Beginners Guide to PHP Form Handling with Cookies
In this guide, we'll explore PHP form handling using cookies to store user data. Cookies are a way to persist small amounts of data on the user's browser, making it possible to remember user preferences or information across different sessions.
Our project involves creating a form where users can input their information, storing the submitted data in cookies, and then viewing or deleting the cookie data. By the end of this tutorial, you'll understand how to set, retrieve, and delete cookies in PHP.
What Are Cookies?
Cookies are small files stored on the user's browser. They allow web servers to store data specific to a user and retrieve it on subsequent visits. In PHP, you can work with cookies using the setcookie() function to create or update cookies and the $_COOKIE superglobal to read them.
The Project: PHP Form with Cookie Handling
We'll create a simple application that:
- Allows users to submit their information via a form.
- Stores the submitted data in cookies.
- Displays the stored cookie data.
- Provides an option to delete the cookies.
File Structure
Our project includes the following files:
project-folder/ │ ├── index.php # Form page ├── submit.php # Form handling and cookie storage ├── view_cookie.php # Viewing cookie data ├── delete_cookie.php # Deleting cookie data
Step 1: Creating the Form (index.php)
The index.php file contains the HTML form for user input, along with buttons to view or delete cookie data.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>PHP Form with Cookie Handling</title> </head> <body> <h1>Submit Your Information</h1> <!-- Form Section for User Input --> <form method="get" action="submit.php"> <label for="name">Name:</label><br> <input type="text"> <hr> <h3> Step 2: Handling Form Submission (submit.php) </h3> <p>The submit.php file processes the form data, validates and sanitizes it, and then stores it in cookies.<br> </p> <pre class="brush:php;toolbar:false"><?php // Initialize error messages and data variables $error_name = ""; $error_age = ""; $error_email = ""; $error_website = ""; $name = $age = $email = $website = $gender = $comments = $hobbies = ""; // Sanitize and validate the form data if ($_SERVER["REQUEST_METHOD"] == "GET") { // Sanitize inputs $name = htmlspecialchars(trim($_GET['name'])); $age = htmlspecialchars(trim($_GET['age'])); $email = htmlspecialchars(trim($_GET['email'])); $website = htmlspecialchars(trim($_GET['website'])); $gender = isset($_GET['gender']) ? $_GET['gender'] : ''; $hobbies = isset($_GET['hobbies']) ? $_GET['hobbies'] : []; $comments = htmlspecialchars(trim($_GET['comments'])); // Validation checks if (empty($name)) { $error_name = "Name is required."; } if (empty($age) || !filter_var($age, FILTER_VALIDATE_INT) || $age <= 0) { $error_age = "Valid age is required."; } if (empty($email) || !filter_var($email, FILTER_VALIDATE_EMAIL)) { $error_email = "Valid email is required."; } if (empty($website) || !filter_var($website, FILTER_VALIDATE_URL)) { $error_website = "Valid website URL is required."; } // If no errors, set cookies if (empty($error_name) && empty($error_age) && empty($error_email) && empty($error_website)) { // Set cookies for the form data setcookie("name", $name, time() + (86400 * 30), "/"); setcookie("age", $age, time() + (86400 * 30), "/"); setcookie("email", $email, time() + (86400 * 30), "/"); setcookie("website", $website, time() + (86400 * 30), "/"); setcookie("gender", $gender, time() + (86400 * 30), "/"); setcookie("hobbies", implode(", ", $hobbies), time() + (86400 * 30), "/"); setcookie("comments", $comments, time() + (86400 * 30), "/"); } } ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Form Submission Result</title> </head> <body> <h1>Form Submission Result</h1> <!-- Show Errors if any --> <?php if ($error_name) { echo "<p> <hr> <h3> Step 3: Viewing Cookie Data (view_cookie.php) </h3> <p>This file displays the cookie data stored on the user's browser.<br> </p> <pre class="brush:php;toolbar:false"><!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>View Cookie Data</title> </head> <body> <h1>View Stored Cookie Data</h1> <?php if (isset($_COOKIE['name'])) { echo "<p><strong>Name:</strong> " . $_COOKIE['name'] . "</p>"; echo "<p><strong>Age:</strong> " . $_COOKIE['age'] . "</p>"; echo "<p><strong>Email:</strong> " . $_COOKIE['email'] . "</p>"; echo "<p><strong>Website:</strong> <a href='" . $_COOKIE['website'] . "' target='_blank'>" . $_COOKIE['website'] . "</a></p>"; echo "<p><strong>Gender:</strong> " . $_COOKIE['gender'] . "</p>"; echo "<p><strong>Hobbies:</strong> " . $_COOKIE['hobbies'] . "</p>"; echo "<p><strong>Comments:</strong> " . $_COOKIE['comments'] . "</p>"; } else { echo "<p>No cookie data found!</p>"; } ?> <br><br> <a href="index.php">Go Back</a> </body> </html>
Step 4: Deleting Cookie Data (delete_cookie.php)
This file deletes the cookies by setting their expiration time to the past.
