


What are the differences between arrays and slices in Go? Why are slices more commonly used?
What are the differences between arrays and slices in Go? Why are slices more commonly used?
In Go, arrays and slices are both used to store sequences of elements, but they have distinct characteristics that set them apart.
Arrays in Go are fixed-size sequences of elements of the same type. When you declare an array, you must specify its size, which cannot be changed once the array is created. Arrays are value types, meaning that when an array is assigned to a new variable or passed to a function, the entire array is copied. This can be inefficient for large arrays. Arrays are typically used when the size of the sequence is known at compile time and does not need to change.
Slices, on the other hand, are more flexible and dynamic. A slice is a reference to an underlying array and consists of three parts: a pointer to the array, the length of the segment the slice represents, and the capacity (which is the maximum length the slice can reach without allocating a new underlying array). Slices can grow or shrink in size, and they are reference types, meaning that multiple slices can reference the same underlying array. This makes slices more efficient in terms of memory usage and data sharing.
Slices are more commonly used in Go because of their flexibility and efficiency. Here are some reasons why:
- Dynamic Size: Slices can grow or shrink dynamically, which is useful for scenarios where the amount of data is not known in advance.
- Efficient Memory Usage: Slices reference an underlying array, so they do not need to copy the entire array when passed around, which saves memory.
-
Ease of Use: Slices provide built-in functions like
append
andcopy
, making it easier to manipulate data. - Interoperability: Slices work well with Go's built-in functions and libraries, such as those for sorting and searching.
How can slices in Go be more efficiently managed in terms of memory?
Managing slices efficiently in terms of memory in Go involves understanding how slices work and using them appropriately. Here are some strategies to optimize memory usage with slices:
-
Reuse Slices: Instead of creating new slices, try to reuse existing ones. For example, if you need to clear a slice, you can set its length to zero (
slice = slice[:0]
) instead of creating a new slice. -
Use
append
Wisely: When usingappend
, be aware that if the slice's capacity is exceeded, a new underlying array will be allocated, which can be costly. Try to pre-allocate the slice to a size that is likely to be sufficient (slice = make([]Type, 0, estimatedSize)
). -
Avoid Unnecessary Allocations: If you know the final size of the slice, allocate it to that size from the start to avoid multiple reallocations. For example, if you're reading lines from a file, you can use
bufio.Scanner
to estimate the number of lines and pre-allocate the slice. -
Use
copy
for Efficient Data Movement: When you need to move data between slices, use thecopy
function, which is optimized for performance and can help avoid unnecessary allocations. -
Profile and Monitor Memory Usage: Use Go's built-in profiling tools like
pprof
to monitor memory usage and identify potential issues. This can help you understand where memory is being allocated and how to optimize it.
What are some common use cases where arrays are preferred over slices in Go programming?
While slices are more commonly used in Go due to their flexibility, there are specific scenarios where arrays are preferred:
- Fixed-Size Data Structures: When the size of the data structure is known and fixed, arrays are more appropriate. For example, a chessboard can be represented as an 8x8 array of squares.
- Stack-Based Operations: In certain algorithms or data structures where the size is known and fixed, such as a stack with a fixed capacity, arrays can be more efficient.
- Performance-Critical Code: In performance-critical sections of code, arrays can be faster because they avoid the overhead of dynamic resizing and reference management. For example, in a high-frequency trading system, using arrays for fixed-size buffers can be beneficial.
-
Interoperability with C Code: When interfacing with C code using
cgo
, arrays are often used because they have a direct mapping to C arrays, making it easier to pass data between Go and C. - Embedded Systems: In resource-constrained environments like embedded systems, the predictability and fixed memory usage of arrays can be advantageous.
Can you explain the performance implications of using slices versus arrays in Go?
The performance implications of using slices versus arrays in Go can be significant, depending on the context:
-
Memory Allocation and Copying:
- Arrays: Since arrays are value types, assigning an array to a new variable or passing it to a function results in a full copy of the array. This can be costly for large arrays.
-
Slices: Slices are reference types, so only the reference is copied when assigned or passed. This is more efficient, especially for large datasets. However, if the underlying array needs to be resized (e.g., when using
append
), a new array may be allocated, which can be costly.
-
Resizing and Capacity:
- Arrays: Arrays cannot be resized, which can be a limitation but also a performance advantage in scenarios where the size is fixed and known.
-
Slices: Slices can be resized using
append
, which can lead to performance overhead if the underlying array needs to be reallocated frequently. However, if the capacity is pre-allocated appropriately, this overhead can be minimized.
-
Garbage Collection:
- Arrays: Since arrays are value types, they are less likely to be affected by garbage collection, as they are not referenced by multiple variables.
- Slices: Slices can lead to more frequent garbage collection because the underlying array may be referenced by multiple slices, and when the last reference is removed, the array becomes eligible for garbage collection.
-
Cache Efficiency:
- Arrays: Arrays can be more cache-friendly because their memory layout is contiguous and predictable, which can improve performance in certain algorithms.
- Slices: Slices can also be cache-friendly if the underlying array is contiguous, but the dynamic nature of slices can sometimes lead to less predictable memory access patterns.
In summary, slices are generally more flexible and efficient for most use cases in Go, but arrays can offer performance advantages in specific scenarios where the size is fixed and known, and where the overhead of dynamic resizing and reference management is undesirable.
The above is the detailed content of What are the differences between arrays and slices in Go? Why are slices more commonly used?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










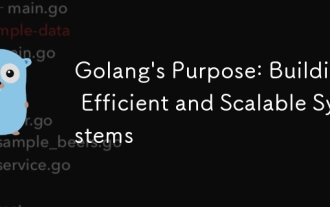
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
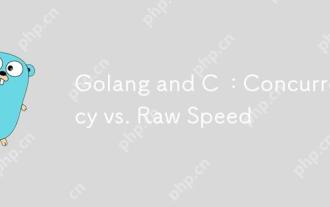
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
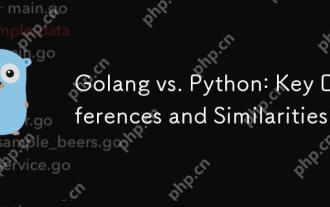
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
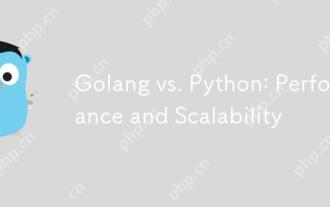
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
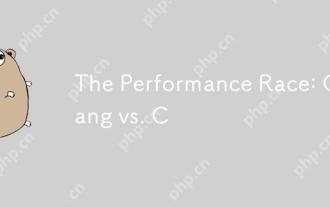
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
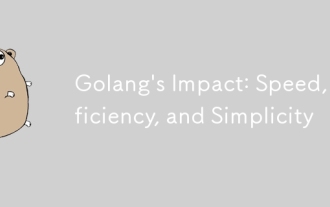
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
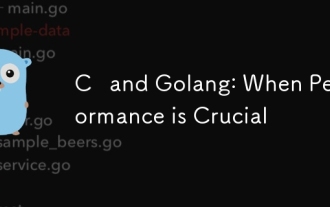
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
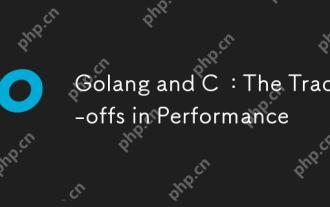
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
