Using @error responsibly in Sass
Key Points
- The
@error
directive in Sass is a powerful tool to control author input and throw errors when problems occur, which is more effective than allowing compiler failures. - For older versions of Sass that do not support
@error
, you can use the@warn
directive instead. To ensure that the compiler still crashes when an error occurs, a hybrid macro can be created that triggers a compilation error after a warning.
The -
feature-exists('at-error')
function can be used to check if@error
is supported. If not supported, use the@warn
directive and then use a function without the@return
statement to crash the compiler. -
log
Functions can be used within other functions, andlog
hybrid macros can be used elsewhere, thus throwing errors responsibly. This allows for efficient error management for different versions of Sass.
Since Ruby Sass 3.4 and LibSass 3.1, the @error
directive can be used. This directive is similar to @warn
and is designed to terminate the execution process and display a custom message to the current output stream (probably the console).
Needless to say, this feature is very useful when building functions and mixed macros involving some Sass logic to control the author's input and throw errors when any problems arise. You have to admit that this is better than letting the compiler fail, right?
Everything is good. Except Sass 3.3 is still widely used. Sass 3.2 is even used in some places. Updating Sass is not easy, especially in large projects. Sometimes it's not feasible to spend time and budget to update something that's working properly. For these older versions, @error
is meaningless and is considered a custom at-directive
, which is completely allowed in Sass for forward compatibility reasons.
Well, does this mean that unless we decide to support only the latest Sass engine, we can't use @error
? Well, you can imagine there is a way, so with this post.
What is the idea?
The idea is simple: if you support @error
, we will use it. Otherwise, we use @warn
. Although @warn
does not prevent the compiler from continuing execution, we may want to trigger a compile error after a warning so that the compiler will crash completely. Enjoy, you don't often have to destroy something unbridled.
This means we need to wrap the entire content in a mixed macro, let's call it log(...)
. We can use it like this:
<code>@include log('哎呀,你刚才的操作出了问题!');</code>
You have to admit, it's cool, isn't it? OK, bragging enough, let's build it.
Build logger
So our hybrid macro works exactly the same way as @error
or @warn
because it's just a wrapper. Therefore, it only requires one parameter: message.
<code>@include log('哎呀,你刚才的操作出了问题!');</code>
You may ask yourself how we will check @error
Support. At first, I came up with a clumsy solution involving version sniffing, but that was awful. Furthermore, I completely overlooked the fact that Sass core designers are smart people who took the whole thing into consideration and introduced the feature-exists(...)
key to the at-error
function, returning whether the function is supported or not.
<code>@mixin log($message) { ... }</code>
If you are a patch description reader, you probably know that the feature-exists(...)
function is only introduced in Sass 3.3. It doesn't cover Sass 3.2! OK, part of it is true. In Sass 3.2, feature-exists('at-error')
is evaluated as a truth string. By adding == true
, we make sure that Sass 3.2 does not enter this condition and move to the @warn
version.
So far, everything went well. Although we have to trigger a compile error after warning. How will we do this? There are many ways to crash Sass, but ideally we want to get something you can recognize. Eric Suzanne came up with an idea before: calling functions without @return
statements is enough to crash. This mode is often called noop, i.e. no operation. Basically, this is an empty function that does nothing. Due to the way Sass works, it crashes the compiler. This is very good!
<code>@mixin log($message) { @if feature-exists('at-error') == true { @error $message; } @else { @warn $message; } }</code>
How will we call this function last point? The Sass function can only be called at a specific location. We have several methods available:
- A dummy variable, for example:
$_: noop()
- A virtual property, for example:
crash: noop()
- A empty condition, for example:
@if noop() {}
- You may find several other ways to call this function.
I want to warn you not to use $_
because it is a common variable in Sass libraries and frameworks. While it may not be a problem in Sass 3.3, in Sass 3.2, this will override any global $_
variables, which in some cases can lead to difficult-to-trace issues. Let's use the null condition because it makes sense when used with noop. A noop condition for the noop function.
<code>@function noop() {}</code>
Okay! Let's test it with the previous code:
<code>@mixin log($message) { @if feature-exists('at-error') == true { @error $message; } @else { @warn $message; // 由于函数不能在 Sass 中的任何地方调用, // 我们需要在一个虚拟条件中进行调用。 @if noop() {} } }</code>
The following is LibSass:
<code>@include log('哎呀,你刚才的操作出了问题!');</code>
The following is Sass 3.4:
<code>message: path/to/file.scss 1:1 哎呀,你刚才的操作出了问题! Details: column: 1 line: 1 file: /path/to/file.scss status: 1 messageFormatted: path/to/file.scss 1:1 哎呀,你刚才的操作出了问题!</code>
The following are Sass 3.2 and 3.3 (the output is a bold guess as I can't easily test these versions in my terminal):
<code>Error: 哎呀,你刚才的操作出了问题! 在 path/to/file.scss 的第 1 行,位于 `log` 中 使用 --trace 获取回溯。</code>
This seems to work! In any engine, even the old engine, the compiler will exit. On those engines that support @at-error
, they will receive an error message immediately. On those unsupported engines, they receive messages as warnings and then crash the compilation due to the noop function.
