Python pass Statement
Python's pass
statement is a concise and powerful tool that serves as a placeholder in your code. It allows you to create a block of code that does nothing, which is especially useful during development. Whether you are planning future features or organizing code, the pass
statement helps keep syntax correct without doing anything.
Catalog
- What is a
pass
statement? - Why use the
pass
statement? - Python
pass
Example of usage of statements - Common traps and best practices
- Conclusion
- FAQ
What is a pass
statement?
The pass
statement in Python is a unique feature that is used as a placeholder for future code. It allows developers to write syntactically correct code without implementing any functionality immediately. This is especially useful when syntactically requiring statements but no operations are required at this time.
pass
A statement is essentially an empty operation; when executed, it does not perform any operation. It is commonly used in various programming structures, including:
- Function definition: When you need to define a function but have not implemented its logic.
- Class definition: Used to create a class that will be described in detail later.
- Loop: In a control flow statement that you may want to skip certain iterations without executing any code.
-
Conditional Statement: In the
if
,elif
orelse
block, no action is required for a specific condition.
Grammar
pass
The syntax of a sentence is very simple:
pass
Why use the pass
statement?
The main reasons for using pass
statements include:
- Maintain code structure: It allows developers to create frameworks for code without having to fill in all the details immediately. This is especially useful in the initial stages of development.
-
Prevent syntax errors: Python requires that certain blocks of code (such as functions, loops, and conditional statements) contain at least one statement. Use
pass
to prevent syntax errors in these cases. -
Improving readability: By using
pass
, the developer signals to others (or himself) that the code is deliberately left blank and will be processed later. - Promote incremental development: Developers can gradually build their code base by adding features over time without worrying about breaking existing syntax rules.
- Placeholder for future logic: It reminds you that more work needs to be done and helps in planning and organization within the code.
Advantages of using pass
- Code readability: It indicates that part of the code is deliberately left blank, making it clear to anyone who reads the code at a glance.
- Syntax placeholder: It allows you to write syntactic correct code without implementing the functionality immediately.
Python pass
Example of usage of statements
Below we will see examples of different usages of the pass
statement:
Function definition
When defining a function that is planned to be implemented later, the pass
statement can be used as a placeholder. This allows you to set up the function structure without writing a full implementation right away.
pass
Here, my_function
is defined, but does nothing when called, because it only contains the pass
statement. This is very useful in the initial stages of development when you want to outline the functions without getting stuck in the details.
Class definition
Thepass
statement can also be used for class definitions, which is especially useful when you want to create a class that will be described in detail later with properties and methods.
def my_function(): pass # 未来实现的占位符
In this example, MyClass
is defined, but has no properties or methods. This allows you to build a class structure that you can extend later without causing syntax errors.
In the conditional statement
You may encounter situations where you need to check certain conditions but certain situations do not require any action. Here you can use the python pass
statement to indicate that nothing should happen under certain conditions.
class MyClass: pass # 尚未定义任何属性或方法
In this code snippet, if x is greater than 5, the program does nothing (due to the pass
statement). If x is not greater than 5, it prints a message. This structure allows for the addition of future logic without interrupting the current flow.
In the loop
In a loop, you may want to skip certain iterations based on the conditions without executing any code on those iterations. The pass
statement is used as a placeholder in this case.
pass
This loop iterates over numbers from 0 to 4. When i equals 3, the pass
statement is executed, meaning that nothing will happen during this iteration. For all other values of i, it prints the number. This structure allows you to explicitly instruct you to intentionally skip iteration without performing any action.
In exception handling
pass
statements can also be used for exception handling blocks, where you may not want to handle exceptions immediately, but still need a valid block of code.
def my_function(): pass # 未来实现的占位符
In this example, if risky_code()
raises a ValueError, the program will execute a pass
statement instead of crashing or performing anything. This allows developers to admit that they need to handle this exception later without interrupting the process of the program.
Common traps and best practices
Let's look at common pitfalls and best practices one by one:
Common Traps
-
Overuse
pass
: While it is useful as a placeholder, over-reliance on it can lead to incomplete code that may never be implemented. -
Ignore future implementations: Developers may forget to return the part marked with
pass
, resulting in the functionality or logic not being completed. -
Misuse in exception handling: Using
pass
in exception handling without any logging or processing may make debugging difficult because errors may be ignored.
Best Practice
-
Using comments: When using
pass
, consider adding comments to explain what should be implemented later. This provides context and reminder of future developments. -
Plan your code structure: Use
pass
strategically during the initial planning phase, but make sure you have plans to implement the functionality later. -
Regular review: Check your code regularly to identify the section that still contains
pass
. This helps ensure that all parts of the code are finally completed. -
Combined with TODO: Consider using
pass
and TODO annotations (e.g.,# TODO: 实现此函数
) to track what needs to be done.
