What are the three ways to call function in C language?
There are three ways to call C function: direct call (compiler embedded function address), pointer call (indirect call through pointer), and function pointer call (passing function pointer as argument).
Three ways to call C function? This question is a bit too superficial, but there are many tricks hidden behind it. Simply put, it is direct call, pointer call and function pointer call. But this is just the most superficial classification, and the real understanding must be in-depth at the memory model and compiler optimization levels.
Direct call: The most common guy
It's like you're calling your friend's name directly, there's nothing fancy. The compiler will directly embed the function's address into the calling instruction. Simple, direct, and the most efficient. But the disadvantage is that the function address is determined at compile time and lacks flexibility.
<code class="c">#include <stdio.h> void myFunc() { printf("Direct call!\n"); } int main() { myFunc(); // 直接调用return 0; }</stdio.h></code>
This code is clear at a glance, nothing to say. However, you have to understand that the compiler does a lot of things behind it. It has to find the address of myFunc
and generate a jump instruction.
Pointer call: Play with memory address
It's like holding a map of a friend's house (pointer) in your hand and then finding a friend's house (function) based on the map. You have to get the address of the function first and then call it indirectly through the pointer. This thing is much more flexible, and you can dynamically decide which function to call.
<code class="c">#include <stdio.h> void myFunc() { printf("Pointer call!\n"); } int main() { void (*funcPtr)() = myFunc; // 定义一个函数指针funcPtr(); // 通过函数指针调用return 0; }</stdio.h></code>
Here funcPtr
is the function pointer, which points to the address of myFunc
. Pay attention to the declaration of function pointers, which is a place where errors are prone to errors. Moreover, when calling with pointer, be careful about the validity of the pointer, otherwise segfaults are prone to occur.
Function pointer as parameters: Advanced gameplay
It's like you invite friends to a party, but you don't know who the friends are, you just know that they all dance (function). You can pass a function pointer as an argument to another function, and let the other function choose which function to call according to the situation.
<code class="c">#include <stdio.h> typedef void (*FuncPtr)(); // 定义函数指针类型void callFunc(FuncPtr func) { func(); } void func1() { printf("Function 1 called!\n"); } void func2() { printf("Function 2 called!\n"); } int main() { callFunc(func1); // 传递func1的地址callFunc(func2); // 传递func2的地址return 0; }</stdio.h></code>
The callFunc
function takes a function pointer as an argument and then calls it. This method can implement very flexible code design, for example, the callback function mechanism relies heavily on this method. However, the code complexity has also increased accordingly, and debugging is also more troublesome.
Deeper thinking:
In fact, the fundamental difference between these three methods lies in how the function address is obtained and used. Direct calls are the easiest, the compiler helps you do everything; pointer calls require programmers to manually operate the function address; function pointers as parameters increase code flexibility, but also have higher complexity. Which method to choose depends on your specific needs and code design. Don't forget to consider the readability, maintainability and performance of your code. Sometimes, seemingly simple direct calls may not be efficient when facing complex scenarios. Choosing the most suitable solution is the kingly way. Remember, the art of programming is not about showing off skills, but about solving problems.
The above is the detailed content of What are the three ways to call function in C language?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










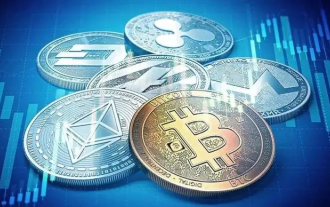
Created by Ripple, Ripple is used for cross-border payments, which are fast and low-cost and suitable for small transaction payments. After registering a wallet and exchange, purchase and storage can be made.
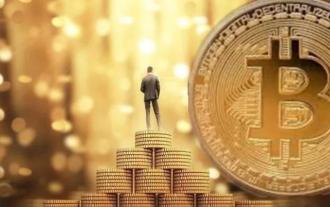
Recommended reliable digital currency trading platforms: 1. OKX, 2. Binance, 3. Coinbase, 4. Kraken, 5. Huobi, 6. KuCoin, 7. Bitfinex, 8. Gemini, 9. Bitstamp, 10. Poloniex, these platforms are known for their security, user experience and diverse functions, suitable for users at different levels of digital currency transactions
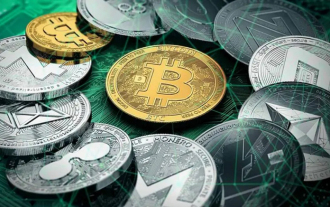
The top ten cryptocurrency trading platforms in the world include Binance, OKX, Gate.io, Coinbase, Kraken, Huobi Global, Bitfinex, Bittrex, KuCoin and Poloniex, all of which provide a variety of trading methods and powerful security measures.
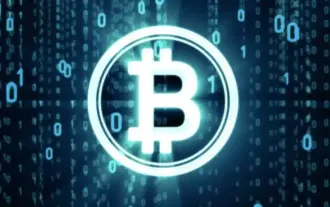
The top ten digital currency exchanges such as Binance, OKX, gate.io have improved their systems, efficient diversified transactions and strict security measures.
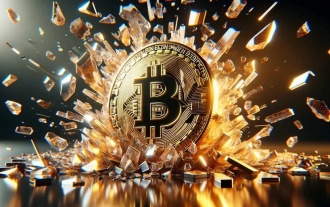
Bitcoin’s price ranges from $20,000 to $30,000. 1. Bitcoin’s price has fluctuated dramatically since 2009, reaching nearly $20,000 in 2017 and nearly $60,000 in 2021. 2. Prices are affected by factors such as market demand, supply, and macroeconomic environment. 3. Get real-time prices through exchanges, mobile apps and websites. 4. Bitcoin price is highly volatile, driven by market sentiment and external factors. 5. It has a certain relationship with traditional financial markets and is affected by global stock markets, the strength of the US dollar, etc. 6. The long-term trend is bullish, but risks need to be assessed with caution.
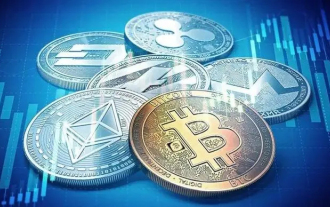
Currently ranked among the top ten virtual currency exchanges: 1. Binance, 2. OKX, 3. Gate.io, 4. Coin library, 5. Siren, 6. Huobi Global Station, 7. Bybit, 8. Kucoin, 9. Bitcoin, 10. bit stamp.
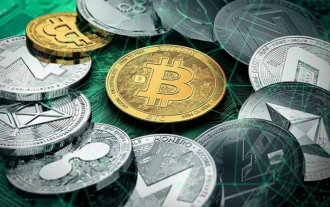
The top ten cryptocurrency exchanges in the world in 2025 include Binance, OKX, Gate.io, Coinbase, Kraken, Huobi, Bitfinex, KuCoin, Bittrex and Poloniex, all of which are known for their high trading volume and security.
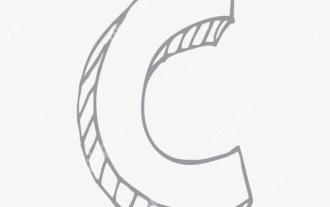
Measuring thread performance in C can use the timing tools, performance analysis tools, and custom timers in the standard library. 1. Use the library to measure execution time. 2. Use gprof for performance analysis. The steps include adding the -pg option during compilation, running the program to generate a gmon.out file, and generating a performance report. 3. Use Valgrind's Callgrind module to perform more detailed analysis. The steps include running the program to generate the callgrind.out file and viewing the results using kcachegrind. 4. Custom timers can flexibly measure the execution time of a specific code segment. These methods help to fully understand thread performance and optimize code.
