ( <div> <input type="text" onchange="{onChange}"> </div> ); }
Problem analysis
The root of this problem lies in React's Strict Mode. In a development environment, Strict Mode performs two renderings to help developers discover potential problems, such as unnecessary side effects.
When the state is object type, setState
updates the reference to the object, not the value itself. Double rendering of Strict Mode causes onChange
event to be called twice, each time the same object reference is updated. The original type state (such as strings, numbers) directly updates the value, so this problem will not occur.
root cause
- Reference update of object type state: When using an object as state,
setState
will create a new object, butconsole.log
inside theonChange
function still prints the old state because of React's asynchronous update mechanism. The status is updated to a new value only during the second rendering. - Dual rendering of Strict Mode: Strict Mode in the development environment triggers dual rendering, exacerbating this problem.
Solution
Avoid using object type state, or optimize the call method of setState
:
Method 1: Use the original type state
Change the state to the original type, such as a string:
import React, { useState } from "react"; export default function Child() { const [inputValue, setInputValue] = useState(""); const onChange = (event) => { setInputValue(event.target.value); console.log("onChange triggered", inputValue); }; Return ( <div> <input type="text" value="{inputValue}" onchange="{onChange}"> </div> ); }
Method 2: Use functional updates
Use functional updates setState
to ensure that each update is based on the latest status:
import React, { useState } from "react"; export default function Child() { const [state, setState] = useState({}); const onChange = (event) => { setState((prevState) => ({ ...prevState, value: event.target.value })); console.log("onChange triggered", state); }; Return ( <div> <input type="text" onchange="{onChange}"> </div> ); }
Through the above methods, the problem of onChange
event triggering multiple times in React can be effectively solved. Remember, Strict Mode is disabled in production environments, so this problem usually only occurs in development environments.
The above is the detailed content of Why does the onChange event in React fire multiple times when input?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
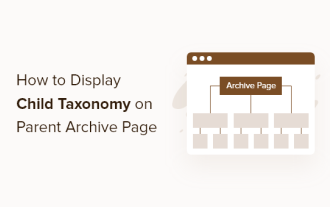
Do you want to know how to display child categories on the parent category archive page? When you customize a classification archive page, you may need to do this to make it more useful to your visitors. In this article, we will show you how to easily display child categories on the parent category archive page. Why do subcategories appear on parent category archive page? By displaying all child categories on the parent category archive page, you can make them less generic and more useful to visitors. For example, if you run a WordPress blog about books and have a taxonomy called "Theme", you can add sub-taxonomy such as "novel", "non-fiction" so that your readers can
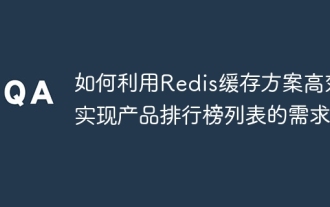
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
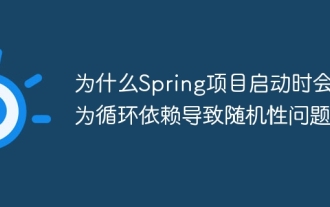
Understand the randomness of circular dependencies in Spring project startup. When developing Spring project, you may encounter randomness caused by circular dependencies at project startup...
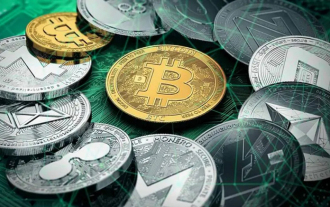
Factors of rising virtual currency prices include: 1. Increased market demand, 2. Decreased supply, 3. Stimulated positive news, 4. Optimistic market sentiment, 5. Macroeconomic environment; Decline factors include: 1. Decreased market demand, 2. Increased supply, 3. Strike of negative news, 4. Pessimistic market sentiment, 5. Macroeconomic environment.
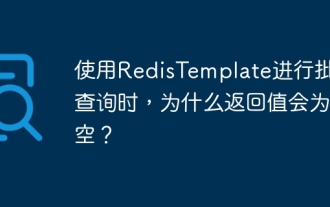
Why is the return value empty when using RedisTemplate for batch query? When using RedisTemplate for batch query operations, you may encounter the returned results...
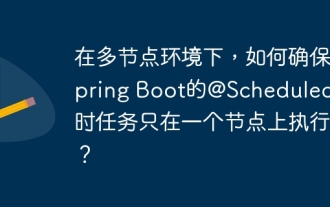
The optimization solution for SpringBoot timing tasks in a multi-node environment is developing Spring...
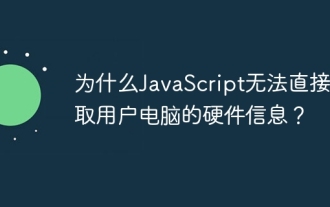
Discussion on the reasons why JavaScript cannot obtain user computer hardware information In daily programming, many developers will be curious about why JavaScript cannot be directly obtained...
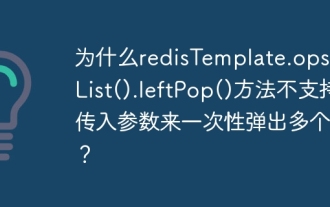
Regarding the reason why RedisTemplate.opsForList().leftPop() does not support passing numbers. When using Redis, many developers will encounter a problem: Why redisTempl...
