Explain the difference between == and === in PHP.
Explain the difference between == and === in PHP.
In PHP, ==
and ===
are both comparison operators used to compare two values, but they do so in different ways.
-
==
(Equal to): This operator compares the values of two operands for equality, performing a type juggling before comparison. This means PHP will convert the data types of the operands if necessary, to check if their values are equal. For example, if you compare a string "5" with an integer 5 using==
, PHP will convert the string to an integer and then compare, resulting intrue
. -
===
(Identical to): This operator is stricter and compares both the value and the type of the operands. No type conversion occurs with===
. Using the same example as above, comparing a string "5" with an integer 5 using===
would result infalse
because although the values are the same, the types are different.
Here's a code snippet to illustrate:
<?php echo "5 == 5: " . (5 == "5") . "\n"; // Outputs: 5 == 5: 1 (true) echo "5 === 5: " . (5 === "5") . "\n"; // Outputs: 5 === 5: (false) ?>
Understanding the difference is crucial for writing clear and predictable code in PHP.
What are some common pitfalls to avoid when using == in PHP?
When using the ==
operator in PHP, developers should be cautious of several common pitfalls that can lead to unexpected results:
- Type Juggling: As mentioned, PHP automatically converts types when comparing with
==
. This can lead to confusing scenarios, like0 == "a"
returningtrue
because both are converted tofalse
in a boolean context. - Array Comparison: When comparing arrays,
==
checks if the arrays have the same keys and values, but it doesn't consider the order of elements. This can lead to issues if the order matters. - Object Comparison: When comparing objects,
==
checks if the two variables reference the same object instance, not if the objects contain the same data. This is different from what one might expect in many other languages. - NULL and Empty String:
NULL == ""
returnstrue
because both are consideredfalse
in a boolean context. - Numeric Strings: Strings that are numeric in nature, like "123", can be compared to integers directly, which might lead to unexpected results in logical operations.
Here's an example showing some of these issues:
<?php echo "0 == 'a': " . (0 == "a") . "\n"; // Outputs: 0 == 'a': 1 (true) echo "NULL == '': " . (NULL == "") . "\n"; // Outputs: NULL == '': 1 (true) $arr1 = [1, 2, 3]; $arr2 = [3, 2, 1]; echo "Array Comparison: " . ($arr1 == $arr2) . "\n"; // Outputs: Array Comparison: 1 (true) ?>
Being aware of these pitfalls can help in writing more robust code.
How can using === improve the accuracy of comparisons in PHP code?
Using the ===
operator in PHP can significantly improve the accuracy and predictability of comparisons by eliminating the type juggling that occurs with ==
. Here’s how it can be beneficial:
- Consistency and Predictability: By comparing both value and type,
===
ensures that comparisons are performed in a more straightforward and predictable manner. This reduces the likelihood of unexpected results due to automatic type conversion. - Error Prevention: Using
===
helps prevent errors that might arise from comparing different data types. For example, it will distinguish between integers and strings, null and empty strings, etc., which can be critical in decision-making logic. - Better Debugging: When debugging code, having
===
in place makes it easier to identify issues since the comparison results are more transparent and less subject to PHP's type juggling rules. - Security: In contexts like authentication or data validation, using
===
can add an extra layer of security by ensuring that inputs are strictly of the expected type and value.
Consider the following example to see how ===
can improve accuracy:
<?php function validateInput($input) { if ($input === "admin") { echo "Valid input"; } else { echo "Invalid input"; } } validateInput("admin"); // Outputs: Valid input validateInput(0); // Outputs: Invalid input, even though 0 == "admin" would be true ?>
In this example, using ===
ensures that only the exact string "admin" is accepted, preventing unexpected matches.
In what scenarios should you prefer === over == in PHP?
Prefer using ===
over ==
in the following scenarios in PHP to ensure the accuracy and reliability of your comparisons:
- Security-Critical Code: When validating user inputs or in security-related operations such as authentication,
===
ensures exact type and value matches, reducing the risk of exploitation due to type juggling. - Strict Data Validation: When validating data against specific criteria,
===
ensures that the data not only matches the value but also the expected type, avoiding unexpected validation results. - Handling NULL and Empty Values: When distinguishing between
NULL
and empty strings, or0
andfalse
,===
is crucial to avoid unexpected equivalences. - Comparing Numeric and String Values: To avoid confusion and errors when comparing numeric values stored as strings with integers,
===
ensures the types match. - Conditional Logic: In complex conditional statements, using
===
can clarify the intent of the code and reduce the risk of logical errors due to type juggling. - Unit Testing and Assertions: When writing tests or assertions,
===
helps in ensuring that the results are precisely what is expected, down to the type, making tests more reliable and less prone to false positives.
Here's a scenario where ===
would be preferred:
<?php function checkAdmin($username) { if ($username === "admin") { return true; } return false; } echo checkAdmin("admin") ? "User is admin" : "User is not admin"; // Outputs: User is admin echo checkAdmin("0") ? "User is admin" : "User is not admin"; // Outputs: User is not admin ?>
In this example, ===
ensures that only the string "admin" will pass the check, preventing potential vulnerabilities from other types being interpreted as true in a less strict comparison.
The above is the detailed content of Explain the difference between == and === in PHP.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










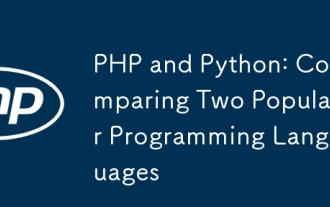
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
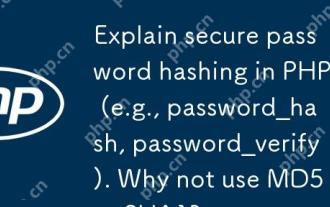
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
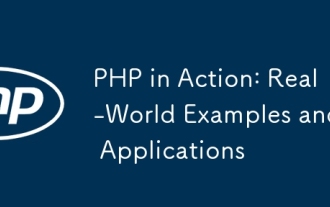
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
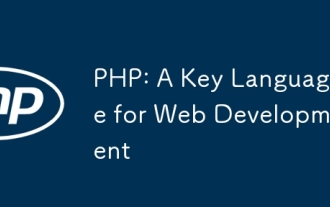
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
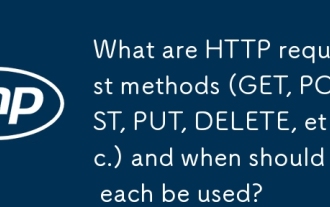
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
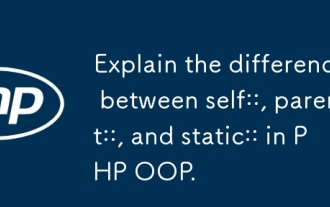
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
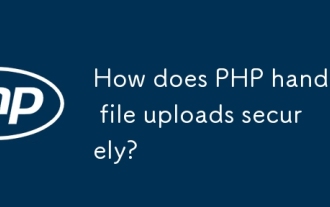
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
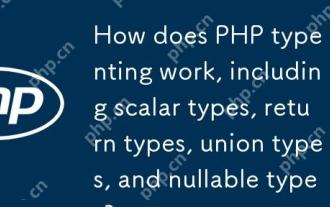
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
