The difference between function declaration and call in C language
Function declarations explicitly define the function interface, including return type, function name, and parameter list; function calls use declared functions, pass parameters and receive return values. Not only is the declaration for compile-time type checking, it also enhances code modularity and readability. Function calls are passed using value, except pointer parameters; array parameters pass the first address. The compiler finds function definitions and executes, including address search, parameter passing and return value processing. Be careful to declare and use parameter types, avoid pointer operation errors, and write high-quality C code in best practices.
C function declarations and calls: those details you may ignore
You may think that C function declarations and calls are a piece of cake, just telling the compiler what the function looks like and then using it? But in fact, there are many mysteries hidden behind this, and you may fall into the pit. In this article, we will explore function declarations and calls in depth to help you avoid potential traps and write more robust and efficient C code.
Let’s talk about the conclusion first: the function declaration tells the compiler the interface of the function - the return type, function name, and parameter list; the function call actually uses the function, passes the parameters and receives the return value. It seems simple, but details determine success or failure.
Basic Review: Compiler Perspective
The compiler is a meticulous guy and it requires a complete understanding of your code during the compilation process. A function declaration is equivalent to giving the compiler a "instruction book" to tell it the function signature:返回值类型函数名(参数类型参数名, ...);
. For example: int add(int a, int b);
This tells the compiler: the add
function receives two integer parameters and returns an integer value. Without a declaration, the compiler cannot determine the type of the function when encountering a function call, resulting in a compilation error.
Necessity of declaration: more than just compilation
Many newbies think that if the function definition is before being called, the declaration seems redundant. But in fact, the statement has other important functions:
- Modular programming: In large projects, functions are often scattered in different source files. Declaration allows you to use functions defined in another file in one file without knowing the specific implementation details of the function in advance. This greatly improves the maintainability and reusability of the code.
- Code readability: Clear declarations can enhance the readability of the code even if the function definition is called, making the code easier to understand and maintain. Imagine how bad a long function definition is before the call is called.
Call: The art of parameter passing
When calling a function, the way parameters are passed is crucial. C language uses "value transfer", which means that the function receives a copy of the parameters, not the parameters themselves. This means that modifying the value of the parameter inside the function will not affect the original parameter in the calling function. This is very important to avoid unexpected data modifications between functions, but we also need to pay attention to:
- Pointer parameters: If you need to modify variables in the calling function inside the function, you need to use pointer parameters. The pointer passes the address of the variable, not a copy of the variable. The function can change the value of the variable through pointer modification.
- Array parameters: When an array is passed as a parameter, the first address of the array is actually passed. Functions can access all elements in an array, but you need to pay attention to the size of the array to avoid out-of-bounds access.
In-depth discussion: How does the compiler handle it?
When the compiler encounters a function call, it will find the definition of the function based on the function declaration and then generate the corresponding machine code to execute the function. This process involves multiple steps such as address search, parameter passing and return value processing of functions. Understanding these underlying details will help you write more efficient code. For example, reducing the number of function calls can improve program performance.
The guide to the pit: those common mistakes
- Declaration is inconsistent with definition: This is the most common mistake. Inconsistent declaration and definition return value types, function names, or parameter lists can lead to compilation errors or runtime errors.
- Parameter type mismatch: The passed parameter type does not match the parameter type specified in the function declaration, which can also lead to an error.
- Forgot to declare: Forgot to declare before using the function, the compiler will report an error.
- Pointer operation error: The use of pointer parameters is relatively complicated, and a little carelessness will cause the program to crash.
Best Practice: Write elegant C code
- Always declare functions: Develop good programming habits and always declare functions before using them.
- Use meaningful function and parameter names: This improves the readability and maintainability of the code.
- Double-check parameter types: Make sure the parameter types are consistent with the type specified in the function declaration.
- Use pointers with caution: Understand the mechanism of pointers and avoid pointer operation errors.
- Write unit tests: Test your functions to make sure they work correctly.
In short, C function declarations and calls seem simple, but there are rich knowledge and skills behind it. Only by deeply understanding these details can we write more efficient, robust and easier to maintain C code. Remember, details determine success or failure, which not only applies to C, but also to every aspect of programming.