<?php // Deleting cookies by setting their expiration time to past setcookie("name", "", time() - 3600, "/"); setcookie("age", "", time() - 3600, "/"); setcookie("email", "", time() - 3600, "/"); setcookie("website", "", time() - 3600, "/"); setcookie("gender", "", time() - 3600, "/"); setcookie("hobbies", "", time() - 3600, "/"); setcookie("comments", "", time() - 3600, "/"); ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Cookie Deleted</title> </head> <body> <h1>Cookies Deleted</h1> <p>All cookies have been deleted successfully.</p> <br><br> <a href="index.php">Go Back</a> </body> </html>
Conclusion
This project demonstrates how to use cookies for form handling in PHP. By implementing cookies, you can persist user data and improve the functionality of your web applications. Experiment with this project and explore more advanced use cases for cookies in PHP.
Happy coding! ?
The above is the detailed content of Beginners Guide to PHP Form Handling with Cookies. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
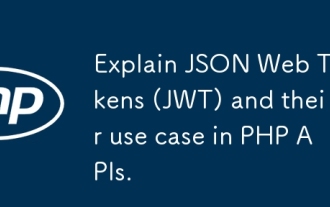
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,

The enumeration function in PHP8.1 enhances the clarity and type safety of the code by defining named constants. 1) Enumerations can be integers, strings or objects, improving code readability and type safety. 2) Enumeration is based on class and supports object-oriented features such as traversal and reflection. 3) Enumeration can be used for comparison and assignment to ensure type safety. 4) Enumeration supports adding methods to implement complex logic. 5) Strict type checking and error handling can avoid common errors. 6) Enumeration reduces magic value and improves maintainability, but pay attention to performance optimization.
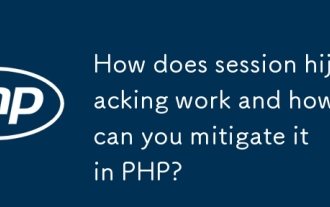
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
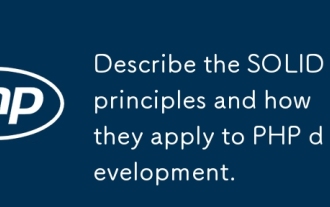
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
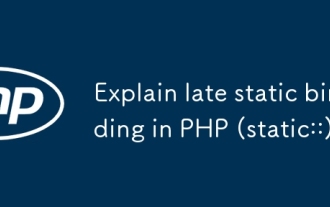
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
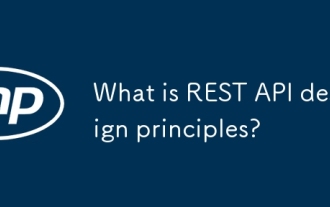
RESTAPI design principles include resource definition, URI design, HTTP method usage, status code usage, version control, and HATEOAS. 1. Resources should be represented by nouns and maintained at a hierarchy. 2. HTTP methods should conform to their semantics, such as GET is used to obtain resources. 3. The status code should be used correctly, such as 404 means that the resource does not exist. 4. Version control can be implemented through URI or header. 5. HATEOAS boots client operations through links in response.
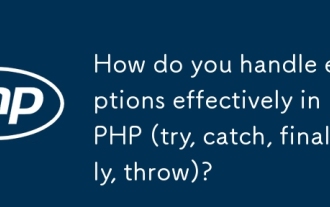
In PHP, exception handling is achieved through the try, catch, finally, and throw keywords. 1) The try block surrounds the code that may throw exceptions; 2) The catch block handles exceptions; 3) Finally block ensures that the code is always executed; 4) throw is used to manually throw exceptions. These mechanisms help improve the robustness and maintainability of your code.
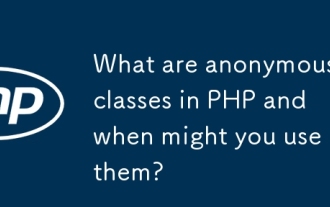
The main function of anonymous classes in PHP is to create one-time objects. 1. Anonymous classes allow classes without names to be directly defined in the code, which is suitable for temporary requirements. 2. They can inherit classes or implement interfaces to increase flexibility. 3. Pay attention to performance and code readability when using it, and avoid repeatedly defining the same anonymous classes.
