


Dependency Injection Container: How it works in Laravel/Symfony.
Dependency Injection Container: How it works in Laravel/Symfony
A Dependency Injection Container (DIC) in Laravel and Symfony is a tool that manages the instantiation and lifecycle of objects, ensuring that dependencies are provided to classes without hardcoding them. Here's how it works in both frameworks:
Laravel:
In Laravel, the DIC is primarily managed through the Illuminate\Container\Container
class, which is accessible via the app()
helper function. Laravel uses a service container to resolve dependencies and manage class instances. When a class is instantiated, Laravel's container checks if the class has any dependencies defined in its constructor. If so, it resolves these dependencies recursively, ensuring all required objects are created and injected.
For example, if a controller has a dependency on a service, Laravel's container will automatically instantiate the service and inject it into the controller when it's created. Laravel also allows for binding interfaces to concrete implementations, which promotes loose coupling and makes the application more testable.
Symfony:
In Symfony, the DIC is a core component of the framework, managed through the Symfony\Component\DependencyInjection\Container
class. Symfony's container is configured via YAML, XML, or PHP files, where services and their dependencies are defined. When a service is requested, Symfony's container reads the configuration, instantiates the service, and injects its dependencies.
Symfony's container supports autowiring, which automatically detects and injects dependencies based on type hints in the constructor. This reduces the need for manual configuration and makes the setup of services more straightforward. Additionally, Symfony allows for service decoration, where one service can wrap another to extend its functionality.
What are the benefits of using a Dependency Injection Container in Laravel or Symfony?
Using a Dependency Injection Container in Laravel or Symfony offers several benefits:
- Decoupling: By injecting dependencies rather than hardcoding them, classes become more independent and easier to test. This promotes a modular architecture where components can be swapped or replaced without affecting the rest of the application.
- Reusability: With a DIC, services can be instantiated and reused across the application, reducing redundancy and improving maintainability.
- Testability: Dependency Injection makes it easier to write unit tests by allowing you to inject mock objects or test doubles, isolating the class under test from its dependencies.
- Flexibility: The DIC allows for easy configuration and reconfiguration of services. In Laravel, you can bind interfaces to different implementations at runtime, while Symfony's configuration files make it simple to adjust service definitions.
- Performance: Both frameworks optimize the instantiation of objects, caching them when possible to improve application performance.
- Centralized Management: The DIC provides a centralized place to manage the lifecycle of objects, making it easier to understand and control the flow of dependencies throughout the application.
How can I configure and manage services with a Dependency Injection Container in these frameworks?
Laravel:
In Laravel, you can configure and manage services using the service container. Here's how:
-
Binding Services: You can bind services in the
App\Providers\AppServiceProvider
class or any other service provider. Use thebind
,singleton
, orinstance
methods to define how services should be resolved.public function register() { $this->app->bind('App\Services\PaymentGateway', function ($app) { return new \App\Services\StripePaymentGateway(); }); }
Copy after login Resolving Services: Services can be resolved using the
app()
helper or dependency injection in constructors.$paymentGateway = app('App\Services\PaymentGateway');
Copy after login- Service Providers: Use service providers to organize the registration of services and their dependencies.
Symfony:
In Symfony, service configuration is typically done in YAML, XML, or PHP files located in the config/services
directory. Here's how to manage services:
Defining Services: Define services in
config/services.yaml
.services: App\Service\PaymentGateway: class: App\Service\StripePaymentGateway
Copy after loginAutowiring: Enable autowiring to automatically inject dependencies based on type hints.
services: _defaults: autowire: true
Copy after loginService Configuration: Configure services with arguments, tags, and other settings.
services: App\Service\PaymentGateway: arguments: - '@App\Service\Logger' tags: - { name: 'app.payment_gateway' }
Copy after loginAccessing Services: Services can be accessed via the container or injected into classes.
use Symfony\Component\DependencyInjection\ContainerInterface; class SomeController { private $paymentGateway; public function __construct(PaymentGateway $paymentGateway) { $this->paymentGateway = $paymentGateway; } }
Copy after login
What common issues might I encounter when implementing Dependency Injection in Laravel/Symfony, and how can I resolve them?
