Understanding Vue Reactivity with Pinia Stores
At my workplace I was being tasked with creating a mock chat store for internal local dev work, and while doing so I made few notes about Vue (I had some experience, but not with hooks), So this is just my obsidian notes, I hope its useful to you :)
Table of Contents
- Ref and Reactive References
- Watch and Reactivity
- Pinia Store Integration
- Practical Examples
- Best Practices
- Common Gotchas
Ref and Reactive References
What is Ref?
ref is Vue's way of making primitive values reactive. It wraps the value in a reactive object with a .value property.
import { ref } from 'vue' // Inside Pinia Store export const useMyStore = defineStore('my-store', () => { // Creates a reactive reference const count = ref<number>(0) // To access or modify: function increment() { count.value++ // Need .value for refs } return { count, // When exposed, components can use it without .value increment } })
Types of Refs in Stores
// Simple ref const isLoading = ref<boolean>(false) // Array ref const messages = ref<Message[]>([]) // Complex object ref const currentUser = ref<User | null>(null) // Ref with undefined const selectedId = ref<string | undefined>(undefined)
Watch and Reactivity
Basic Watch Usage
import { watch, ref } from 'vue' export const useMyStore = defineStore('my-store', () => { const messages = ref<Message[]>([]) // Simple watch watch(messages, (newMessages, oldMessages) => { console.log('Messages changed:', newMessages) }) })
Watch Options
// Immediate execution watch(messages, (newMessages) => { // This runs immediately and on changes }, { immediate: true }) // Deep watching watch(messages, (newMessages) => { // Detects deep object changes }, { deep: true }) // Multiple sources watch( [messages, selectedId], ([newMessages, newId], [oldMessages, oldId]) => { // Triggers when either changes } )
Pinia Store Integration
Store Structure with Refs
export const useMyStore = defineStore('my-store', () => { // State const items = ref<Item[]>([]) const isLoading = ref(false) const error = ref<Error | null>(null) // Computed const itemCount = computed(() => items.value.length) // Actions const fetchItems = async () => { isLoading.value = true try { items.value = await api.getItems() } catch (e) { error.value = e as Error } finally { isLoading.value = false } } return { items, isLoading, error, itemCount, fetchItems } })
Composing Stores
export const useMainStore = defineStore('main-store', () => { // Using another store const otherStore = useOtherStore() // Watching other store's state watch( () => otherStore.someState, (newValue) => { // React to other store's changes } ) })
Practical Examples
Auto-refresh Implementation
export const useChatStore = defineStore('chat-store', () => { const messages = ref<Message[]>([]) const refreshInterval = ref<number | null>(null) const isRefreshing = ref(false) // Watch for auto-refresh state watch(isRefreshing, (shouldRefresh) => { if (shouldRefresh) { startAutoRefresh() } else { stopAutoRefresh() } }) const startAutoRefresh = () => { refreshInterval.value = window.setInterval(() => { fetchNewMessages() }, 5000) } const stopAutoRefresh = () => { if (refreshInterval.value) { clearInterval(refreshInterval.value) refreshInterval.value = null } } return { messages, isRefreshing, startAutoRefresh, stopAutoRefresh } })
Loading State Management
export const useDataStore = defineStore('data-store', () => { const data = ref<Data[]>([]) const isLoading = ref(false) const error = ref<Error | null>(null) // Watch loading state for side effects watch(isLoading, (loading) => { if (loading) { // Show loading indicator } else { // Hide loading indicator } }) // Watch for errors watch(error, (newError) => { if (newError) { // Handle error (show notification, etc.) } }) })
Best Practices
1. Ref Initialisation
// ❌ Bad const data = ref() // Type is 'any' // ✅ Good const data = ref<string[]>([]) // Explicitly typed
2. Watch Cleanup
// ❌ Bad - No cleanup watch(source, () => { const timer = setInterval(() => {}, 1000) }) // ✅ Good - With cleanup watch(source, () => { const timer = setInterval(() => {}, 1000) return () => clearInterval(timer) // Cleanup function })
3. Computed vs Watch
// ❌ Bad - Using watch for derived state watch(items, (newItems) => { itemCount.value = newItems.length }) // ✅ Good - Using computed for derived state const itemCount = computed(() => items.value.length)
4. Store Organization
// ✅ Good store organization export const useStore = defineStore('store', () => { // State refs const data = ref<Data[]>([]) const isLoading = ref(false) // Computed properties const isEmpty = computed(() => data.value.length === 0) // Watchers watch(data, () => { // Handle data changes }) // Actions const fetchData = async () => { // Implementation } // Return public interface return { data, isLoading, isEmpty, fetchData } })
Common Gotchas
- Forgetting .value
// ❌ Bad const count = ref(0) count++ // Won't work // ✅ Good count.value++
- Watch Timing
// ❌ Bad - Might miss initial state watch(source, () => {}) // ✅ Good - Catches initial state watch(source, () => {}, { immediate: true })
- Memory Leaks
// ❌ Bad - No cleanup const store = useStore() setInterval(() => { store.refresh() }, 1000) // ✅ Good - With cleanup const intervalId = setInterval(() => { store.refresh() }, 1000) onBeforeUnmount(() => clearInterval(intervalId))
Remember: Always consider cleanup, type safety, and proper organization when working with refs and watches in Pinia stores
The above is the detailed content of Understanding Vue Reactivity with Pinia Stores. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
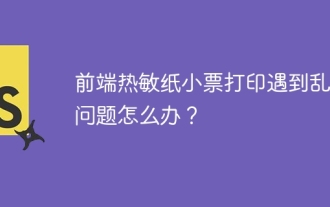
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
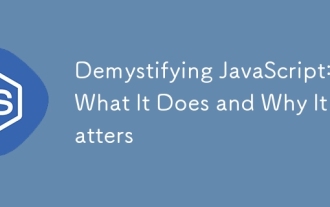
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
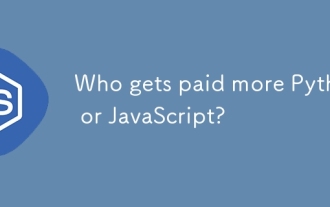
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
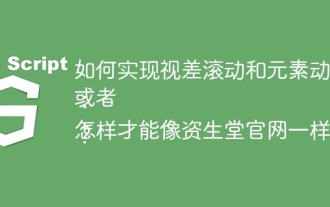
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
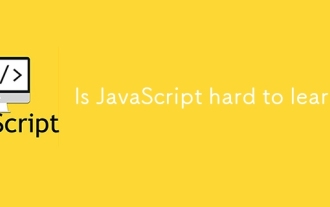
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
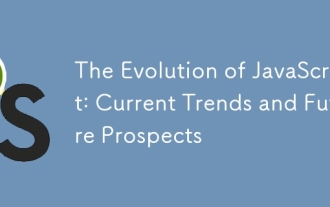
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
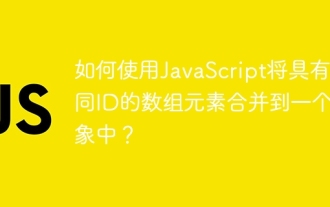
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
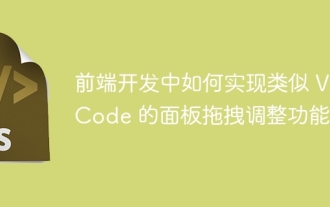
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