Conclusion
pass
statements in Python are an important tool for developers and provide a way to maintain structure and readability while allowing future development. It is used as an effective placeholder in various programming structures, making it easier to organize ideas and plans in your code.
Main gains
-
pass
Statements do nothing, but keep the syntax correct. - It is useful in function definitions, loops, conditional statements, and class definitions.
- Use
pass
Enhance the readability of the code by indicating incomplete parts. - It allows developers to plan their code without immediate implementation.
FAQ
Q1. What happens if I don't use pass
where I need it?
A. If you omit the pass
statement where Python expects indentation blocks (such as after a function or loop definition), you will encounter an indentation error.
Q2. Can I use comments instead of pass
?
A. Although comments can indicate that some work needs to be done later, they do not meet Python's requirements for indenting code blocks. The pass
statement meets this purpose.
Q3. Is there any performance impact when using pass
?
A. No, using the python pass
statement will not have any performance impact, because it does nothing; it is just a placeholder.
Q4. Can I replace pass
with simple comments?
A. No, because the comments do not meet Python's syntax requirements for certain structures, which require executable statements.
The above is the detailed content of Python pass Statement. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










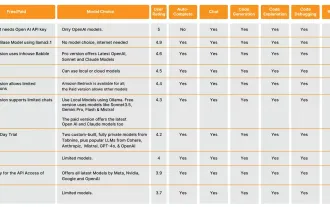
Hey there, Coding ninja! What coding-related tasks do you have planned for the day? Before you dive further into this blog, I want you to think about all your coding-related woes—better list those down. Done? – Let’
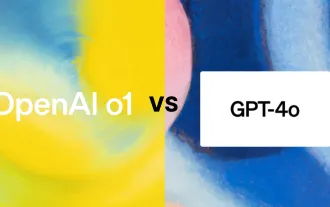
Introduction OpenAI has released its new model based on the much-anticipated “strawberry” architecture. This innovative model, known as o1, enhances reasoning capabilities, allowing it to think through problems mor

Introduction Mistral has released its very first multimodal model, namely the Pixtral-12B-2409. This model is built upon Mistral’s 12 Billion parameter, Nemo 12B. What sets this model apart? It can now take both images and tex
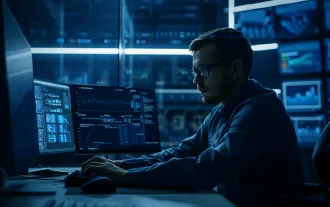
SQL's ALTER TABLE Statement: Dynamically Adding Columns to Your Database In data management, SQL's adaptability is crucial. Need to adjust your database structure on the fly? The ALTER TABLE statement is your solution. This guide details adding colu
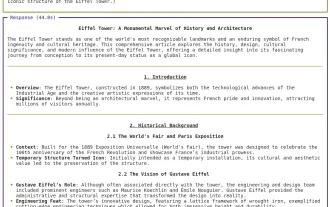
While working on Agentic AI, developers often find themselves navigating the trade-offs between speed, flexibility, and resource efficiency. I have been exploring the Agentic AI framework and came across Agno (earlier it was Phi-
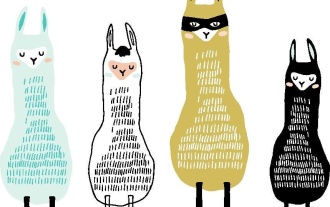
Troubled Benchmarks: A Llama Case Study In early April 2025, Meta unveiled its Llama 4 suite of models, boasting impressive performance metrics that positioned them favorably against competitors like GPT-4o and Claude 3.5 Sonnet. Central to the launc
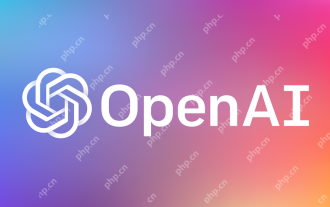
The release includes three distinct models, GPT-4.1, GPT-4.1 mini and GPT-4.1 nano, signaling a move toward task-specific optimizations within the large language model landscape. These models are not immediately replacing user-facing interfaces like
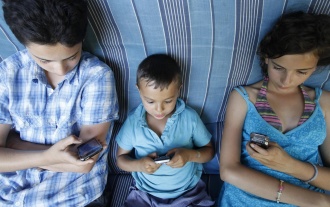
Can a video game ease anxiety, build focus, or support a child with ADHD? As healthcare challenges surge globally — especially among youth — innovators are turning to an unlikely tool: video games. Now one of the world’s largest entertainment indus