When implementing Dependency Injection in Laravel or Symfony, you might encounter the following issues and resolve them as follows:
-
Circular Dependencies:
- Issue: Two or more services depend on each other, causing a circular reference.
-
Resolution: Refactor the services to break the cycle. In Laravel, you can use lazy loading with the
app()->make()
method. In Symfony, you can use lazy services or refactor the dependency structure.
-
Performance Overhead:
- Issue: The DIC can introduce performance overhead due to the instantiation and management of services.
-
Resolution: Use caching mechanisms provided by the frameworks. In Laravel, you can use the
singleton
method to ensure a service is instantiated only once. In Symfony, enable service optimization and use thelazy
tag for services that are not always needed.
-
Configuration Complexity:
- Issue: Managing a large number of services and their dependencies can become complex.
- Resolution: Organize services into logical groups using service providers in Laravel or separate configuration files in Symfony. Use autowiring in Symfony to reduce manual configuration.
-
Debugging and Error Handling:
- Issue: It can be challenging to debug issues related to dependency injection, especially when errors occur during service instantiation.
-
Resolution: Use the debugging tools provided by the frameworks. In Laravel, the
dd()
function can help inspect the container's state. In Symfony, thedebug:container
command can list all services and their dependencies, helping to identify issues.
-
Testing Challenges:
- Issue: Testing classes with injected dependencies can be complex, especially when mocking services.
-
Resolution: Use mocking libraries like PHPUnit's MockObject or Mockery to create test doubles. In Laravel, you can use the
shouldReceive
method to define mock behavior. In Symfony, you can override services in the test environment to inject mocks.
By understanding these common issues and their resolutions, you can effectively implement and manage Dependency Injection in Laravel and Symfony, leading to more maintainable and scalable applications.
The above is the detailed content of Dependency Injection Container: How it works in Laravel/Symfony.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
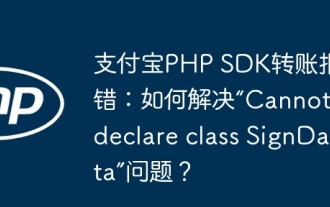
Alipay PHP...
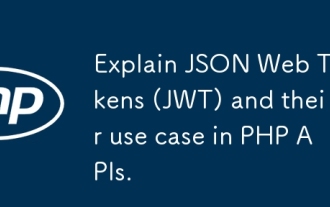
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
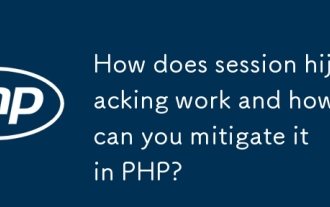
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
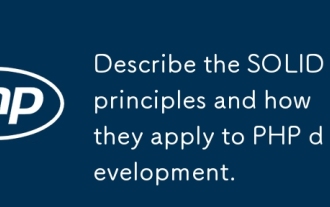
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
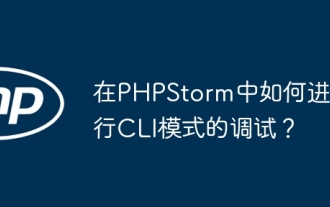
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...

The enumeration function in PHP8.1 enhances the clarity and type safety of the code by defining named constants. 1) Enumerations can be integers, strings or objects, improving code readability and type safety. 2) Enumeration is based on class and supports object-oriented features such as traversal and reflection. 3) Enumeration can be used for comparison and assignment to ensure type safety. 4) Enumeration supports adding methods to implement complex logic. 5) Strict type checking and error handling can avoid common errors. 6) Enumeration reduces magic value and improves maintainability, but pay attention to performance optimization.
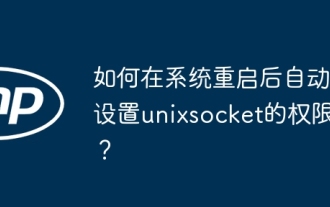
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
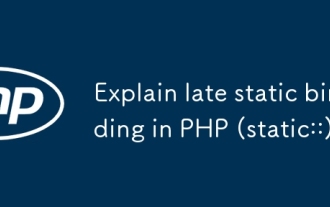
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