Enable it to record logs inside the function
The only problem with our current setup is that we cannot throw an error from inside the function because we built a hybrid macro. Mixed macros cannot be included inside a function (because it may print CSS code, which has nothing to do with the Sass function)!
What if we convert the mixed macro to a function first? At this point, something strange happened: @error
is not recognized as a valid function in Sass 3.3-, so it will fail miserably: at-directive
Functions can only contain variable declarations and control instructions.To be fair. This means we no longer need noop hacks, because unsupported engines will crash without us having to force them. Although we have to put the
directive above the process so that the message is printed to the author's console before crashing. @warn
<code>@include log('哎呀,你刚才的操作出了问题!');</code>
<code>@mixin log($message) { ... }</code>
Final Thoughts
That's it! We can now use the function inside the function (due to limitations) and we can use the log(...)
hybrid macro anywhere else to responsibly throw errors. Very neat! log(...)
Try this gist on SassMeister. (SassMeister link should be provided here)
For more advanced logging systems in Sass, I recommend you read "Build Logger Hybrid Macros". Regarding supporting older versions of Sass, I recommend you check out When and How to Support Multiple Versions of Sass.
(The FAQ section on the responsible use of errors in Sass should be included here, but I have omitted it due to space limitations.)
The above is the detailed content of Using @error responsibly in Sass. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










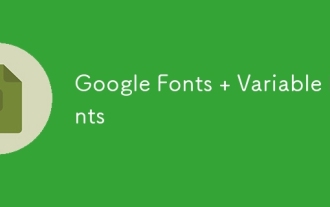
I see Google Fonts rolled out a new design (Tweet). Compared to the last big redesign, this feels much more iterative. I can barely tell the difference
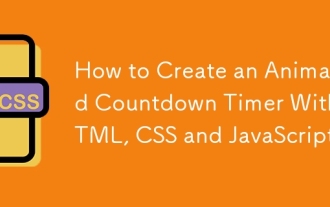
Have you ever needed a countdown timer on a project? For something like that, it might be natural to reach for a plugin, but it’s actually a lot more
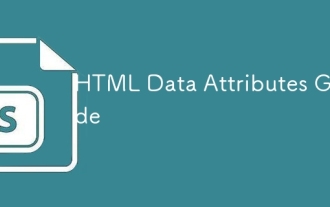
Everything you ever wanted to know about data attributes in HTML, CSS, and JavaScript.
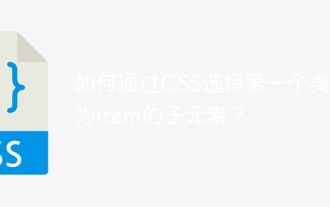
When the number of elements is not fixed, how to select the first child element of the specified class name through CSS. When processing HTML structure, you often encounter different elements...
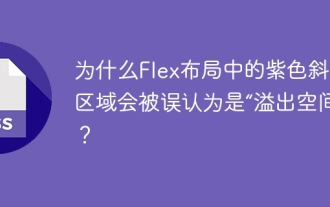
Questions about purple slash areas in Flex layouts When using Flex layouts, you may encounter some confusing phenomena, such as in the developer tools (d...
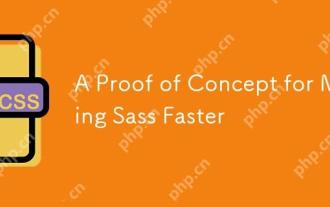
At the start of a new project, Sass compilation happens in the blink of an eye. This feels great, especially when it’s paired with Browsersync, which reloads
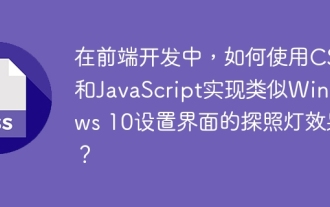
How to implement Windows-like in front-end development...
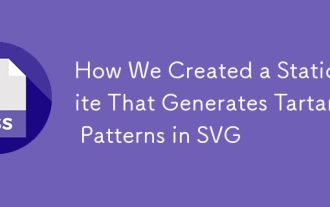
Tartan is a patterned cloth that’s typically associated with Scotland, particularly their fashionable kilts. On tartanify.com, we gathered over 5,000 tartan
