Nested and recursive calls of c language functions
Function nested calls are similar to dolls, function A calls function B, and B may call C; recursive calls are like a mirror, and the function itself calls itself. Nested calls improve readability, but too many layers will reduce the difficulty of understanding; recursive calls are suitable for self-similar problems, but there is a risk of stack overflow. Performance, nested calls are better than recursive calls, but for specific problems, recursive code is more concise. Use nesting and recursion with caution, avoid code complications, and pursue concise and elegant high-quality code.
Nested and recursive calls of C functions: maze and echo
You may ask: What is the difference between nested function calls and recursive calls? How should they be controlled gracefully? Simply put, nested calls are like Russian dolls one by one, while recursive calls are more like a mirror, constantly reflecting themselves. After reading this article, you will be able to distinguish between these two ways of calling and master some tips to avoid falling into common traps.
Basic preparation: the soul of function
In the C world, functions are the cornerstone of code, breaking complex tasks into smaller, easy-to-manage modules. Understanding the definition of functions, parameter passing and return values is the key to mastering nesting and recursion. Imagine a function is an independent room with its own entrance (parameter), operation space (function body) and exit (return value).
Nesting Call: The Art of a Doll
Function nested calls are like putting one doll into another. A function A calls another function B within its function body, and function B may call function C, and so on. This is a very common programming technique that improves the readability and maintainability of your code.
<code class="c">#include <stdio.h> int add(int a, int b) { return ab; } int multiply(int a, int b) { return a * b; } int main() { int x = 5, y = 10; int sum = add(x, y); // 函数add的调用int result = multiply(sum, 2); // 函数multiply的调用,嵌套调用add printf("Result: %d\n", result); return 0; }</stdio.h></code>
In this example, main
function calls the add
function, and the multiply
function uses the return value of the add
function. This reflects the simplicity of nested calls of functions. It should be noted that too many nested call layers may make the code difficult to understand and debug, so keep it moderate.
Recursive call: World in the Mirror
Recursive calls are like a mirror, and the function itself calls itself. It requires a clear terminating condition or it will fall into an infinite loop, like falling into a bottomless pit. Recursion is often used to solve problems with self-similar structures such as factorial calculations, Fibonacci sequences and traversals of trees.
<code class="c">#include <stdio.h> int factorial(int n) { if (n == 0) { return 1; // 终止条件} else { return n * factorial(n - 1); // 递归调用} } int main() { int num = 5; int result = factorial(num); printf("Factorial of %d is %d\n", num, result); return 0; }</stdio.h></code>
This example calculates factorial. factorial
function calls itself until n
equals 0, and then the recursion ends. Recursion is elegant, but it also has some problems: Stack overflow is the biggest risk of recursive calls, especially when dealing with large data. In addition, debugging of recursive code is also relatively difficult. You need to carefully design the termination conditions and monitor the usage of the stack.
Advanced: Performance and Traps
Function nested calls are usually better performance than recursive calls, because recursive calls can generate a lot of function call overhead, which consumes more memory and time. However, for certain specific problems, recursion can provide a more concise and easy to understand solution. Which method to choose depends on the specific problem and your programming style. Remember that excessive nesting or recursion can make the code difficult to maintain, so use it with caution.
Experience Talk: The Poetics of Code
Writing code is like writing poetry, which requires conciseness, elegance and efficiency. Function nesting and recursion are both powerful tools, but they need to be used with caution. Only by understanding their advantages and disadvantages and choosing the right way according to actual conditions can you write high-quality code. Remember that the readability and maintainability of the code are more important than the skills. Avoid over-complex nesting and recursion, making your code as clear and smooth as a beautiful poem, easy to understand.
The above is the detailed content of Nested and recursive calls of c language functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
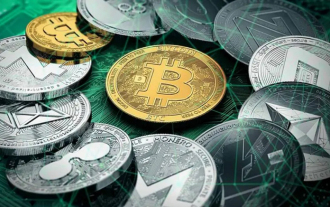
The top ten cryptocurrency trading platforms in the world include Binance, OKX, Gate.io, Coinbase, Kraken, Huobi Global, Bitfinex, Bittrex, KuCoin and Poloniex, all of which provide a variety of trading methods and powerful security measures.

