How to configure the template of the component in Vue
Understanding export default
and Vue Component Templates
This article addresses common questions regarding the use of export default
with templates in Vue.js components. We'll explore its configuration, best practices, and impact on reusability and performance.
Vue中export default如何配置组件的template
In Vue.js, export default
is used to export the default component from a .vue
file or a JavaScript file containing a component definition. When it comes to configuring the template, you don't directly configure export default
to handle the template. Instead, export default
exports the entire component object, which includes the template. The template itself can be defined in several ways:
1. Using the <template>
tag within a .vue
single-file component: This is the most common and recommended approach. The <template>
tag neatly encapsulates your component's HTML structure.
<template> <div> <h1>My Component</h1> <p>{{ message }}</p> </div> </template> <script> export default { data() { return { message: 'Hello from the template!' } } } </script>
2. Using the template
option within the export default
object (in a .js
file): This approach is less common but works when you're defining components entirely within JavaScript files.
export default { name: 'MyComponent', template: ` <div> <h1>My Component</h1> <p>{{ message }}</p> </div> `, data() { return { message: 'Hello from the template!' } } };
In both cases, export default
simply exports the entire object containing the template
(along with data
, methods
, computed
, etc.). It doesn't directly manage the template; it's a container for the whole component definition.
How can I use export default
to effectively structure my Vue component's template for better organization?
export default
itself doesn't directly influence template organization. However, using it in conjunction with good component structuring practices leads to better organization. This includes:
-
Single-File Components (.vue): Leverage the
<template>
,<script>
, and<style>
sections within.vue
files for clear separation of concerns. This improves readability and maintainability. -
Component Composition: Break down complex templates into smaller, reusable sub-components. Import and use these sub-components within your main component's template. Each sub-component can have its own
export default
. -
Scoped Styles: Use scoped styles (e.g.,
<style scoped>
) within your.vue
files to prevent CSS conflicts between components. -
Template Syntax: Utilize features like
v-for
,v-if
, and other directives to create dynamic and well-structured templates. -
Code Splitting: For large applications, consider code splitting techniques to load only necessary components on demand. This doesn't directly involve
export default
, but it improves performance and organization.
What are the best practices for using export default
when defining the template within a Vue component?
Best practices center around maintaining clean and maintainable code:
-
Always use
export default
for single components: This makes it clear which component is the primary export of the file. -
Prefer
.vue
single-file components: They offer the best organization and maintainability for templates, scripts, and styles. - Keep templates concise and focused: Avoid overly complex templates within a single component. Break down complex logic into smaller, reusable components.
- Use consistent indentation and formatting: This improves readability and maintainability across your project.
- Use meaningful component names: Choose descriptive names that reflect the component's purpose.
- Thoroughly test your components: Ensure your templates render correctly and interact as expected with the component's logic.
Does using export default
with a template in a Vue component affect its reusability or performance?
No, using export default
itself doesn't directly impact reusability or performance. Reusability depends on how well you design and structure your component, independent of the export default
mechanism. Similarly, performance is largely affected by factors such as component size, rendering complexity, and data handling, not by the use of export default
. export default
is simply a mechanism for exporting the component, it doesn't inherently add overhead. However, poorly written components (regardless of how they're exported) will negatively affect reusability and performance.
The above is the detailed content of How to configure the template of the component in Vue. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics











Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
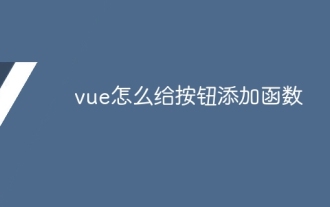
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.

Netflix mainly considers performance, scalability, development efficiency, ecosystem, technical debt and maintenance costs in framework selection. 1. Performance and scalability: Java and SpringBoot are selected to efficiently process massive data and high concurrent requests. 2. Development efficiency and ecosystem: Use React to improve front-end development efficiency and utilize its rich ecosystem. 3. Technical debt and maintenance costs: Choose Node.js to build microservices to reduce maintenance costs and technical debt.

Netflixusesacustomframeworkcalled"Gibbon"builtonReact,notReactorVuedirectly.1)TeamExperience:Choosebasedonfamiliarity.2)ProjectComplexity:Vueforsimplerprojects,Reactforcomplexones.3)CustomizationNeeds:Reactoffersmoreflexibility.4)Ecosystema
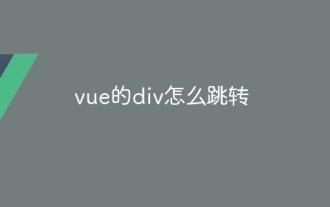
There are two ways to jump div elements in Vue: use Vue Router and add router-link component. Add the @click event listener and call this.$router.push() method to jump.

Netflix mainly uses React as the front-end framework, supplemented by Vue for specific functions. 1) React's componentization and virtual DOM improve the performance and development efficiency of Netflix applications. 2) Vue is used in Netflix's internal tools and small projects, and its flexibility and ease of use are key.
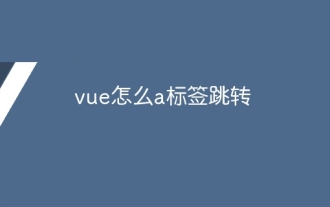
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.

Netflix uses React as its front-end framework. 1) React's componentized development model and strong ecosystem are the main reasons why Netflix chose it. 2) Through componentization, Netflix splits complex interfaces into manageable chunks such as video players, recommendation lists and user comments. 3) React's virtual DOM and component life cycle optimizes rendering efficiency and user interaction management.
