JavaScript Fundamentals for Vue Developers
Start at the Basics
With the advent of AI and some tech-ed influencers, there seem to be alot of skipping of the essentials before using a framework in Javascript land. Understanding core JavaScript concepts is crucial, its like learning to walk before running. When I got this new job and had to get decent at understanding Vue, I took time to review these JavaScript to have an effective approach to Vue 3 development, I understand aand can use React ... but it isn't NOT my favorite framework, this is another discussion. Here's why these fundamentals matter :
Variables and Data Types
- Why it matters: Vue 3's reactivity system relies heavily on proper variable declarations.
- The composition API requires an understanding of const for refs and reactive objects.
- Type awareness helps with Vue3's template rendering and prop validation.
const count = ref(0) const user = reactive({ name: 'John', age: 30 })
Template Literals
- Why it matters: This is essential for Vue3 template expressions and string interpolation.
- It is extensively used in computed properties and methods.
- Template literals can be helpful for dynamic component templates and prop values.
const greeting = computed(() => `Hello, ${user.name}!`)
Arrow Functions
- Why it matters: Critical for Vue 3's Composition API.
- Used in setup() functions, computed properties, and watchers.
- Essential for maintaining correct this binding in methods.
const doubleCount = computed(() => count.value * 2) watch(() => user.name, (newValue, oldValue) => { console.log(`Name changed from ${oldValue} to ${newValue}`) })
Objects and Object Destructuring
- Why it matters: Fundamental for working with Vue's reactive objects.
- Required for component props and emits declarations.
- Essential for destructuring from setup() returns.
export default { setup(props, { emit }) { const { title, description } = props return { title, description } } }
Arrays and Array Methods
- Why it matters: Critical for rendering lists with v-for.
- Essential for reactive data manipulation.
- Used in computed properties for data transformation.
<template> <ul> <li v-for="item in filteredItems" :key="item.id">{{ item.name }}</li> </ul> </template> <script setup> const items = ref([/* ... */]) const filteredItems = computed(() => items.value.filter(item => item.isActive) ) </script>
Promises and Async/Await
- Why it matters: Crucial for data fetching in setup().
- Required for async component operations.
- Essential for lifecycle hooks and watchers.
import { onMounted } from 'vue' export default { async setup() { const data = ref(null) onMounted(async () => { data.value = await fetchData() }) return { data } } }
Modules and Exports
- Why it matters: Fundamental for component organization.
- Required for composables and plugins.
- Essential for maintaining clean architecture.
// useCounter.js import { ref } from 'vue' export function useCounter() { const count = ref(0) const increment = () => count.value++ return { count, increment } } // Component.vue import { useCounter } from './useCounter' export default { setup() { const { count, increment } = useCounter() return { count, increment } } }
Classes and Object-Oriented Concepts
- Why it matters: Helpful for understanding component inheritance.
- Used in custom directive implementations.
- Valuable for complex state management.
class BaseComponent { constructor(name) { this.name = name } sayHello() { console.log(`Hello from ${this.name}`) } } class SpecialComponent extends BaseComponent { constructor(name, special) { super(name) this.special = special } }
Optional Chaining
- Why it matters: Essential for safe property access in templates.
- Useful in computed properties.
- Helpful for handling async data states.
<template> <div>{{ user?.profile?.name }}</div> </template> <script setup> const user = ref(null) const userName = computed(() => user.value?.profile?.name ?? 'Guest') </script>
Event Handling
- Why it matters:Critical for component communication.
- Required for DOM event management.
- Essential for custom event implementations.
<template> <button @click="handleClick">Click me</button> </template> <script setup> import { defineEmits } from 'vue' const emit = defineEmits(['custom-event']) function handleClick() { emit('custom-event', { data: 'Some data' }) } </script>
Error Handling
- Why it matters:Important for component error boundaries.
- Critical for API calls and async operations.
- Essential for maintaining app stability.
const count = ref(0) const user = reactive({ name: 'John', age: 30 })
These code snippets demonstrate practical applications of each concept within the context of Vue 3 development, providing concrete examples for developers to understand and apply these fundamental JavaScript skills.
