Laravel Quick Tip: Model Route Binding
Laravel routing component: Simplify and efficient routing management
This article discusses Laravel's powerful routing component, which provides simple and efficient routing management methods, and supports simple URLs, parameters, groups, naming, and event protection of routing groups. Its routing model binding function simplifies the processing of repetitive tasks by prompting the model name rather than ID parameters.
Core points:
- Laravel's routing component provides simple and efficient routing management methods, supporting simple URLs, parameters, groups, naming, and event protection routing groups. The routing model binding function simplifies repetitive task processing by prompting the model name rather than the ID parameters.
- Laravel's routing model binding will automatically parse the model using ID parameters, and throw an exception if the model does not exist. The
App\Exceptions\Handler@render
method is responsible for converting the exception to an HTTP response and can be used to processModelNotFoundException
and redirect to a 404 page. - Laravel allows custom routing model binding, such as rewriting the
getRouteKeyName
method of the parent model class to use different attribute names, such as UUID. This helps avoid exposing internal IDs to the end user.
Example: Manage backend category
Suppose there are a series of categories in the database that administrators can manage in the background. The routing file is as follows:
Route::group(['namespace' => 'Admin', 'prefix' => 'admin', 'middleware' => 'admin'], function () { Route::resource('categories', 'CategoriesController'); });
CategoriesController
class contains seven resource methods. In the edit
operation, you need to check whether the category to be edited exists in the database, otherwise an error message will be returned and redirected:
public function edit($id) { $category = Category::find($id); if (!$category) { return redirect()->route('admin.categories.index')->withErrors([trans('errors.category_not_found')]); } // ... }
Routing model binding
This is a common practice, but Laravel provides a more optimized way - routing model binding. Just type prompts the model name, no ID parameters are required.
The available route list is as follows:
<code>+--------+-----------+------------------------------------+------------------------------------+----------------------------------------------------------------------+-----------------+ | Domain | Method | URI | Name | Action | Middleware | +--------+-----------+------------------------------------+------------------------------------+----------------------------------------------------------------------+-----------------+ | | GET|HEAD | admin/categories | admin.categories.index | App\Http\Controllers\Admin\CategoriesController@index | web,admin | | | POST | admin/categories | admin.categories.store | App\Http\Controllers\Admin\CategoriesController@store | web,admin | | | GET|HEAD | admin/categories/create | admin.categories.create | App\Http\Controllers\Admin\CategoriesController@create | web,admin | | | GET|HEAD | admin/categories/{categories} | admin.categories.show | App\Http\Controllers\Admin\CategoriesController@show | web,admin | | | PUT|PATCH | admin/categories/{categories} | admin.categories.update | App\Http\Controllers\Admin\CategoriesController@update | web,admin | | | DELETE | admin/categories/{categories} | admin.categories.destroy | App\Http\Controllers\Admin\CategoriesController@destroy | web,admin | | | GET|HEAD | admin/categories/{categories}/edit | admin.categories.edit | App\Http\Controllers\Admin\CategoriesController@edit | web,admin |</code>
Routing parameters are {categories}
, which can be modified as needed. Laravel provides an option to modify it:
Route::resource('categories', 'CategoriesController', [ 'parameters' => 'singular', ]);
Modified route:
<code>+--------+-----------+------------------------------------+------------------------------------+----------------------------------------------------------------------+-----------------+ | Domain | Method | URI | Name | Action | Middleware | +--------+-----------+------------------------------------+------------------------------------+----------------------------------------------------------------------+-----------------+ | | GET|HEAD | admin/categories | admin.categories.index | App\Http\Controllers\Admin\CategoriesController@index | web,admin | | | POST | admin/categories | admin.categories.store | App\Http\Controllers\Admin\CategoriesController@store | web,admin | | | GET|HEAD | admin/categories/create | admin.categories.create | App\Http\Controllers\Admin\CategoriesController@create | web,admin | | | GET|HEAD | admin/categories/{category} | admin.categories.show | App\Http\Controllers\Admin\CategoriesController@show | web,admin | | | PUT|PATCH | admin/categories/{category} | admin.categories.update | App\Http\Controllers\Admin\CategoriesController@update | web,admin | | | DELETE | admin/categories/{category} | admin.categories.destroy | App\Http\Controllers\Admin\CategoriesController@destroy | web,admin | | | GET|HEAD | admin/categories/{category}/edit | admin.categories.edit | App\Http\Controllers\Admin\CategoriesController@edit | web,admin |</code>
Note: Laravel 5.3 uses the singular form by default.
public function edit(Category $category) { return view('admin.categories.edit', [ 'category' => $category ]); }
Laravel will now automatically parse the category with the ID parameter, throwing an exception if the model does not exist.
