Stress-test your PHP App with ApacheBench
Stress Test Your PHP Apps: A Beginner's Guide to Apache Bench
Sponsored by New Relic. Thank you for supporting the sponsors that make SitePoint possible!
Unexpected traffic surges can cripple your application. Whether it's a viral Reddit post or a sudden spike in popularity, handling massive influxes of visitors is crucial. While cloud platforms offer automatic scaling, proactive local testing saves time and money. This guide introduces Apache Bench (ab), a powerful tool for load testing your PHP applications before deployment.
Introducing Apache Bench (ab)
Apache Bench is a command-line tool for stress-testing web servers. It simulates various load conditions, allowing you to fine-tune your application's performance under pressure. While often included with Apache installations, you can install it using your system's package manager (e.g., sudo apt-get install apache2-utils
on Ubuntu).
For this tutorial, we'll use a simple Laravel application. Ensure you have Laravel and Composer installed. Create a new Laravel project:
composer create-project laravel/laravel Laravel --prefer-dist
Configure your virtual host (e.g., Homestead) to point to the public
directory of your Laravel project. You should now be able to access your application via a URL (e.g., http://homestead.app:8000
).
Running Your First Test
A basic Apache Bench command looks like this:
ab homestead.app/
This command will likely return results too fast to be useful. To simulate a more realistic load, use the -n
(number of requests) and -c
(concurrency) options:
ab -n 500 -c 100 homestead.app/
This command sends 500 requests with 100 concurrent connections. The output shows the percentage of requests completed within specific timeframes.
Introducing Artificial Delays
Let's intentionally slow down our application to illustrate the impact of inefficient code. Modify the showWelcome
function in app/Http/Controllers/HomeController.php
:
public function showWelcome() { if (isset($_GET['slower']) && $_GET['slower'] == 'true') { sleep(1); // Introduce a 1-second delay } else { usleep(1); // Minimal delay } return view('welcome'); }
And update your route in routes/web.php
:
Route::get('/', 'HomeController@showWelcome');
Now run Apache Bench against both homestead.app
and homestead.app?slower=true
. The difference in results will highlight how long-running scripts significantly impact performance under load.
Conclusion
This tutorial demonstrated the importance of optimizing your PHP application for performance. Apache Bench is a valuable tool for identifying bottlenecks and ensuring your application can handle high traffic. Experiment with different parameters, and remember that even small optimizations can make a big difference.
Frequently Asked Questions (FAQs)
This section contains answers to common questions about using Apache Bench for stress testing PHP applications. (The original FAQs have been consolidated and slightly reworded for brevity and clarity).
Q: What is Apache Bench and why is it important?
A: Apache Bench (ab) is a command-line tool for benchmarking HTTP servers. It's crucial for stress testing because it helps you understand how your application performs under various load levels, allowing for proactive optimization.
Q: How do I install and use Apache Bench?
A: Installation depends on your system (check your system's package manager). Usage involves the ab
command followed by options (like -n
for requests and -c
for concurrency) and the target URL.
Q: How do I interpret Apache Bench results?
A: Key metrics include requests per second (higher is better), time per request (lower is better), and failed requests (should be zero). The "Time taken for tests" shows the total test duration.
Q: Can Apache Bench test HTTPS sites?
A: Yes, simply use the https
protocol in your URL.
Q: How does Apache Bench compare to other load testing tools?
A: Apache Bench is simple and quick for basic testing. More advanced tools offer features like scripting and more sophisticated scenario testing.
Q: Can Apache Bench help identify bottlenecks?
A: While it doesn't pinpoint the exact cause, it reveals performance issues (low requests per second, high failed requests) that require further investigation using debugging and profiling tools.
The above is the detailed content of Stress-test your PHP App with ApacheBench. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
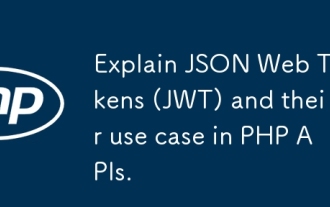
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
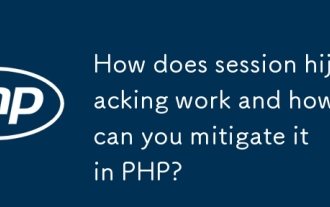
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.

The enumeration function in PHP8.1 enhances the clarity and type safety of the code by defining named constants. 1) Enumerations can be integers, strings or objects, improving code readability and type safety. 2) Enumeration is based on class and supports object-oriented features such as traversal and reflection. 3) Enumeration can be used for comparison and assignment to ensure type safety. 4) Enumeration supports adding methods to implement complex logic. 5) Strict type checking and error handling can avoid common errors. 6) Enumeration reduces magic value and improves maintainability, but pay attention to performance optimization.
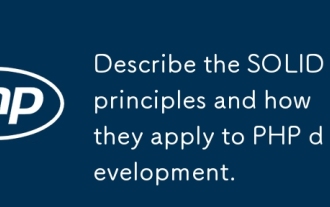
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
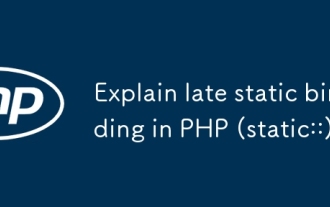
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
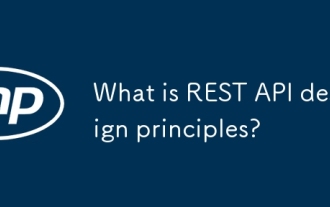
RESTAPI design principles include resource definition, URI design, HTTP method usage, status code usage, version control, and HATEOAS. 1. Resources should be represented by nouns and maintained at a hierarchy. 2. HTTP methods should conform to their semantics, such as GET is used to obtain resources. 3. The status code should be used correctly, such as 404 means that the resource does not exist. 4. Version control can be implemented through URI or header. 5. HATEOAS boots client operations through links in response.
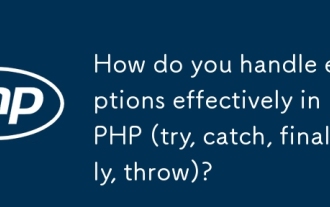
In PHP, exception handling is achieved through the try, catch, finally, and throw keywords. 1) The try block surrounds the code that may throw exceptions; 2) The catch block handles exceptions; 3) Finally block ensures that the code is always executed; 4) throw is used to manually throw exceptions. These mechanisms help improve the robustness and maintainability of your code.
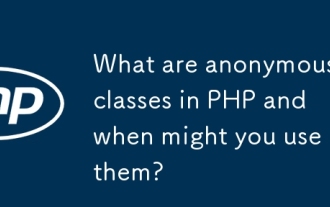
The main function of anonymous classes in PHP is to create one-time objects. 1. Anonymous classes allow classes without names to be directly defined in the code, which is suitable for temporary requirements. 2. They can inherit classes or implement interfaces to increase flexibility. 3. Pay attention to performance and code readability when using it, and avoid repeatedly defining the same anonymous classes.
