Understanding Reacts useEffect and Event Listeners: A Deep Dive
Have you ever wondered how React components can maintain active event listeners without re-registering them on every render? Let's unravel this mystery by examining a common use case: tracking mouse coordinates.
The Puzzle
Consider this React component that tracks mouse position:
import React from 'react'; function MouseCoords() { const [mousePosition, setMousePosition] = React.useState({ x: 0, y: 0, }); React.useEffect(() => { function handleMouseMove(event) { setMousePosition({ x: event.clientX, y: event.clientY, }); } window.addEventListener('mousemove', handleMouseMove); }, []); return ( <div className="wrapper"> <p> {mousePosition.x} / {mousePosition.y} </p> </div> ); } export default MouseCoords;
Here's the interesting part: the empty dependency array ([]) means our useEffect only runs once, yet the component still updates when we move our mouse. How does this work? ?
The Browser's Event System vs React's Rendering
To understand this behavior, we need to recognize that two separate systems are at play:
- The Browser's Event System: Manages event listeners and triggers callbacks
- React's Rendering System: Handles component updates and UI rendering
Think of it Like a Security Camera
Imagine setting up a security camera in your home:
- The installation (useEffect with []) happens once
- The camera (event listener) stays active independently
- When movement occurs, it triggers the alarm (state update)
- You don't need to reinstall the camera every time it detects movement!
Breaking Down the Flow
Let's examine what happens step by step:
1. Initial Setup (Mount Phase)
React.useEffect(() => { // Effect runs once on mount function handleMouseMove(event) { setMousePosition({ x: event.clientX, y: event.clientY, }); } window.addEventListener('mousemove', handleMouseMove); }, []); // Empty array = run once
2. Event Handling
When the mouse moves:
- Browser's event system detects movement
- Calls our registered handleMouseMove function
- Function updates React state with setMousePosition
- Component re-renders with new coordinates
3. Cleanup (Important!)
We should always clean up our event listeners when the component unmounts. Here's the complete code:
React.useEffect(() => { function handleMouseMove(event) { setMousePosition({ x: event.clientX, y: event.clientY, }); } window.addEventListener('mousemove', handleMouseMove); // Cleanup function return () => { window.removeEventListener('mousemove', handleMouseMove); }; }, []);
Common Pitfalls to Avoid
- Missing Cleanup: Always remove event listeners to prevent memory leaks
- Unnecessary Dependencies: Don't add dependencies unless the event listener needs them
- Re-registration: Avoid putting event listeners in the render body
Real-World Example: Enhanced Mouse Tracker
Here's a more practical version with additional features:
import React from 'react'; function MouseCoords() { const [mousePosition, setMousePosition] = React.useState({ x: 0, y: 0, }); React.useEffect(() => { function handleMouseMove(event) { setMousePosition({ x: event.clientX, y: event.clientY, }); } window.addEventListener('mousemove', handleMouseMove); }, []); return ( <div className="wrapper"> <p> {mousePosition.x} / {mousePosition.y} </p> </div> ); } export default MouseCoords;
Key Takeaways
- The event listener registration (useEffect) and event handling are separate systems
- Empty dependency array ([]) means "run once on mount"
- Browser events operate independently of React's rendering cycle
- Always clean up listeners on unmount
Conclusion
Understanding the relationship between React's useEffect and browser events is crucial for building performant React applications. By leveraging the browser's event system correctly, we can create responsive interfaces without unnecessary re-renders or event listener registrations.
Remember: the event listener is like our faithful security camera - install it once, and let it do its job!
Did this explanation help you understand useEffect and event listeners better? Leave a comment below with your thoughts or questions!
The above is the detailed content of Understanding Reacts useEffect and Event Listeners: A Deep Dive. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
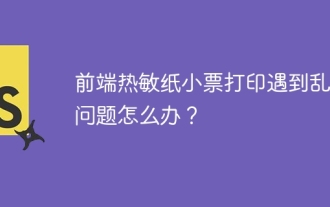
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
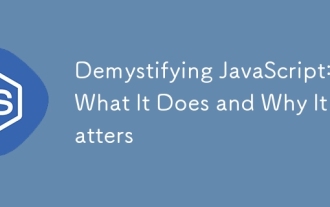
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
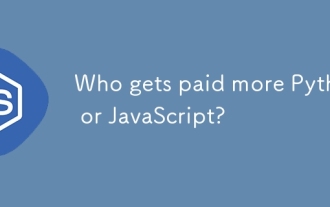
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
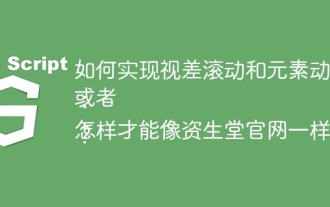
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
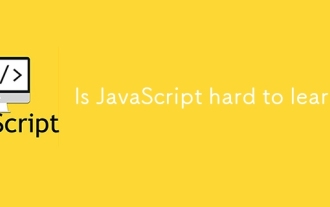
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
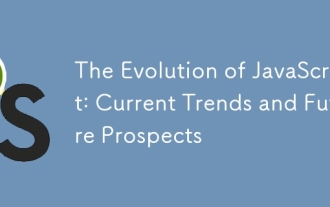
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
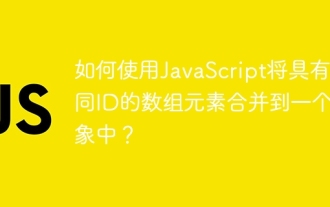
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
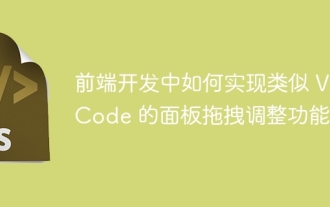
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