Note: Unless the parameter has a default value, it uses the findOrFail
Eloquent method to parse the parameters.
Exception handling
TheApp\Exceptions\Handler@render
method is responsible for converting exceptions into HTTP responses. We will use it to handle ModelNotFoundException
and redirect to the 404 page.
This method has the request
and exception
parameters, which can be used to determine the action to perform.
Route::group(['namespace' => 'Admin', 'prefix' => 'admin', 'middleware' => 'admin'], function () { Route::resource('categories', 'CategoriesController'); });
We test whether the thrown exception is an instance of ModelNotFoundException
. We can also test the model name to display the correct error message. To avoid adding multiple if
tests to all models, we can create an array of indexed messages and use the model class name to extract the correct messages.
Parameter analysis
Laravel uses name and type hints to resolve routing parameters. If the parameter type is a model, it tries to look up records in the database using ID, and fails if the record cannot be found.
Custom routing key
To avoid exposing internal IDs to end users, UUIDs are usually used. But since Laravel uses table primary keys to parse bound parameters, an error is always thrown!
To do this, Laravel allows us to rewrite the getRouteKeyName
method of the parent model class. This method should return the property name, in this case, UUID.
public function edit($id) { $category = Category::find($id); if (!$category) { return redirect()->route('admin.categories.index')->withErrors([trans('errors.category_not_found')]); } // ... }
Now if we try to edit a specific category with UUID, it should work as expected, for example: https://www.php.cn/link/604541b9b9f266538ed001ea49fcc956.
FAQs on Laravel routing model binding (This part of the content has been answered in detail in the original text and will not be described here)
I hope the above content will be helpful to you!
The above is the detailed content of Laravel Quick Tip: Model Route Binding. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
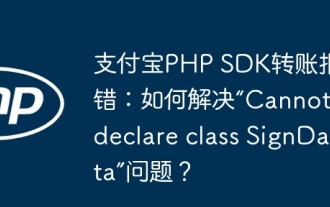
Alipay PHP...
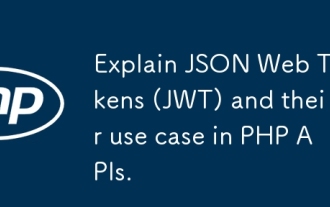
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
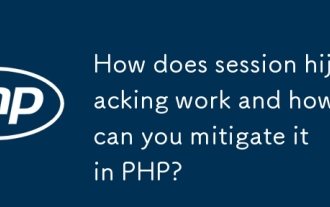
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.

The enumeration function in PHP8.1 enhances the clarity and type safety of the code by defining named constants. 1) Enumerations can be integers, strings or objects, improving code readability and type safety. 2) Enumeration is based on class and supports object-oriented features such as traversal and reflection. 3) Enumeration can be used for comparison and assignment to ensure type safety. 4) Enumeration supports adding methods to implement complex logic. 5) Strict type checking and error handling can avoid common errors. 6) Enumeration reduces magic value and improves maintainability, but pay attention to performance optimization.
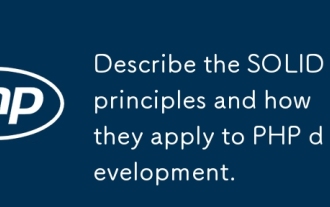
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
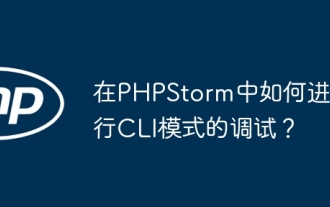
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
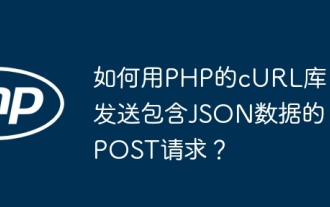
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
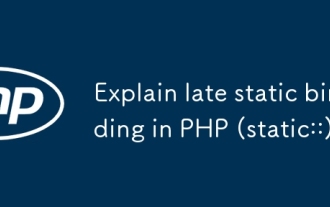
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