Practical Applications of Core JavaScript Concepts
To illustrate how these essential JavaScript concepts are used in widely used beginner scenarios, let's explore three mini-projects: a weather app, a background color changer, and a todo app. These examples will demonstrate the practical application of the concepts we've discussed.
Weather App
const greeting = computed(() => `Hello, ${user.name}!`)
Core Concepts Implemented:
- Async/Await: For handling asynchronous API calls.
- Fetch API: To retrieve weather data from an external service.
- DOM Manipulation: To update the HTML content dynamically.
- Template Literals: For easy string interpolation and multi-line strings.
- Error Handling: Using try/catch to manage potential errors during the fetch operation.
Background Color Changer
const doubleCount = computed(() => count.value * 2) watch(() => user.name, (newValue, oldValue) => { console.log(`Name changed from ${oldValue} to ${newValue}`) })
Core Concepts Implemented:
- Arrow Functions: For concise function expressions.
- Math Object: To generate random RGB values for colors.
- Template Literals: For constructing the RGB string.
- Event Listeners: To handle user interactions (button clicks).
- DOM Manipulation: To change the background color and display the current color.
Todo App
export default { setup(props, { emit }) { const { title, description } = props return { title, description } } }
Core Concepts Implemented:
- Local Storage: For persisting todos across sessions.
- Array Methods: Using map for rendering and push/splice for modifying the todo list.
- Arrow Functions: For concise syntax in functions.
- Event Handling: To manage form submissions and button clicks.
- Template Literals: For generating HTML markup dynamically.
These mini-projects illustrate how core JavaScript concepts come together in practical applications. They showcase asynchronous programming, DOM manipulation, event handling, array methods, and more, providing a tangible context for understanding the above essential fundamental JavaScript skills before getting into Vue3.js development.
<template> <ul> <li v-for="item in filteredItems" :key="item.id">{{ item.name }}</li> </ul> </template> <script setup> const items = ref([/* ... */]) const filteredItems = computed(() => items.value.filter(item => item.isActive) ) </script>
The above is the detailed content of JavaScript Fundamentals for Vue Developers. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










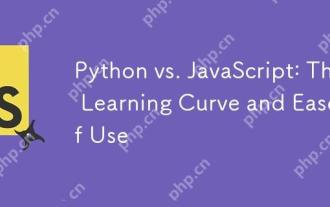
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
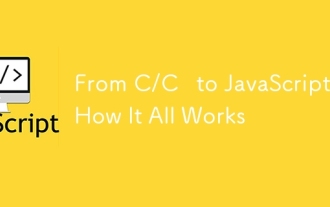
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
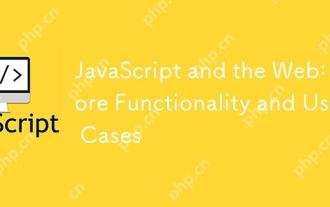
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
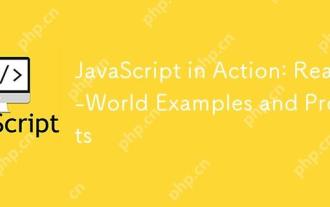
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
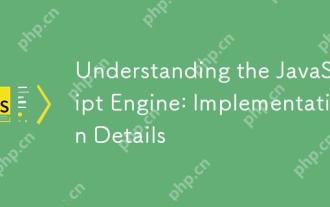
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.
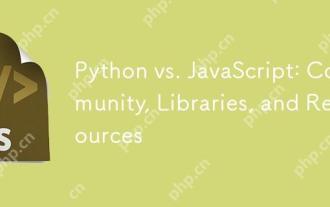
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
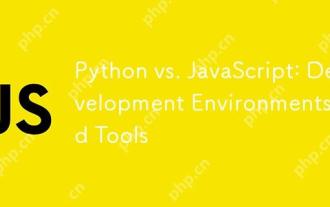
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
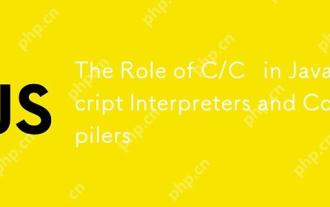
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
