What is the definition and format of call function in C language?
Function definition and call format: Function definition: return_type function_name(parameter_type parameter1, parameter_type parameter2, ...) { ... //Function body// ... return value; } Function call: function_name(argument1, argument2, ...);
C language function, this thing is simple and simple, but complicated, it can also be a bit of a mess. Let's get straight to the point and answer your question first: What is the format of function definition and call?
Defining a function is like building a house for it. It must have a house number (function name), a room (function body), and also explain what the house is used for (return value type), and what is needed to move in (parameter list). Therefore, the standard format looks like this:
<code class="c">return_type function_name(parameter_type parameter1, parameter_type parameter2, ...) { // 函数体,也就是干活的地方// ...一堆代码... return value; // 返回值,如果函数有返回值的话}</code>
return_type
specifies what type of data the function returns, such as int
, float
, char
, or void
means that no value is returned. function_name
is the name of the function, which must comply with the naming rules of C language. You cannot use keywords, so it is best to know the name. parameter_type
, parameter1
, parameter2
and other parameters are the parameter types and names of functions. They can have multiple parameters or no parameters. The part enclosed in {...}
is the function body, which writes the code you want to execute. return value
is the return value of the function. If return_type
is not void
, there must be a return value.
Calling a function is like when you go to live in that house, you have to know the house number (function name), and you have to prepare the corresponding "baggage" (parameters). Call format:
<code class="c">function_name(argument1, argument2, ...);</code>
function_name
is the function name you want to call, argument1
and argument2
are the parameters passed to the function, and the type must match the parameter type in the function definition. If the function has a return value, you can assign the return value to a variable, or use the return value directly.
This seems simple, but there are many pitfalls hidden inside. For example, is the method of parameter passing, is it a value passing or an address passing? This directly affects whether the modification of parameters inside the function will affect external variables. When passing the value, the function internally modifies a copy of the parameters, and the external variables are not affected; when passing the address, the function internally modifies the external variable itself. This has to be chosen according to the actual situation. If you use it wrong, the bug will come, making you suspicious of your life.
For example, if the recursive call of a function is not handled properly, it is easy to cause stack overflow and program crash. Also, although the function prototype declaration looks inconspicuous, if it is not declared correctly, the compiler may report an error or some unpredictable errors.
Finally, what I want to say is that when writing functions, you must pay attention to the readability and maintainability of the code. The function should be single, and do not write it as a "hodgepodge"; the function should be as few as possible, and do not exceed five; the function comments should be written clearly to facilitate others (and to your future understanding). This seems to be a detail, but these details determine the quality of your code and the efficiency of your future maintenance code. Don’t think it’s troublesome. These good habits can help you avoid many detours. Remember, writing code is not only to make the machine understand, but also to make people understand.
The above is the detailed content of What is the definition and format of call function in C language?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
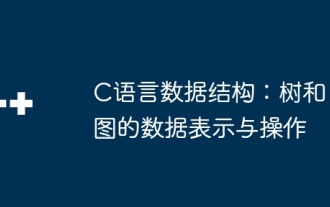
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
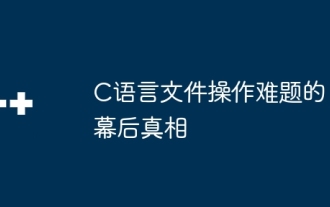
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
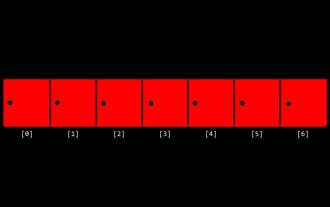
Algorithms are the set of instructions to solve problems, and their execution speed and memory usage vary. In programming, many algorithms are based on data search and sorting. This article will introduce several data retrieval and sorting algorithms. Linear search assumes that there is an array [20,500,10,5,100,1,50] and needs to find the number 50. The linear search algorithm checks each element in the array one by one until the target value is found or the complete array is traversed. The algorithm flowchart is as follows: The pseudo-code for linear search is as follows: Check each element: If the target value is found: Return true Return false C language implementation: #include#includeintmain(void){i
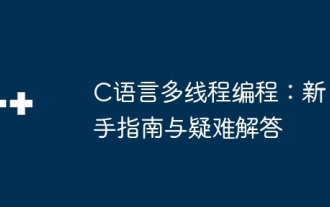
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
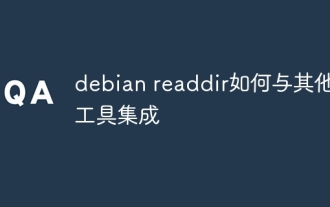
The readdir function in the Debian system is a system call used to read directory contents and is often used in C programming. This article will explain how to integrate readdir with other tools to enhance its functionality. Method 1: Combining C language program and pipeline First, write a C program to call the readdir function and output the result: #include#include#include#includeintmain(intargc,char*argv[]){DIR*dir;structdirent*entry;if(argc!=2){
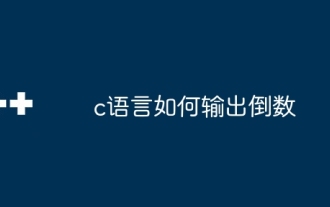
How to output a countdown in C? Answer: Use loop statements. Steps: 1. Define the variable n and store the countdown number to output; 2. Use the while loop to continuously print n until n is less than 1; 3. In the loop body, print out the value of n; 4. At the end of the loop, subtract n by 1 to output the next smaller reciprocal.
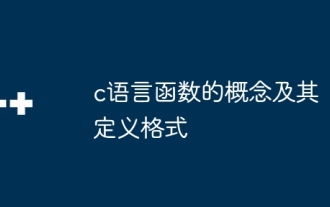
C language functions are reusable code blocks, receive parameters for processing, and return results. It is similar to the Swiss Army Knife, powerful and requires careful use. Functions include elements such as defining formats, parameters, return values, and function bodies. Advanced usage includes function pointers, recursive functions, and callback functions. Common errors are type mismatch and forgetting to declare prototypes. Debugging skills include printing variables and using a debugger. Performance optimization uses inline functions. Function design should follow the principle of single responsibility. Proficiency in C language functions can significantly improve programming efficiency and code quality.
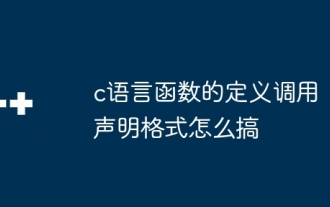
C language functions include definitions, calls and declarations. Function definition specifies function name, parameters and return type, function body implements functions; function calls execute functions and provide parameters; function declarations inform the compiler of function type. Value pass is used for parameter pass, pay attention to the return type, maintain a consistent code style, and handle errors in functions. Mastering this knowledge can help write elegant, robust C code.
