How to Capture Web Page Screenshots with Next.js and Puppeteer
Capturing screenshots of web pages programmatically can be incredibly useful for generating previews, creating image-based reports, and more. In this guide, we’ll build a Next.js API route that takes a URL and generates a PNG screenshot. Our setup uses Puppeteer and chrome-aws-lambda to leverage a headless Chrome browser, making it versatile and production-ready.
We’ll start by setting up a new Next.js project and walking through the code step-by-step to understand how the API captures screenshots.
Prerequisites
- Setting up the Next.js app
- Configuring the API route with Puppeteer
- Creating the React component for the capture interface
- Explanation of local vs. deployment configurations for Puppeteer
Getting Started with a New Next.js Project
- Create a new Next.js app:
npx create-next-app@latest capture-image-app cd capture-image-app
- Install the necessary dependencies:
npm install puppeteer puppeteer-core chrome-aws-lambda busboy
Step 2: Create the API Route to Generate Screenshots
Now, we’ll set up an API endpoint to capture and return screenshots based on a provided URL.
In the pages/api folder, create a new file named generate-png.ts and add this code:
import { NextApiRequest, NextApiResponse } from "next"; import busboy, { Busboy } from "busboy"; // Use busboy for multipart parsing import chromium from "chrome-aws-lambda"; import puppeteerCore from "puppeteer-core"; // Import puppeteer-core directly import puppeteer from "puppeteer"; // Import puppeteer directly // Conditional import for Puppeteer based on the environment const puppeteerModule = process.env.NODE_ENV === "production" ? puppeteerCore : puppeteer; export const config = { api: { bodyParser: false, // Disable default body parsing to handle raw binary data (Blob) }, }; const delay = (ms: number): Promise<void> => new Promise((resolve) => setTimeout(resolve, ms)); export default async function handler( req: NextApiRequest, res: NextApiResponse ): Promise<void> { try { if (req.method === "POST") { const bb: Busboy = busboy({ headers: req.headers }); let width: number = 1920; // Default width let height: number = 0; // Default height let delayTime: number = 6000; const buffers: Buffer[] = []; bb.on("file", (_name: string, file: NodeJS.ReadableStream) => { file.on("data", (data: Buffer) => buffers.push(data)); }); bb.on("field", (name: string, value: string) => { if (name === "width") width = parseInt(value, 10) || 1920; if (name === "height") height = parseInt(value, 10) || 0; if (name === "delay") delayTime = parseInt(value, 10) || 6000; }); bb.on("finish", async () => { const blobBuffer: Buffer = Buffer.concat(buffers); const htmlContent: string = blobBuffer.toString("utf-8"); const browser = await puppeteerModule.launch({ args: ["--start-maximized"], executablePath: process.env.NODE_ENV === "production" ? await chromium.executablePath || "/usr/bin/chromium-browser" : undefined, // No custom executable path needed for local headless: true, }); const page = await browser.newPage(); // Load the HTML content directly await page.setContent(htmlContent, { waitUntil: "networkidle0" }); //@ts-expect-error todo const bodyHeight = await page.evaluate(() => { return document.body.scrollHeight; // Get the full scrollable height of the body }); await page.setViewport({ width: Number(width), height: height || bodyHeight, // Use the provided height or fallback to the full body height deviceScaleFactor: 2, }); await delay(delayTime); const screenshotBuffer = await page.screenshot({ fullPage: !height, type: "png", omitBackground: false, }); await browser.close(); res.setHeader("Content-Type", "image/png"); res.setHeader( "Content-Disposition", "attachment; filename=screenshot.png" ); res.status(200).end(screenshotBuffer); }); req.pipe(bb); // Pipe the request stream to busboy } else { res.setHeader("Allow", ["POST"]); res.status(405).end(`Method ${req.method} Not Allowed`); } } catch (error) { console.error("ERROR", error); res.status(500).end("Internal Server Error"); } }
*Explanation: Choosing Puppeteer for Local vs. Production Environments
*
In this code, we’ve set up a dynamic import for puppeteer:
Local Development: If NODE_ENV is not production, it uses puppeteer, which is simpler to set up and doesn’t require chrome-aws-lambda.
Production: For serverless deployments, the environment will detect NODE_ENV as production and load puppeteer-core along with chrome-aws-lambda, which allows it to work in AWS Lambda and other similar environments. In this setup, chrome-aws-lambda provides the correct Chromium path, ensuring compatibility with serverless providers.
Step 3: Create a Simple React Component for the UI
Here, we’ll create a straightforward form that lets users input values for the webpage capture. This form will trigger the generate function to capture and download the screenshot in PDF format.
