


How to efficiently store and read Go structure arrays to Redis using go-redis/redis v8?
This article discusses how to efficiently store and read Go structure arrays to Redis using go-redis/redis v8
Saving the Go structure array directly to Redis will cause an error, because the SET
command of go-redis/redis v8
only supports string type key-value pairs.
The following code snippet demonstrates trying to store an array of type []model.sysrolemenu
directly to Redis and explains the cause of the error. The code is designed to get the menu tree structure and store it in Redis for quick access. After getting the getmenutree
tree data, I tried to use global.gva_redis.set
to directly store the menus
array, but because SET
method requires the value to be a string type, it failed.
The workaround is to convert the []model.sysrolemenu
array to a JSON string supported by Redis. After serializing it into a JSON string, it can be successfully stored in Redis; when reading it, it will be deserialized back to the Go structure array.
The modified getmenutree
function is as follows, converting menus
arrays to JSON strings before saving to Redis:
import ( "context" "encoding/json" "github.com/go-redis/redis/v8" "go.uber.org/zap" ) // ... other imports and code ... func GetMenuTree(roleId string) (err error, menus []model.SysRoleMenu) { err, menuTree := GetMenuTreeMap(roleId) menus = menuTree["0"] // ... other code ... jsonData, err := json.Marshal(menus) if err != nil { zap.L().Error("json marshal error", zap.Error(err)) return err, nil } err = global.gvaRedis.Set(context.Background(), "menuTree:" roleId, string(jsonData), 0).Err() if err != nil { zap.L().Error("redis set error", zap.Error(err)) return err, nil } return nil, menus } //A sample to read data func ReadMenuTree(roleId string) (err error, menus []model.SysRoleMenu) { val, err := global.gvaRedis.Get(context.Background(), "menuTree:" roleId).Result() if err != nil { if err == redis.Nil { return nil, nil //Key does not exist} return err, nil } err = json.Unmarshal([]byte(val), &menus) if err != nil { zap.L().Error("json unmarshal error", zap.Error(err)) return err, nil } return nil, menus }
Encode menus
array into a JSON string through the json.Marshal
function and store it in Redis. When reading data, use json.Unmarshal
for deserialization. This solves the problem that go-redis/redis v8
does not support direct storage of array structures.
The above is the detailed content of How to efficiently store and read Go structure arrays to Redis using go-redis/redis v8?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
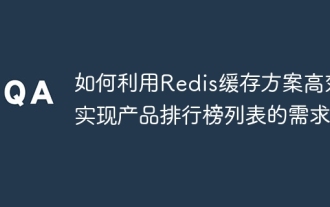
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
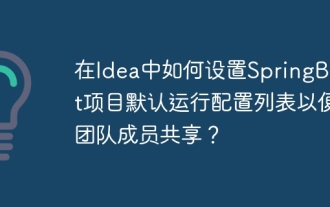
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...
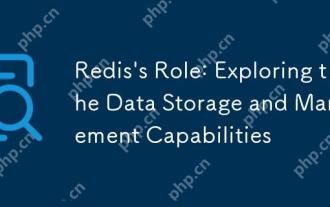
Redis plays a key role in data storage and management, and has become the core of modern applications through its multiple data structures and persistence mechanisms. 1) Redis supports data structures such as strings, lists, collections, ordered collections and hash tables, and is suitable for cache and complex business logic. 2) Through two persistence methods, RDB and AOF, Redis ensures reliable storage and rapid recovery of data.
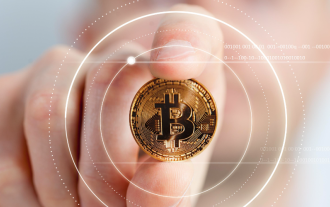
The steps to draw a Bitcoin structure analysis chart include: 1. Determine the purpose and audience of the drawing, 2. Select the right tool, 3. Design the framework and fill in the core components, 4. Refer to the existing template. Complete steps ensure that the chart is accurate and easy to understand.
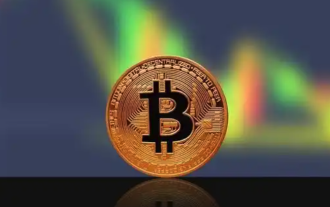
Cryptocurrency data platforms suitable for beginners include CoinMarketCap and non-small trumpet. 1. CoinMarketCap provides global real-time price, market value, and trading volume rankings for novice and basic analysis needs. 2. The non-small quotation provides a Chinese-friendly interface, suitable for Chinese users to quickly screen low-risk potential projects.
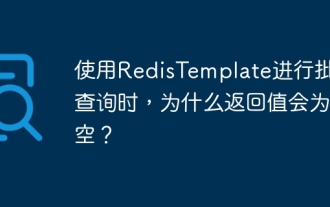
Why is the return value empty when using RedisTemplate for batch query? When using RedisTemplate for batch query operations, you may encounter the returned results...
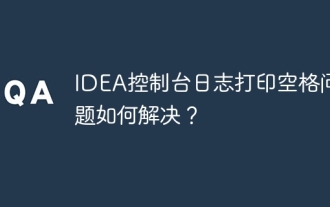
How to solve the problem of printing spaces in IDEA console logs? When using IDEA for development, many developers may encounter a problem: the console printed...
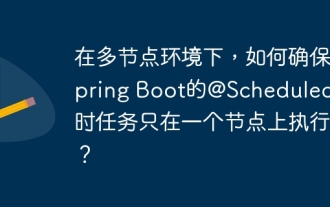
The optimization solution for SpringBoot timing tasks in a multi-node environment is developing Spring...
