CS- Week 4
Pixels
Pixels are the smallest colored dots that make up an image.
If we imagine the image as consisting of zeros and ones, the zeros represent black and the ones represent white:
RGB (Red, Green, Blue) are numbers representing the amount of each color. In Adobe Photoshop we see these settings:
We can see in the image above how the selected amount of red, green and blue changes the color. We can also see in this image that color is not represented by only three values, but by a value made up of special numbers and symbols. For example, the value 255 is represented as FF.
Hexadecimal
Hexadecimal number system is a number system based on writing numbers using only 16 characters. They are as follows:
0 1 2 3 4 5 6 7 8 9 A B C D E F
In the hexadecimal number system, each column represents 16 levels.
0 – 00 as
1 – 01 as
9 – as 09
10 – as 0A
15 – as 0F
16 – 10 as
255 is expressed as FF because 16 x 15 (or F) equals 240 plus 15 to make 255. This is the largest two-digit number that can be represented in hexadecimal.
The hexadecimal number system allows to express data in a shorter form. Therefore, it is convenient to express information more compactly.
Memory
If we number memory blocks using the hexadecimal number system, we can imagine them as follows:
It can be difficult to determine whether the block 10 in the figure represents a memory location or the value 10. Therefore, all hexadecimal numbers are usually represented by the prefix 0x:
We assign the value 50 to the integer variable n:
#include <stdio.h> int main(void) { int n = 50; printf("%i\n", n); }
How the program stores this value in memory can be visualized as follows:
The C language has the following memory manipulation operators:
- & – Gives the address of a value in memory.
- * – Tells the compiler to go to memory location.
If we want to know the memory address of our n learner, we can change our above code as follows:
0 1 2 3 4 5 6 7 8 9 A B C D E F
%p – lets you see the address of a memory location. And &n returns the address of variable n in memory starting with 0x when we run the code.
Pointers
Pointer is a variable that stores the address of this value in computer memory.
#include <stdio.h> int main(void) { int n = 50; printf("%i\n", n); }
Where p is a pointer that contains the address of an integer n.
Pointers are usually stored as 8-byte values. p is storing the address of the value 50 in the above image.
We can think of a pointer as an arrow pointing from one location in memory to another:
String
String is simply an array of characters. For example, the string s = "HI!" can be represented in computer memory as:
s tells the compiler where the first byte of the given value is located:
string variable as follows:
#include <stdio.h> int main(void) { int n = 50; printf("%p\n", &n); }
The code above prints an array of characters starting at position s.
String Comparison
We compare variable values ofstring data type with each other:
int n = 50; int *p = &n;
In our code above, we give our variables s and t the same "Hi!" even if we give the value "Different" message appears on the screen as a result.
To find out why this happens, we can give the same value to our variables s and t and represent them in computer memory as follows:
So the above code is actually trying to compare the memory locations of the variables s and t, not their values.
Copying
Let the following code be given:
#include <stdio.h> int main(void) { char *s = "HI!"; printf("%s\n", s); }
string t = s, the address of s is copied to t. This does not produce the result we want because the value is not copied - only its address is copied.
s and t are pointing to the same memory blocks. We couldn't copy its value from s to t, instead they became two pointers pointing to a single string.
malloc - allows the programmer to allocate a block of memory of a certain size.
free – asks the computer to free the previously allocated memory block.
We change the code to create a real copy:
0 1 2 3 4 5 6 7 8 9 A B C D E F
malloc(strlen(s) 1) - Adds one to the length of the variable s and allocates space for its character. Then, through the for loop, the values of s are copied to t.
Garbage Values
If we ask the compiler for a block of memory, there is no guarantee that this memory will be free. The allocated memory may have been used by the computer before, so there is a chance that there are unwanted values:
#include <stdio.h> int main(void) { int n = 50; printf("%i\n", n); }
When we run this code, 1024 memory locations are allocated for the array, but when we display the values of the array elements using a for loop, we see that not all of them are 0.
Whenever we ask the computer to allocate memory for a variable, it is good practice to initialize it to 0 or some other value.
This article uses CS50x 2024 source.
The above is the detailed content of CS- Week 4. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










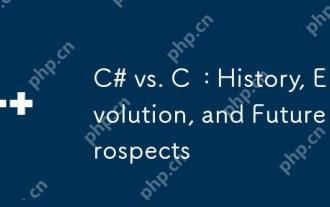
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
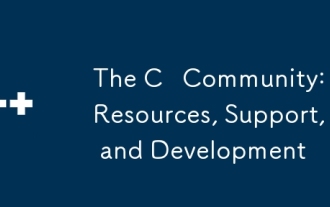
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
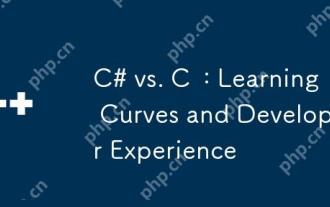
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
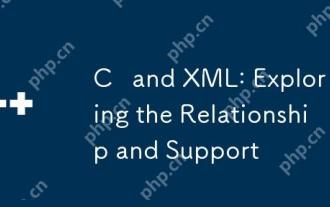
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
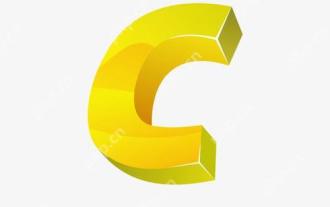
The application of static analysis in C mainly includes discovering memory management problems, checking code logic errors, and improving code security. 1) Static analysis can identify problems such as memory leaks, double releases, and uninitialized pointers. 2) It can detect unused variables, dead code and logical contradictions. 3) Static analysis tools such as Coverity can detect buffer overflow, integer overflow and unsafe API calls to improve code security.
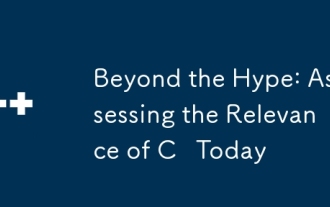
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
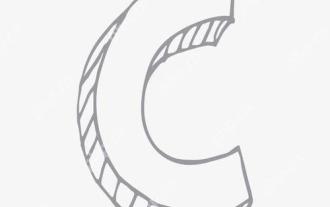
Using the chrono library in C can allow you to control time and time intervals more accurately. Let's explore the charm of this library. C's chrono library is part of the standard library, which provides a modern way to deal with time and time intervals. For programmers who have suffered from time.h and ctime, chrono is undoubtedly a boon. It not only improves the readability and maintainability of the code, but also provides higher accuracy and flexibility. Let's start with the basics. The chrono library mainly includes the following key components: std::chrono::system_clock: represents the system clock, used to obtain the current time. std::chron
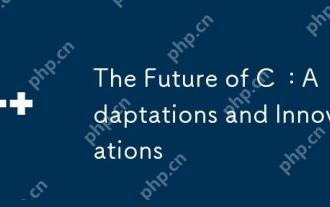
The future of C will focus on parallel computing, security, modularization and AI/machine learning: 1) Parallel computing will be enhanced through features such as coroutines; 2) Security will be improved through stricter type checking and memory management mechanisms; 3) Modulation will simplify code organization and compilation; 4) AI and machine learning will prompt C to adapt to new needs, such as numerical computing and GPU programming support.
