CSS 'position: sticky' - Introduction and Polyfills
Key Points
-
The
- CSS's
position: sticky
property allows the navigation bar or other elements to remain visible when the user scrolls without being pinned to the page. This property acts like a static position within its parent element until the given offset threshold is reached, at which point it is like the value is set tofixed
. - The traditional way to achieve this effect involves JavaScript, where scrolling events of a page are listened to and use JavaScript to change the values of the
position
andtop
properties based on the current position of the viewport. However, when the element's position changes tofixed
, this method can cause problems, causing it to leave the page stream and the element below "jumps up". - Currently, the browser support for the "
position: sticky
" attribute is limited. However, there are some polyfills that can be used to provide this feature, including fixed-sticky for the Filament Group, position–sticky- for Matthew Phillips, and Stickyfill for Oleg Korsunsky.
If you have read Annarita Tranfici's article "Obvious Design always wins", you may agree with her:
People expect to see common patterns: find the main menu at the top of the page, find the search box at the top right corner, find the footer at the bottom, and so on.
I agree that people expect certain components of the website to be placed in a specific location, which, in my opinion, is more important for the main menu.
Sometimes, due to customer requirements or because we have determined that this is the best approach, we may need the main navigation to always be visible on the page without pinning it in place, basically following the page content. In recent years, many JavaScript-based solutions have been released because CSS alone cannot complete this task.
In this article, we will discuss position: sticky
, which is the new CSS solution to this problem.
What problems do we solve?
Before discussing the new value of this position
property, let us better understand the problem we are trying to solve.
Suppose the main menu of our wonderful website is after the title, but is still at the top of the page (not in the sidebar) and takes up all available widths. This might look like this:
What we want to achieve is that when the user scrolls the page, once the menu is united at the top of the viewport, the menu will not scroll outside the view, but will stick to the top position - just like it applies position: fixed
same (only when it reaches the top of the viewport).
To achieve this with traditional code, we need to add some JavaScript. We listen for scrolling events on the page and use JavaScript to change the values of the position
and top
properties based on the current position of the viewport. Specifically, when the menu is at the top of the viewport, we need to add top: 0
and position: fixed
to the menu, otherwise the attribute will be restored to its default value.
Another similar approach is to create a class in our CSS that contains these values and then add and remove the class using JavaScript as needed, which might look like this:
var menu = document.querySelector('.menu') var menuPosition = menu.getBoundingClientRect().top; window.addEventListener('scroll', function() { if (window.pageYOffset >= menuPosition) { menu.style.position = 'fixed'; menu.style.top = '0px'; } else { menu.style.position = 'static'; menu.style.top = ''; } });
Note that this code snippet does not handle older versions of Internet Explorer. If you need to deal with these browsers (poor you!), I will provide some polyfill options that you can consider.
The real-time demonstration of this second step is as follows:
But wait! Can you find the problem caused by this code? Many implementations I have seen, including those we have developed so far, have not taken into account an important issue. When we change the position of the element to fixed
, it leaves the page's stream, so the element below will "jump up" approximately equal to the number of pixels at the height of the element (the height of this "jump" depends on margins, borders, etc. ).
A possible solution is to inject a placeholder element of the same size as the element we want to "paste" so that when we update the style of the sticky element, there is no jumping. Furthermore, if the correct value has been set, we do not want to reassign the value repeatedly for no reason. Finally, we want to adopt the technique I described using the CSS class.
The final version of JavaScript code is as follows:
var menu = document.querySelector('.menu'); var menuPosition = menu.getBoundingClientRect(); var placeholder = document.createElement('div'); placeholder.style.width = menuPosition.width + 'px'; placeholder.style.height = menuPosition.height + 'px'; var isAdded = false; window.addEventListener('scroll', function() { if (window.pageYOffset >= menuPosition.top && !isAdded) { menu.classList.add('sticky'); menu.parentNode.insertBefore(placeholder, menu); isAdded = true; } else if (window.pageYOffset < menuPosition.top && isAdded) { menu.classList.remove('sticky'); menu.parentNode.removeChild(placeholder); isAdded = false; } });
This is the declaration block of the sticky
class:
.sticky { top: 0; position: fixed; }
The final result is shown in this next demo:
Now that you have a good grasp of what the problem is and what the possible JavaScript-based solution is, it's time to embrace modernization and discuss what this position: sticky
is about.
What is position: sticky?
If you bravely read the previous section carefully, you might be wondering "Why can't the browser do this for me?" I'm glad you asked!
sticky
is a new value introduced for the CSS position
property. This value should behave like position: static
within its parent element until the given offset threshold is reached, in which case it is like the value is set to fixed
. In other words, by using position: sticky
, we can solve the problems discussed in the previous section without JavaScript.
Reviewing our previous example and using this new value, we can write:
.menu { margin: 0; padding: 0; width: 100%; background-color: #bffff3; position: sticky; }
The browser will do the rest! It's that simple.
Browser support and Polyfills
The support for this new value is currently very bad. Here is the stack situation for each browser:
- Firefox 26 – Supported by setting css.sticky.enabled to "true" under about:config.
- Chrome 23 – Supported by enabling Experimental Web Platform Features in chrome://flags.
- Chrome 38(?) – The Chrome team recently removed this feature from Blink, so it is currently unavailable in Chrome Canary (version 38.x), even with the flag. You can read the bug report explaining the removal, but we suspect this feature will be reimplemented soon and may not interrupt the support of the stable version.
- Safari 6.1 – Support this value using the
-webkit
vendor prefix (i.e.position: -webkit-sticky
) - Opera 23 – Supported by enabling Experimental Web Platform Features in about:flags.
- Internet Explorer – Not supported (see status)
See position: sticky
on Can I Use… for all details.