MeMebox 2.0 redefines crypto asset management through innovative architecture and performance breakthroughs. 1) It solves three major pain points: asset silos, income decay and paradox of security and convenience. 2) Through intelligent asset hubs, dynamic risk management and return enhancement engines, cross-chain transfer speed, average yield rate and security incident response speed are improved. 3) Provide users with asset visualization, policy automation and governance integration, realizing user value reconstruction. 4) Through ecological collaboration and compliance innovation, the overall effectiveness of the platform has been enhanced. 5) In the future, smart contract insurance pools, forecast market integration and AI-driven asset allocation will be launched to continue to lead the development of the industry.
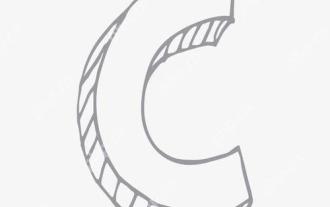
Using the chrono library in C can allow you to control time and time intervals more accurately. Let's explore the charm of this library. C's chrono library is part of the standard library, which provides a modern way to deal with time and time intervals. For programmers who have suffered from time.h and ctime, chrono is undoubtedly a boon. It not only improves the readability and maintainability of the code, but also provides higher accuracy and flexibility. Let's start with the basics. The chrono library mainly includes the following key components: std::chrono::system_clock: represents the system clock, used to obtain the current time. std::chron
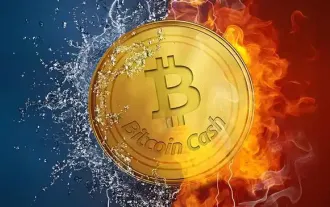
Bitcoin’s price fluctuations today are affected by many factors such as macroeconomics, policies, and market sentiment. Investors need to pay attention to technical and fundamental analysis to make informed decisions.
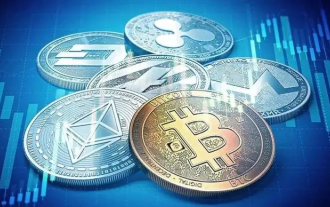
Created by Ripple, Ripple is used for cross-border payments, which are fast and low-cost and suitable for small transaction payments. After registering a wallet and exchange, purchase and storage can be made.
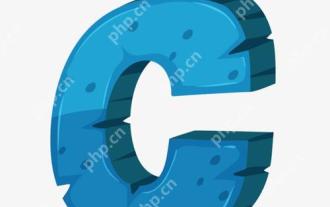
ABI compatibility in C refers to whether binary code generated by different compilers or versions can be compatible without recompilation. 1. Function calling conventions, 2. Name modification, 3. Virtual function table layout, 4. Structure and class layout are the main aspects involved.
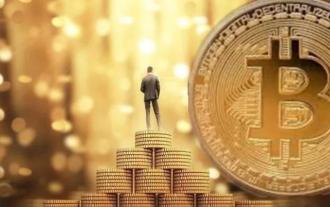
Recommended reliable digital currency trading platforms: 1. OKX, 2. Binance, 3. Coinbase, 4. Kraken, 5. Huobi, 6. KuCoin, 7. Bitfinex, 8. Gemini, 9. Bitstamp, 10. Poloniex, these platforms are known for their security, user experience and diverse functions, suitable for users at different levels of digital currency transactions
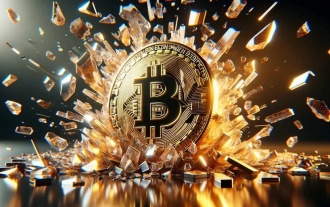
Bitcoin’s price ranges from $20,000 to $30,000. 1. Bitcoin’s price has fluctuated dramatically since 2009, reaching nearly $20,000 in 2017 and nearly $60,000 in 2021. 2. Prices are affected by factors such as market demand, supply, and macroeconomic environment. 3. Get real-time prices through exchanges, mobile apps and websites. 4. Bitcoin price is highly volatile, driven by market sentiment and external factors. 5. It has a certain relationship with traditional financial markets and is affected by global stock markets, the strength of the US dollar, etc. 6. The long-term trend is bullish, but risks need to be assessed with caution.