import { useState } from "react"; export default function ScreenCaptureComponent() { const [isProcessing, setProcessing] = useState(false); const [width, setWidth] = useState<string>("1920"); const [height, setHeight] = useState<string>("1000"); const [delay, setDelay] = useState<string>("6000"); // Function to clone HTML and prepare for capture function takeScreenshot() { const clonedElement = document.body.cloneNode(true) as HTMLElement; const blob = new Blob([clonedElement.outerHTML], { type: "text/html" }); return blob; } // Function to capture screenshot by sending cloned HTML to API async function generateCapture() { setProcessing(true); const htmlBlob = takeScreenshot(); if (!htmlBlob) { setProcessing(false); return; } try { const formData = new FormData(); formData.append("file", htmlBlob); formData.append("width", width); formData.append("height", height); formData.append("delay", delay); const response = await fetch("/api/generate-png", { method: "POST", body: formData, }); if (!response.ok) throw new Error("Capture failed"); const blob = await response.blob(); const downloadUrl = URL.createObjectURL(blob); const link = document.createElement("a"); link.href = downloadUrl; link.download = "capture.png"; link.click(); URL.revokeObjectURL(downloadUrl); } catch (error) { console.error("Failed to capture screenshot", error); } finally { setProcessing(false); } } return ( <div style={{ maxWidth: "400px", margin: "50px auto", padding: "24px", backgroundColor: "white", borderRadius: "8px", width: "100%", boxShadow: "0 4px 6px rgba(0, 0, 0, 0.1)", }} > <h2 style={{ fontSize: "24px", fontWeight: "600", textAlign: "center", marginBottom: "16px", }} > Webpage Screenshot Capture </h2> <form onSubmit={(e) => { e.preventDefault(); generateCapture(); }} style={{ display: "flex", flexDirection: "column", alignItems: "center", marginBottom: "16px", }} > <label style={{ marginBottom: "8px", fontWeight: "500" }} htmlFor="width" > Width (px) </label> <select id="width" value={width} onChange={(e) => setWidth(e.target.value)} style={{ width: "100%", padding: "8px", marginBottom: "16px", borderRadius: "4px", border: "1px solid #ccc", outline: "none", }} > <option value="1920">1920 (Full HD)</option> <option value="1366">1366 (Laptop)</option> <option value="1280">1280 (Desktop)</option> <option value="1024">1024 (Tablet Landscape)</option> <option value="768">768 (Tablet Portrait)</option> <option value="375">375 (Mobile)</option> </select> <label style={{ marginBottom: "8px", fontWeight: "500" }} htmlFor="height" > Height (px) </label> <input type="number" id="height" value={height} onChange={(e) => setHeight(e.target.value)} required style={{ width: "100%", padding: "8px", marginBottom: "16px", borderRadius: "4px", border: "1px solid #ccc", outline: "none", }} /> <label style={{ marginBottom: "8px", fontWeight: "500" }} htmlFor="delay" > Delay (ms) </label> <input type="number" id="delay" value={delay} onChange={(e) => setDelay(e.target.value)} required style={{ width: "100%", padding: "8px", marginBottom: "16px", borderRadius: "4px", border: "1px solid #ccc", outline: "none", }} /> <button type="submit" disabled={isProcessing} style={{ padding: "8px 16px", color: "white", borderRadius: "4px", transition: "background-color 0.3s", backgroundColor: isProcessing ? "#b0bec5" : "#2196F3", cursor: isProcessing ? "not-allowed" : "pointer", }} > {isProcessing ? "Capturing..." : "Capture Screenshot"} </button> </form> {/* Example HTML Element to Capture */} <div id="capture-area" style={{ display: "none" }}> <h3 style={{ fontSize: "20px", fontWeight: "600", }} > Content to Capture </h3> <p>This is an example of the HTML content that will be captured.</p> </div> </div> ); }
Conclusion
This tutorial covers setting up a webpage capture tool in Next.js, handling screenshots with Puppeteer, and creating an interactive frontend component. Remember to use puppeteer locally and switch to puppeteer-core in production to reduce bundle size and optimize for serverless environments. Happy coding!
The above is the detailed content of How to Capture Web Page Screenshots with Next.js and Puppeteer. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










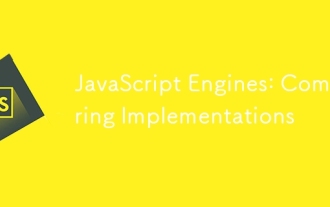
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
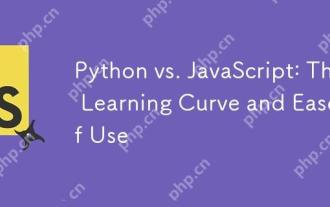
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
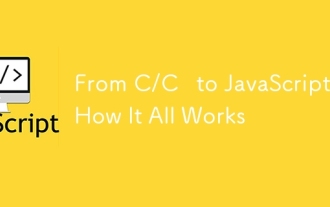
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
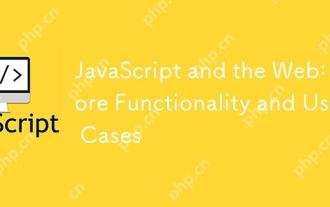
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
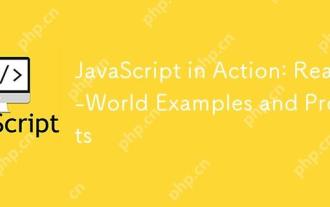
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
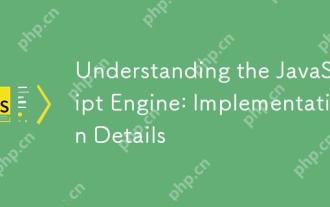
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.
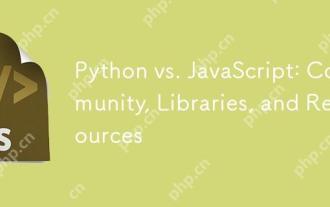
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
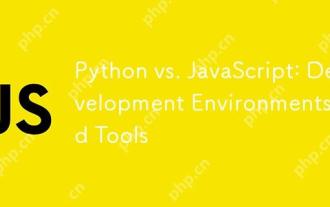
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