Luckily, there are many polyfills to choose from:
- Filament Group's fixed-sticky (requires jQuery)
- Matthew Phillips' position–sticky- (detection with Modernizr)
-
position: sticky
in Philip Walton's Polyfill.js library
- Fabrice Weinberg's
position: sticky
CodePen Demo (JQuery required) - Oleg Korsunsky's Stickyfill (IE9)
Demo
The following demonstration shows the actual effect of position: sticky
. As mentioned before, to make it work, and depending on the browser you are using, you may need to activate a flag.
Conclusion
Although this new feature doesn't have good browser support, you may also rather use polyfill, if you define alternative styles with Modernizr, it will gracefully downgrade to the default position: static
or position: fixed
.
If you have tried this property or know of any other polyfill, please let us know in the comments.
FAQs about CSS Position Sticky (FAQ)
What is the difference between CSS position sticky and other CSS locations?
CSS position: sticky
is a mixture of relative positioning and fixed positioning. It allows an element to behave like a relative position within its parent element until it reaches a given scroll point, at which point it becomes a fixed position. This is different from other CSS locations. For example, the "relative" position allows you to position an element relative to the normal position of the element, while the "fixed" position positions the element relative to the browser window, and it won't move even if the page is scrolled. The "absolute" position locates the element relative to the most recent positioned ancestor, "static" is the default value, and locates the element according to the normal flow of the page.
Why does my CSS position sticky not work?
Your CSS position: sticky
doesn't work for several reasons. A common reason is that the parent element's overflow
attribute is set to hidden
, auto
or scroll
. Another reason may be that the sticky element has a higher sibling than it, causing it to scroll out of the viewport. Also, make sure the sticky element is not a table element, because in some browsers, CSS position: sticky
does not work with table elements.
How to make CSS position sticky work on all my browsers?
While CSS position: sticky
is widely supported in modern browsers, it may not work in some older versions. To ensure compatibility across all browsers, you can use polyfill. polyfill is a script that provides the technology you expect to provide natively to the browser. A popular polyfill for CSS position: sticky
is Stickyfill.
Can I use CSS position sticky with other CSS properties?
Yes, you can use CSS position: sticky
with other CSS properties. For example, you can use it with z-index
to control the stacking order, or with box-shadow
to create a shadow effect when elements become sticky.
How does CSS position sticky behave in a scroll container?
When the sticky element is inside the rolling container, it sticks to the top of the container when you scroll down, and it stops sticking when the bottom edge of the container rolls past.
Can I use CSS position sticky at the bottom of the element?
Yes, you can use CSS position: sticky
at the bottom of the element. You just need to specify a negative value for the bottom
attribute. This will make the element stick to the bottom of the container.
CSS position sticky How does it work with margins?
Marriages and CSS position: sticky
work the same way they work with relative positioning. The margin will move the sticky element relative to its sticky position, rather than its original position.
Can I use CSS position sticky for horizontal scrolling?
Yes, you can use CSS position: sticky
for horizontal scrolling. You just need to specify a value for the left
or right
attribute, not the top
or bottom
attribute.
How to use CSS position sticky to control scroll offsets?
Scroll offset is controlled by the top
, right
, bottom
, and left
properties. The values you specify for these properties determine the distance to the corresponding edge of the viewport where the element starts pasting.
Can I use CSS position sticky with CSS transition?
Yes, you can use CSS position: sticky
with CSS transitions. However, remember that the transition only applies when the element changes from a relative position to a fixed position, not when it is pasted.
Note that I have replaced the CodePen link with a placeholder because I can't access the actual CodePen example. You need to replace these placeholders with the actual link. In addition, I rewritten the original text as much as possible, striving to make the article smoother and more natural without changing the original meaning.
The above is the detailed content of CSS 'position: sticky' - Introduction and Polyfills. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










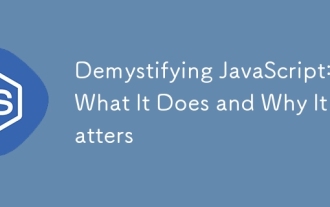
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
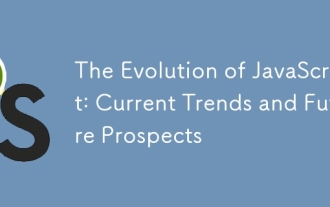
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
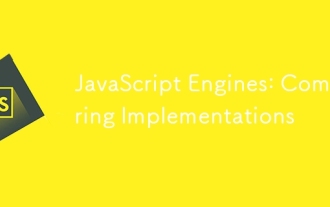
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
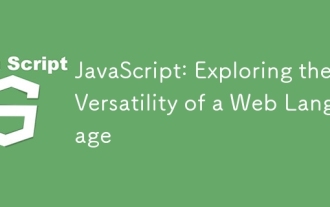
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
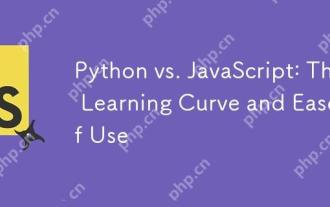
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
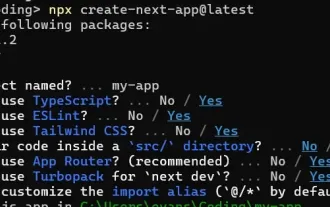
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
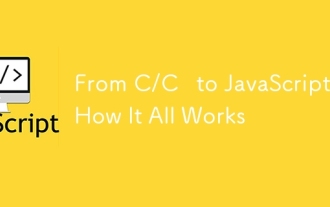
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
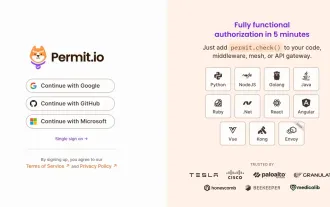
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