The above is the detailed content of The difference between function declaration and call in C language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
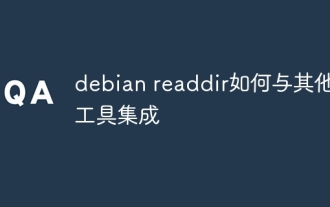
The readdir function in the Debian system is a system call used to read directory contents and is often used in C programming. This article will explain how to integrate readdir with other tools to enhance its functionality. Method 1: Combining C language program and pipeline First, write a C program to call the readdir function and output the result: #include#include#include#includeintmain(intargc,char*argv[]){DIR*dir;structdirent*entry;if(argc!=2){
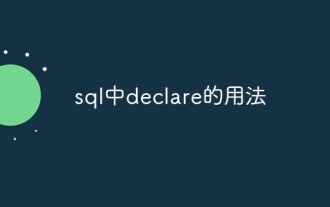
The DECLARE statement in SQL is used to declare variables, that is, placeholders that store variable values. The syntax is: DECLARE <Variable name> <Data type> [DEFAULT <Default value>]; where <Variable name> is the variable name, <Data type> is its data type (such as VARCHAR or INTEGER), and [DEFAULT <Default value>] is an optional initial value. DECLARE statements can be used to store intermediates
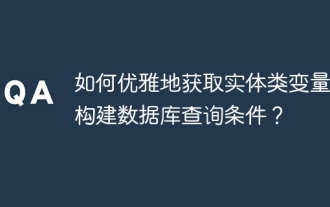
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
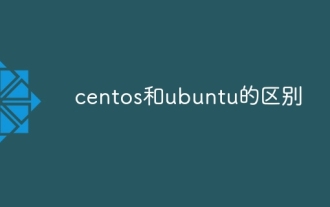
The key differences between CentOS and Ubuntu are: origin (CentOS originates from Red Hat, for enterprises; Ubuntu originates from Debian, for individuals), package management (CentOS uses yum, focusing on stability; Ubuntu uses apt, for high update frequency), support cycle (CentOS provides 10 years of support, Ubuntu provides 5 years of LTS support), community support (CentOS focuses on stability, Ubuntu provides a wide range of tutorials and documents), uses (CentOS is biased towards servers, Ubuntu is suitable for servers and desktops), other differences include installation simplicity (CentOS is thin)
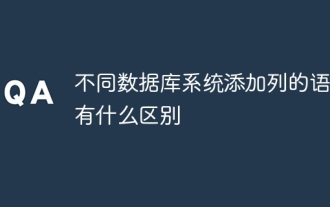
不同数据库系统添加列的语法为:MySQL:ALTER TABLE table_name ADD column_name data_type;PostgreSQL:ALTER TABLE table_name ADD COLUMN column_name data_type;Oracle:ALTER TABLE table_name ADD (column_name data_type);SQL Server:ALTER TABLE table_name ADD column_name data_
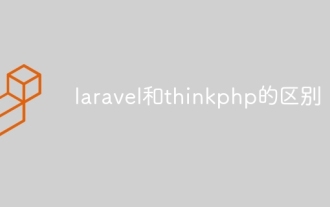
Laravel and ThinkPHP are both popular PHP frameworks and have their own advantages and disadvantages in development. This article will compare the two in depth, highlighting their architecture, features, and performance differences to help developers make informed choices based on their specific project needs.
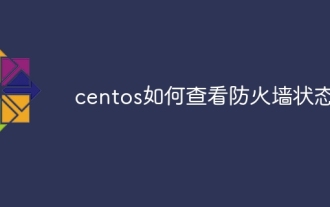
The state of the CentOS firewall can be viewed through the sudo firewall-cmd --state command, returning to running or not running. For more detailed information, you can use sudo firewall-cmd --list-all to view, including configured areas, services, ports, etc. If firewall-cmd does not solve the problem, you can use sudo iptables -L -n to view iptables rules. Be sure to make a backup before modifying the firewall configuration to ensure server security.
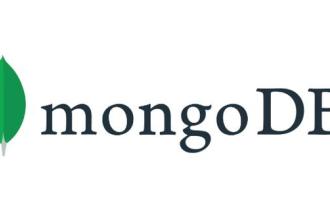
Choosing MongoDB or relational database depends on application requirements. 1. Relational databases (such as MySQL) are suitable for applications that require high data integrity and consistency and fixed data structures, such as banking systems; 2. NoSQL databases such as MongoDB are suitable for processing massive, unstructured or semi-structured data and have low requirements for data consistency, such as social media platforms. The final choice needs to weigh the pros and cons and decide based on the actual situation. There is no perfect database, only the most suitable database.
