


Can You Dynamically Find All Structs Implementing an Interface in Go?
Scanning for Structs Implementing an Interface in Go
Background:
In Go, we may encounter scenarios where we need to dynamically access multiple structs that implement a common interface. However, since Go is a statically typed language, it doesn't provide a built-in mechanism to scan for all structs of a specific interface type.
Question:
Given an interface with start() and stop() methods, is it possible to retrieve a list of all structs that implement that interface? This information would enable us to invoke start() and stop() on all instances of the implemented structs dynamically.
Answer:
Unfortunately, no, this is not possible directly. Go's static typing eliminates unused type definitions, making it difficult to access structs that have not been explicitly used in the application.
Alternative Approach:
Instead of dynamically scanning for structs, an alternative solution is to create a global map or slice. Each struct implementing the interface can then add an instance to this map during application initialization using an init() function. This ensures that all instances of the various structs are accessible and can be managed centrally.
Example:
<code class="go">var instMap = map[string]StartStopper type A struct {} func init() { instMap["A"] = new(A) }</code>
By iterating over this map, we can dynamically invoke the start() method on all registered instances.
Considerations for Multiple Instances:
If multiple instances of each type can exist, you'll need to add instances to the map manually whenever they're created. Additionally, you should remove instances from the map when they're no longer needed to prevent the Garbage Collector from ignoring them.
The above is the detailed content of Can You Dynamically Find All Structs Implementing an Interface in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
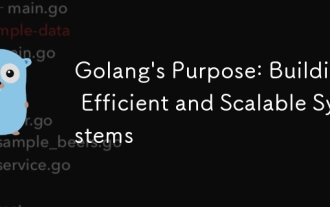
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
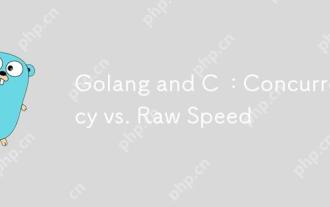
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
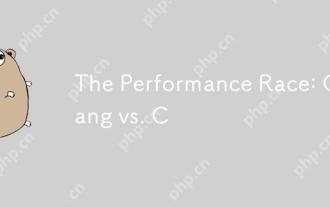
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
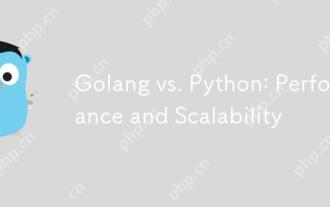
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
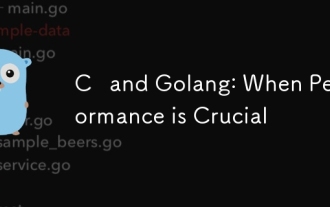
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
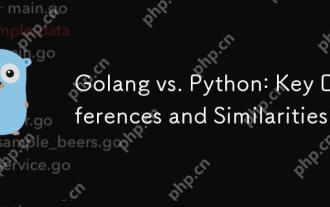
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
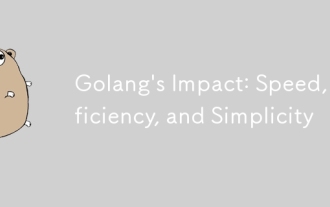
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
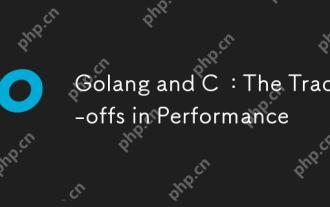
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
