


JavaScript Variables and Data Types: Storing and manipulating data in JavaScript.
JavaScript is a versatile programming language that powers the web, enabling developers to create interactive and dynamic websites. One of the core concepts in JavaScript, and in any programming language, is how data is stored and manipulated. To effectively build web applications, it's essential to understand variables and data types in JavaScript.
In this article, we'll cover what variables are, how to declare them, and the various data types that JavaScript supports for storing and manipulating data.
Variables in JavaScript
What is a Variable?
A variable in JavaScript is like a container that holds data. It allows you to store and retrieve values that you can use throughout your program. Think of variables as labels attached to values. Once you assign a value to a variable, you can refer to it by its name, rather than using the value directly every time.
For example, instead of writing "John" multiple times, you can assign it to a variable like this:
let name = "John"; console.log(name); // Outputs: John
Declaring Variables
In JavaScript, variables can be declared using the var, let, or const keywords.
1. var
var is the oldest way of declaring variables in JavaScript. However, it has some issues with scope, which is why modern JavaScript developers prefer using let and const.
var age = 30; console.log(age); // Outputs: 30
2. let
let is block-scoped, meaning the variable exists only within the block it is defined in (e.g., inside a function or a loop). It is the most commonly used way to declare variables in modern JavaScript.
let city = "New York"; console.log(city); // Outputs: New York
3. const
const is similar to let, but it is used to declare variables whose values will not change. Once a value is assigned to a variable declared with const, it cannot be re-assigned.
const country = "USA"; console.log(country); // Outputs: USA // This will throw an error // country = "Canada";
Naming Variables
When naming variables, keep the following rules in mind:
- Variable names can contain letters, numbers, underscores (_), and dollar signs ($).
- They must begin with a letter, underscore, or dollar sign.
- Variable names are case-sensitive (e.g., myVar and myvar are different variables).
- JavaScript keywords (e.g., var, let, if, function) cannot be used as variable names.
A common convention is to use camelCase for variable names, like myVariableName.
Data Types in JavaScript
JavaScript supports various data types that specify the kind of value a variable can hold. Data types are divided into two categories:
- Primitive Data Types
- Non-Primitive (Reference) Data Types
Primitive Data Types
Primitive data types are the most basic types of data in JavaScript. They include:
1. String
Strings are used to represent textual data. They are enclosed in quotes—either single ('), double (") or backticks (`).
let greeting = "Hello, World!"; let anotherGreeting = 'Hi there!'; console.log(greeting); // Outputs: Hello, World!
2. Number
The number data type represents both integers and floating-point numbers (i.e., decimals).
let age = 25; // Integer let price = 99.99; // Floating-point number
3. Boolean
Booleans represent logical values—true or false. They are often used in conditional statements and comparisons.
let isLoggedIn = true; let hasAccess = false;
4. Undefined
When a variable is declared but not assigned a value, it is automatically initialized with the value undefined.
let myVar; console.log(myVar); // Outputs: undefined
5. Null
null represents an explicitly empty or non-existent value. It is used when you want to indicate that a variable should have no value.
let emptyValue = null;
6. Symbol
Symbols are unique and immutable values, typically used to create unique property keys for objects. While not commonly used by beginners, they are useful in advanced applications.
let symbol1 = Symbol("description");
7. BigInt
The BigInt type allows for the representation of whole numbers larger than the range of the Number type. It’s especially useful when working with very large integers.
let bigNumber = BigInt(123456789012345678901234567890);
Non-Primitive (Reference) Data Types
Non-primitive data types store more complex data structures and objects. They are known as reference types because variables store references to the actual data.
1. Object
Objects are collections of key-value pairs. They allow you to store multiple related values as properties.
let person = { name: "John", age: 30, isStudent: false }; console.log(person.name); // Outputs: John
2. Array
Arrays are ordered collections of values (elements). Arrays can store multiple values in a single variable, and the values can be of any data type.
let fruits = ["Apple", "Banana", "Cherry"]; console.log(fruits[1]); // Outputs: Banana
3. Function
Functions are blocks of code designed to perform a particular task. In JavaScript, functions themselves are treated as objects, allowing them to be passed as arguments or stored in variables.
function greet() { console.log("Hello!"); } greet(); // Outputs: Hello!
Type Coercion and Dynamic Typing
JavaScript is dynamically typed, which means you don’t need to explicitly declare the type of a variable. JavaScript will automatically infer the type based on the value assigned. For example:
let variable = "Hello"; // variable is of type string variable = 42; // variable is now of type number
In addition, JavaScript performs type coercion, which means it will automatically convert values from one type to another when necessary.
console.log("5" + 10); // Outputs: "510" (String concatenation) console.log("5" - 1); // Outputs: 4 (Number subtraction)
In the first example, JavaScript coerces 10 to a string and concatenates it with "5". In the second example, "5" is coerced into a number for subtraction.
Conclusion
Understanding variables and data types is a fundamental step in learning JavaScript. Variables allow you to store and manage data in your programs, while data types define the kind of data you’re working with, from strings to numbers, booleans, and beyond.
As you continue learning JavaScript, you'll frequently use variables and work with various data types to build interactive and dynamic web applications. By mastering how to manipulate these data types, you’ll be able to write more efficient and effective code.
The above is the detailed content of JavaScript Variables and Data Types: Storing and manipulating data in JavaScript.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










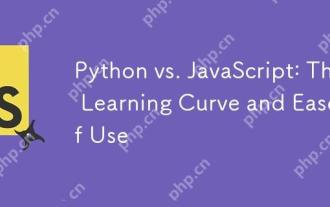
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
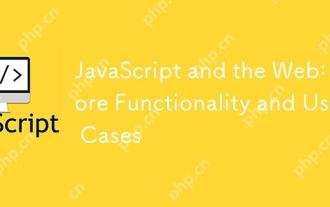
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
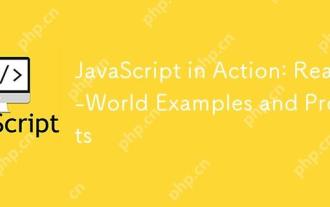
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
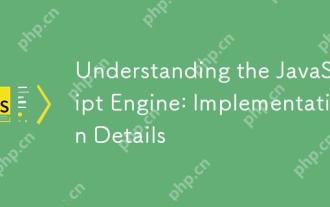
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.
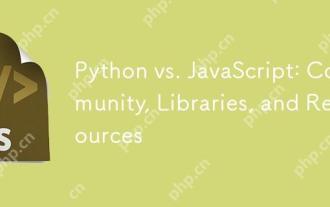
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
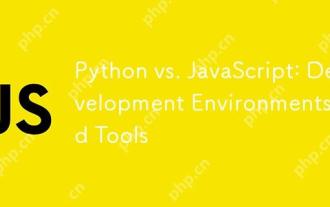
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
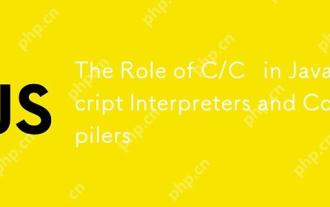
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.

Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
